C++에서 랜덤 더블을 생성하는 방법
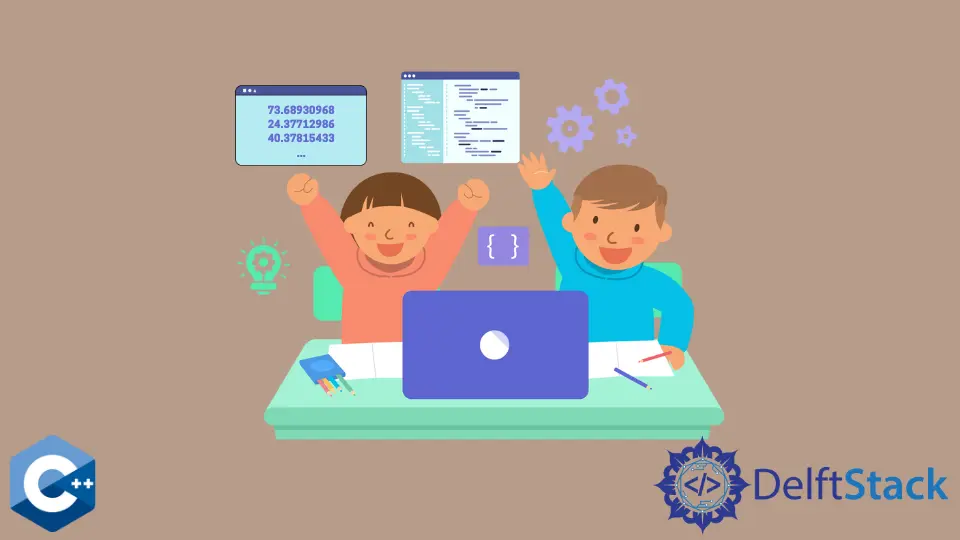
이 기사에서는 C++에서 임의의 double
부동 소수점 숫자를 생성하는 방법에 대한 몇 가지 방법을 설명합니다.
C++ 11 <random>
라이브러리를 사용하여 C++에서 랜덤 더블 생성
C++ 11 버전부터 표준 라이브러리는 난수/의사 난수 생성을위한 클래스와 메서드를 제공합니다. 고품질 난수가 필요한 모든 응용 프로그램은 이러한 방법을 사용해야하지만 다른 경우에도이 깔끔하고 기능이 풍부한 STL 인터페이스의 이점을 누릴 수 있습니다.
처음에 초기화되는std::random_device
객체는 다음 줄에서 초기화 된 난수 엔진std::default_random_engine
을 시드하는 데 사용되는 비 결정적 균일 랜덤 비트 생성기입니다. 이 단계는 엔진이 동일한 시퀀스를 생성하지 않도록합니다. 여러 난수 엔진이 C++ 숫자 라이브러리에 구현되어 있으며 시간/공간 요구 사항이 다릅니다 (전체 목록은 여기 참조).
다음 예제에서는std::default_random_engine
을 사용하여 의사 난수 값을 생성하지만 애플리케이션 제약 조건에 따라 특정 알고리즘 엔진을 초기화 할 수 있습니다. 다음으로 균일 분포를 초기화하고 최소/최대 값을 선택적 인수로 전달합니다. 마지막으로[10-100]
간격에서 임의의 double 값 5 개를 콘솔에 출력합니다.
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
// Modify as needed
constexpr int MIN = 10;
constexpr int MAX = 100;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_real_distribution<double> distr(MIN, MAX);
for (int n = 0; n < 5; ++n) {
cout << setprecision(10) << distr(eng) << "\n";
}
cout << endl;
return EXIT_SUCCESS;
}
출력:
73.68930968
24.37712986
40.37815433
77.24899374
94.62192505
std::uniform_real_distribution <T>
는float
,double
또는long double
유형 중 하나가 템플릿 매개 변수로 전달되지 않으면 정의되지 않은 동작을 생성합니다. 다음 예제는 단 정밀도 부동 소수점 숫자를 생성합니다.
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
// Modify as needed
constexpr int MIN = 10;
constexpr int MAX = 100;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_real_distribution<float> distr(MIN, MAX);
for (int n = 0; n < 5; ++n) {
cout << setprecision(10) << distr(eng) << "\n";
}
cout << endl;
return EXIT_SUCCESS;
}
std::rand
함수를 사용하여 C++에서 랜덤 더블 생성
rand
함수는 C 표준 라이브러리 난수 생성 기능의 일부입니다. 고품질 임의성이 필요한 애플리케이션에는 권장되지 않지만 여러 시나리오에 사용할 수 있습니다. 행렬이나 벡터를 난수로 채우려는 경우.
이 함수는 0과 RAND_MAX
(둘 다 포함) 사이의 의사 난수 정수를 생성합니다. RAND_MAX
값은 구현에 따라 다르며 보장 된 최소값은 32767에 불과하므로 생성 된 숫자는 임의성이 제한됩니다. 이 함수는std::srand
로 시드해야합니다 (가급적std::time
을 사용하여 현재 시간을 전달). 마지막으로, 복잡한 산술로 배정 밀도 부동 소수점 값을 생성 할 수 있습니다.
#include <iomanip>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
using std::setprecision;
// Modify as needed
constexpr int MIN = 10;
constexpr int MAX = 100;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < 5; i++)
cout << setprecision(10)
<< MIN + (double)(rand()) / ((double)(RAND_MAX / (MAX - MIN))) << endl;
return EXIT_SUCCESS;
}
출력:
84.70076228
11.08804226
20.78055909
74.35545741
18.64741151
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook