How to Check if Key Exists in a Map in C++
-
Use the
std::map::find
Function to Check if Key Exists in a C++ Map -
Use the
std::map::count
Function to Check if Key Exists in a C++ Map -
Use the
std::map::contains
Function to Check if Key Exists in a C++ Map -
Use the
std::map::at
Function With Exception Handling to Check if Key Exists in a C++ Map -
Use a
for
Loop to Check if Key Exists in a C++ Map - Conclusion
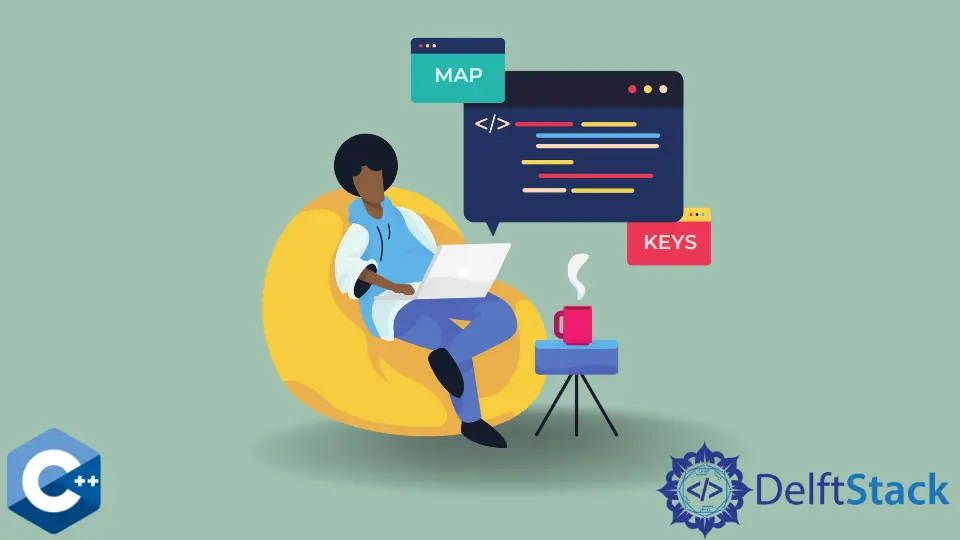
In C++, maps are a fundamental data structure that stores elements in a key-value pair fashion. Often, it becomes crucial to determine whether a specific key exists within a map before attempting any operations involving it. This task is essential for avoiding potential errors or unexpected behavior in a program.
This article will introduce methods on how to check if a key exists in a map in C++. Each method offers its approach, catering to different scenarios and preferences.
Use the std::map::find
Function to Check if Key Exists in a C++ Map
The std::map
is a part of the Standard Template Library (STL) in C++. It is a container that stores elements in key-value pairs, where each key is unique. The elements are sorted by their keys, which allows for efficient lookup operations based on the key.
On the other hand, STL also provides an unsorted version of the same container named std::unordered_map
. Both of these containers support the key searching methods that are described in this article.
To use std::map
, you need to include the <map>
header file:
#include <map>
You can declare a map using the following syntax:
std::map<KeyType, ValueType> myMap;
Here, KeyType
is the type of the keys, and ValueType
is the type of the values.
The find()
function is a member function of the std::map
class that allows you to search for a specific key within the map. It returns an iterator pointing to the element if the key is found or myMap.end()
if the key is not present.
Syntax:
iterator find(const KeyType& key);
const_iterator find(const KeyType& key) const;
key
: The the key you want to search for.iterator
: The type of the iterator for a non-const
map.const_iterator
: The type of the iterator for aconst
map.
In the following example, we initialize the map
of std::pair<string, string>
types and then take the key value from the user input passed to the find()
function. The example program outputs the affirmative string to the cout
stream.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
for (const auto& [key, value] : lang_map) {
cout << key << " : " << value << endl;
}
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.find(key_to_find) != lang_map.end()) {
cout << "Key Exists!" << endl;
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
This code first includes necessary header files like <iostream>
and <map>
. Then, it sets up aliases for std::cout
, std::cin
, std::endl
, std::map
, and std::string
for convenience.
Inside the main
function, a string variable key_to_find
is declared to hold the user’s input for the key they want to search. Next, a std::map
called lang_map
is created and initialized with key-value pairs representing programming languages and their respective keys.
The code then enters a loop that iterates through each element in lang_map
using a range-based for
loop. In each iteration, it prints out the key-value pairs using std::cout
.
After displaying the available keys and their corresponding languages, the program prompts the user to enter a key they want to search for. This input is stored in the key_to_find
variable.
Next, it uses lang_map.find(key_to_find)
to search for the specified key in the map. If the find
function does not return lang_map.end()
(indicating that the key was found), it prints "Key Exists!"
. Otherwise, it prints "Key does not exist!"
.
Output:
j : Julia
l : Lua
m : MATLAB
o : Octave
p : Python
s : Scala
Enter the key to search for: l
Key Exists!
Use the std::map::count
Function to Check if Key Exists in a C++ Map
Alternatively, one can utilize the count
built-in function of the std::map
container to check if a given key exists in a map object. Note that the count
function retrieves the number of elements that have the given key value.
Syntax:
size_type count(const KeyType& key) const;
key
: The key that you want to count in the map.size_type
: This is an unsigned integral type representing the size or count of elements in the map. It is typicallystd::size_t
.
Since map keys are unique, the return value will either be 1
(if the key is found) or 0
(if not found). Thus, we can use the count
function call as an if
condition to output the affirming string when the given key exists in a map object.
The following code prompts the user for a key, checks if it exists in the map using the count()
function, and then provides feedback based on whether or not the key is found.
#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.count(key_to_find)) {
cout << "Key Exists!" << endl;
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
This code begins by including necessary header files like <iostream>
for input/output operations and <map>
for using the std::map
container. Then, it sets up aliases for std::cout
, std::cin
, std::endl
, std::map
, and std::string
to simplify the code.
In the main
function, a string variable key_to_find
is declared to store the user’s input. Next, a std::map
named lang_map
is created and initialized with key-value pairs representing programming languages and their respective keys.
The program then prompts the user with the message "Enter the key to search for: "
. The user’s input is stored in the variable key_to_find
.
Afterwards, it uses lang_map.count(key_to_find)
to check if the entered key exists in the map. If the count is greater than 0
(indicating that the key was found), it prints "Key Exists!"
. Otherwise, it prints "Key does not exist!"
.
Output:
Enter the key to search for: l
Key Exists!
Use the std::map::contains
Function to Check if Key Exists in a C++ Map
The contains()
method was introduced in C++20 and is another built-in function that can be used to find if the key exists in a map. This function returns a Boolean value if the element with the given key exists in the object.
Syntax:
bool contains(const KeyType& key) const;
key
: The key that you want to check for in the map.
This function returns true
if the map contains an element with the specified key and false
otherwise.
The following code prompts the user for a key, checks if it exists in the map using the contains()
method, and then provides feedback based on whether or not the key is found.
contains()
method is available.#include <iostream>
#include <map>
using std::cin;
using std::cout;
using std::endl;
using std::map;
using std::string;
int main() {
string key_to_find;
std::map<string, string> lang_map = {{
"j",
"Julia",
},
{
"p",
"Python",
},
{
"m",
"MATLAB",
},
{
"o",
"Octave",
},
{
"s",
"Scala",
},
{
"l",
"Lua",
}};
cout << "Enter the key to search for: ";
cin >> key_to_find;
if (lang_map.contains(key_to_find)) {
cout << "Key Exists!" << endl;
} else {
cout << "Key does not exist!" << endl;
}
return EXIT_SUCCESS;
}
After including necessary libraries and setting up aliases for convenience, the main
function begins. A string
variable named key_to_find
is declared to store the user’s input for the key they wish to search.
Next, a std::map
called lang_map
is created and initialized with key-value pairs representing programming languages and their respective keys. This map associates single-letter keys ("j"
for Julia
, "p"
for Python
, and so on) with the corresponding language names.
The program then prompts the user with the message "Enter the key to search for: "
, and awaits their input, which is stored in the key_to_find
variable.
Subsequently, it employs the lang_map.contains(key_to_find)
statement to check if the specified key exists in the map. If the condition evaluates to true
, it prints "Key Exists!"
, otherwise it prints "Key does not exist!"
.
Output:
Enter the key to search for: l
Key Exists!
Use the std::map::at
Function With Exception Handling to Check if Key Exists in a C++ Map
The at()
function is used to access the element at a specified position in the map. By using this function in combination with exception handling, we can determine if a key exists in the map.
Syntax:
mapped_type& at(const KeyType& key);
const mapped_type& at(const KeyType& key) const;
key
: The key whose associated value you want to access.mapped_type
: The type of the mapped values in the map.KeyType
: The type of the keys in the map.
This function returns a reference to the value associated with the specified key. If the key does not exist in the map, it throws a std::out_of_range
exception.
The following code demonstrates how to use exception handling to check if a key exists in a map. If the key is found, it prints the associated value; otherwise, it catches the exception and prints a message indicating that the key was not found. This approach ensures robustness in handling potential key lookup errors.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
// Check if the key exists
try {
std::string value = myMap.at(2);
std::cout << "Key found! Value is: " << value << std::endl;
} catch (const std::out_of_range& oor) {
std::cout << "Key not found." << std::endl;
}
return 0;
}
In this code, a std::map
named myMap
is declared and initialized with three key-value pairs. The code proceeds to perform a key lookup operation using the try-catch
mechanism.
Within the try
block, it attempts to access the value associated with the key 2
using the statement myMap.at(2)
. If the key is present in the map, the associated value ("Two"
) is assigned to the variable value
, and it prints "Key found! Value is: Two"
to the console.
However, if the key is not found in the map, a std::out_of_range
exception is thrown. This is caught in the catch
block, and it executes the code within. If this is the case, it prints "Key not found"
to the console.
Output:
Key found! Value is: Two
Use a for
Loop to Check if Key Exists in a C++ Map
One approach to check if a key exists in a map is by using a for
loop to iterate through the map’s elements and comparing each key with the target key. You can use a for
loop along with an if
statement to check if a key exists in a map in C++.
In this example, the for
loop iterates through the map and checks if the specified key exists. If found, key_exists
is set to true
, and the loop is exited. Finally, it prints a message indicating whether the key exists or not.
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "One"}, {2, "Two"}, {3, "Three"}};
int key_to_find = 2;
bool key_exists = false;
// Check if the key exists using a for loop
for (const auto& pair : myMap) {
if (pair.first == key_to_find) {
key_exists = true;
break;
}
}
if (key_exists) {
std::cout << "Key exists!" << std::endl;
} else {
std::cout << "Key does not exist." << std::endl;
}
return 0;
}
This code demonstrates how to check if a specific key exists in a std::map
without using the find()
or at()
functions. It begins by including the necessary header files, <iostream>
and <map>
. In the main
function, a std::map
called myMap
is created, associating integer keys with corresponding string values.
The code then sets key_to_find
to 2
and initializes a Boolean variable key_exists
as false
. Next, it employs a for
loop to iterate through each pair in myMap
.
For each pair, it checks if the first element (the key) matches the value of key_to_find
. If a match is found, key_exists
is set to true
, and the loop is exited using the break
statement.
Following the loop, it evaluates key_exists
. If it is true
, it prints "Key exists!"
, otherwise, it prints "Key does not exist."
.
Output:
Key exists!
Using a for
loop to check for key existence in a C++ map provides a straightforward and effective approach. However, it’s worth noting that this method may not be as efficient as using the find()
function for large maps.
Conclusion
In summary, this article explored various methods to check if a key exists in a C++ map.
The std::map::find
function provides a direct way to search for a key, returning an iterator to the element if found. The std::map::count
function efficiently retrieves the number of elements with a given key, yielding either 1
(if found) or 0
(if not).
Introduced in C++20, the std::map::contains
method offers a concise way to perform key existence checks. Exception handling with std::map::at
provides a robust approach to determine if a key exists.
Additionally, a for
loop with an if
statement allows for manual key checking by iterating through the map.
These methods cater to different scenarios, empowering developers to effectively handle key existence checks in maps.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook