How to Raise Number to Power in C++
-
Use the
std::pow
Function to Raise a Number to the Power in C++ - Use the Custom Function to Raise a Vector of Numbers to the Power of 2 in C++
- Use the Custom Function to Raise a Vector of Numbers to the Power in C++
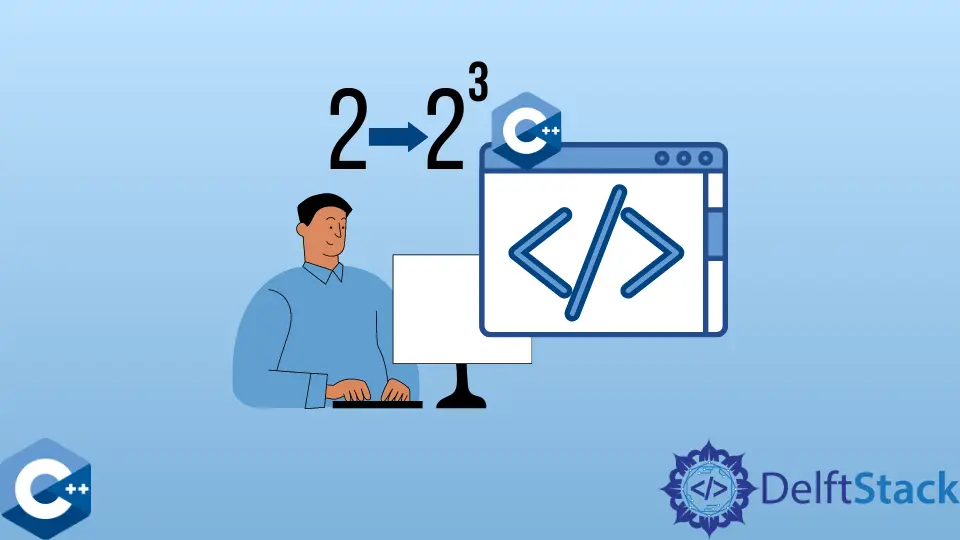
This article will demonstrate multiple methods of how to raise a number to the power in C++.
Use the std::pow
Function to Raise a Number to the Power in C++
The std::pow
function can be used to compute the product of a given base number raised to the power of n
, where n
can be an integral or floating-point value. Note that this function has multiple exceptions and special cases that need to be handled by the programmer or implemented using the separate function provided in the C++ <cmath>
library header. E.g. pow
can’t be used to calculate the root of a negative number, and instead, we should utilize std::sqrt
or std::cbrt
. In the following example, we raise each element of an int
vector to the arbitrary power of 3
.
#include <cmath>
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::ostream_iterator;
using std::pow;
using std::vector;
template <typename T>
void PrintVector(vector<T> &arr) {
copy(arr.begin(), arr.end(), ostream_iterator<T>(cout, "; "));
cout << endl;
}
constexpr int POWER = 3;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
PrintVector(arr);
for (auto &item : arr) {
item = pow(item, POWER);
}
PrintVector(arr);
return EXIT_SUCCESS;
}
Output:
1; 2; 3; 4; 5; 6; 7; 8; 9; 10;
1; 8; 27; 64; 125; 216; 343; 512; 729; 1000;
Use the Custom Function to Raise a Vector of Numbers to the Power of 2 in C++
Alternatively to the previous method, we can implement various custom functions using the pow
function to extend standard functionality. This example demonstrates a Pow2Vector
function that takes a vector
container and raises its elements to the power of 2. Notice that, PrintVector
function template outputs vector elements to the console, and it can take any vector of built-in data types.
#include <cmath>
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::ostream_iterator;
using std::pow;
using std::vector;
template <typename T>
void PrintVector(vector<T> &arr) {
copy(arr.begin(), arr.end(), ostream_iterator<T>(cout, "; "));
cout << endl;
}
template <typename T>
vector<T> &Pow2Vector(vector<T> &arr) {
for (auto &i : arr) {
i = pow(i, 2);
}
return arr;
}
constexpr int POWER = 3;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
PrintVector(Pow2Vector(arr));
return EXIT_SUCCESS;
}
Output:
1; 4; 9; 16; 25; 36; 49; 64; 81; 100;
Use the Custom Function to Raise a Vector of Numbers to the Power in C++
As a continuation of the previous method, we can tweak the underlying function to take an additional argument of exponent value. This way, we basically have implemented the function that takes the given elements in the vector
to the power we provide. Notice that for the template type deduction to work, you would need to initialize the float
/double
variable as exponent value and then pass it to the PowVector
function.
#include <cmath>
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::ostream_iterator;
using std::pow;
using std::vector;
template <typename T>
void PrintVector(vector<T> &arr) {
copy(arr.begin(), arr.end(), ostream_iterator<T>(cout, "; "));
cout << endl;
}
template <typename T>
vector<T> &PowVector(vector<T> &arr, T power) {
for (auto &i : arr) {
i = pow(i, power);
}
return arr;
}
constexpr int POWER = 3;
int main() {
vector<int> arr = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
vector<float> arr2 = {1.2, 2.3, 3.2, 4.5, 5.5, 6.2, 7.1, 8.2, 9.0, 10.1};
float power = 2.0;
PrintVector(PowVector(arr, 5));
PrintVector(PowVector(arr2, power));
return EXIT_SUCCESS;
}
Output:
1; 32; 243; 1024; 3125; 7776; 16807; 32768; 59049; 100000;
1.44; 5.29; 10.24; 20.25; 30.25; 38.44; 50.41; 67.24; 81; 102.01;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook