C++에서 Double을 Int로 반올림하는 방법
-
Double to Int 반올림에
round()
함수 사용 -
lround()
함수를 사용하여 Double을 가장 가까운 정수로 변환 -
Double에서 Int로 반올림하려면
trunc()
함수 사용
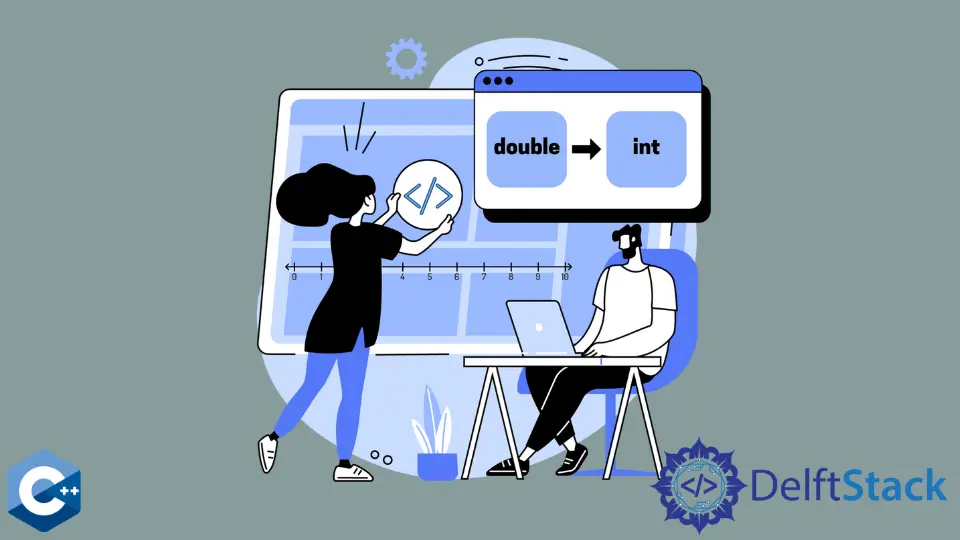
이 기사에서는 C++에서 double을 정수로 반올림하는 방법에 대한 몇 가지 방법을 설명합니다.
Double to Int 반올림에round()
함수 사용
round()
함수는<cmath>
헤더에 정의되어 있습니다. 사용자가 전달하는 인수의 가장 가까운 정수 값을 계산할 수 있습니다. 중간 케이스는 0에서 반올림되며 반환 된 값은 인수와 동일한 유형입니다. 다음 예제에서는 임의의 double 벡터를 초기화하고 각 요소에 대해round()
함수를 출력합니다.
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << round(item) << endl;
}
return EXIT_SUCCESS;
}
출력:
round(-3.5) = -4
round(-21.1) = -21
round(-1.99) = -2
round(0.129) = 0
round(2.5) = 3
round(3.111) = 3
lround()
함수를 사용하여 Double을 가장 가까운 정수로 변환
또는round()
와 거의 동일하게 작동하는lround()
함수를 사용할 수 있습니다.이 함수는 한 가지 차이점을 제외하고는long
정수 형식으로 값을 반환합니다. 두 함수 모두에서 반환 값이 예상치 못한데, 이는 어디에나있는 다른 반올림 오류로 인해 발생할 수 있습니다. 해당 시나리오는 여기 문서에 지정된대로 처리해야합니다.
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << lround(item) << endl;
}
return EXIT_SUCCESS;
}
Double에서 Int로 반올림하려면trunc()
함수 사용
trunc()
는<cmath>
헤더의 또 다른 유용한 함수로, 인수로 전달 된 숫자보다 크지 않은 가장 가까운 정수를 계산합니다. trunc()
는 부동 소수점 형식의 반환 값을round()
로 계산합니다. 이 두 함수는 현재 반올림 모드에서 효과가 없습니다. 오류 처리 사례는 매뉴얼 페이지에 설명되어 있습니다.
#include <cmath>
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> dfloats{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
for (auto &item : dfloats) {
cout << "round(" << item << ") = " << trunc(item) << endl;
}
return EXIT_SUCCESS;
}
round(-3.5) = -3
round(-21.1) = -21
round(-1.99) = -1
round(0.129) = 0
round(2.5) = 2
round(3.111) = 3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook