How to Generate Random Number in Range in C++
- Using the C++ Standard Library
- Generating Random Floating-Point Numbers
-
Use the
rand
Function to Generate a Random Number in Range - Custom Random Number Generation Function
- Conclusion
- FAQ
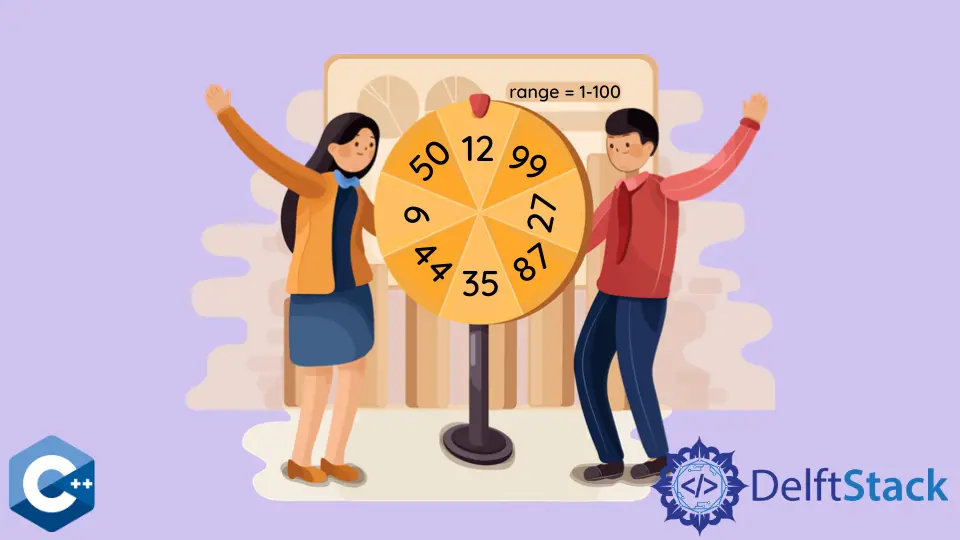
Generating random numbers is a fundamental aspect of programming, particularly when it comes to simulations, games, or any application that requires unpredictability. In C++, generating a random number within a specific range is straightforward, thanks to the powerful Standard Library. Whether you’re creating a simple game or testing algorithms, knowing how to generate random numbers can enhance your project significantly.
In this article, we’ll explore various methods to generate random numbers in a specified range in C++. By the end, you’ll have a clear understanding of how to implement this in your own projects.
Using the C++ Standard Library
One of the simplest and most effective ways to generate random numbers in C++ is by utilizing the <random>
header from the Standard Library. This method provides a robust framework for generating random numbers, ensuring better randomness than older methods.
Here’s how you can do it:
#include <iostream>
#include <random>
int main() {
int lower_bound = 1;
int upper_bound = 100;
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> distr(lower_bound, upper_bound);
int random_number = distr(gen);
std::cout << "Random number: " << random_number << std::endl;
return 0;
}
Output:
Random number: 42
In this code, we first include the necessary headers. We define our lower and upper bounds for the random number. The std::random_device
is a non-deterministic random number generator, which seeds our generator, std::mt19937
. The std::uniform_int_distribution
ensures that our random numbers are uniformly distributed within the specified range. Finally, we generate and print the random number. This method is reliable and should be your go-to for generating random numbers in C++.
Generating Random Floating-Point Numbers
Sometimes, you might need a random floating-point number instead of an integer. The process is quite similar, but we’ll use std::uniform_real_distribution
instead of std::uniform_int_distribution
. Let’s see how this works:
#include <iostream>
#include <random>
int main() {
double lower_bound = 1.0;
double upper_bound = 10.0;
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_real_distribution<> distr(lower_bound, upper_bound);
double random_number = distr(gen);
std::cout << "Random floating-point number: " << random_number << std::endl;
return 0;
}
Output:
Random floating-point number: 5.678
In this example, we define our lower and upper bounds as doubles. The std::uniform_real_distribution
generates random floating-point numbers within this range. The rest of the code remains largely unchanged, demonstrating the flexibility of the C++ Standard Library in handling different data types. This approach is particularly useful in simulations where decimal precision is essential.
Use the rand
Function to Generate a Random Number in Range
The rand
function is part of the C standard library and can be called from the C++ code. Although it’s not recommended to use the rand
function for high-quality random number generation, it can be utilized to fill arrays or matrices with arbitrary data for different purposes. In this example, the function generates a random integer between 0 and MAX
number interval. Note that this function should be seeded with std::srand
(preferably passing the current time with std::time(nullptr)
) to generate different values across the multiple runs, and only then we can call the rand
.
#include <ctime>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < RAND_NUMS_TO_GENERATE; i++) cout << rand() % MAX << "; ";
cout << endl;
return EXIT_SUCCESS;
}
Output (*random):
36; 91; 99; 40; 3; 60; 90; 63; 44; 22;
Custom Random Number Generation Function
If you prefer a more customized approach, you can create your own function to generate random numbers. This can be particularly useful if you need to incorporate additional logic or specific requirements into your random number generation.
Here’s an example of a custom function:
#include <iostream>
#include <random>
int generateRandomNumber(int lower_bound, int upper_bound) {
std::random_device rd;
std::mt19937 gen(rd());
std::uniform_int_distribution<> distr(lower_bound, upper_bound);
return distr(gen);
}
int main() {
int lower = 1;
int upper = 50;
int random_number = generateRandomNumber(lower, upper);
std::cout << "Random number from custom function: " << random_number << std::endl;
return 0;
}
Output:
Random number from custom function: 23
In this code, we define a function generateRandomNumber
that takes two parameters: lower_bound
and upper_bound
. Inside the function, we set up the random number generator and distribution just like before. This allows you to call this function anywhere in your code, making it reusable and cleaner. This method is particularly useful if you have multiple places in your code that need random numbers.
Conclusion
Generating random numbers in C++ is a straightforward task, especially with the help of the Standard Library. Whether you need integers, floating-point numbers, or a custom solution, C++ provides the tools necessary to achieve your goals. By understanding these methods, you can enhance your programming projects, making them more dynamic and interesting. So, the next time you’re faced with the need for randomness, you’ll know exactly how to implement it effectively in your C++ code.
FAQ
-
How do I generate a random number between two specific values in C++?
You can use the<random>
header and thestd::uniform_int_distribution
orstd::uniform_real_distribution
to generate random numbers within a specified range. -
Is the random number generation in C++ truly random?
The random number generation in C++ is pseudo-random, meaning it uses algorithms to generate sequences that appear random. For true randomness, you can usestd::random_device
. -
Can I generate random numbers in a specific distribution other than uniform?
Yes, C++ provides various distributions in the<random>
header, including normal, binomial, and Poisson distributions, allowing for more complex random number generation. -
What is the difference between
std::random_device
andstd::mt19937
?
std::random_device
is used for seeding the random number generator and produces non-deterministic random numbers, whilestd::mt19937
is a specific pseudo-random number generator that produces deterministic sequences based on its seed. -
Can I use random number generation in multithreading?
Yes, but you should ensure that each thread has its own instance of the random number generator to avoid contention and ensure thread safety.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook