Seed Random Number Generator in C++
-
Seed Random Number Generator in C++ Using the
srand()
Function -
Seed Random Number Generator in C++ Using the
time()
Function -
Seed Random Number Generator in C++ Using
std::random_device
andstd::mt19937
- Avoid Seed Random Number Generator Mistakes in C++
- Conclusion
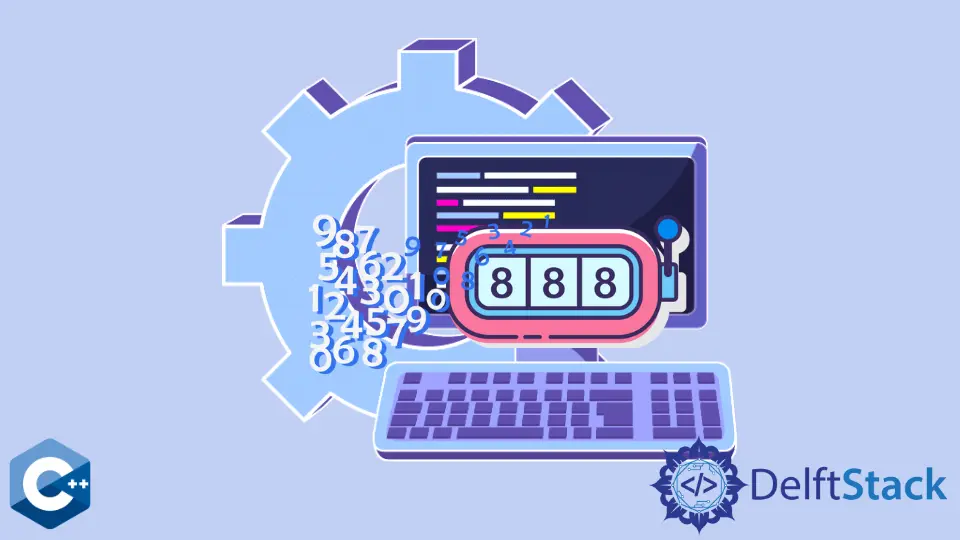
Random number generation is a fundamental aspect of many applications, ranging from simulations and games to cryptography and scientific research. In C++, the ability to generate pseudo-random numbers is essential, and the process begins with the initialization of a random number generator.
Seeding, the act of providing an initial value to the generator, plays a pivotal role in determining the sequence of pseudo-random numbers produced.
This article explores the methods available for seeding random number generation in C++. From manual input using the srand()
function to dynamic seeding with the time()
function and advanced approaches involving std::random_device
and std::mt19937
, we will delve into the syntax, mechanisms, and practical applications of each method.
Seed Random Number Generator in C++ Using the srand()
Function
The rand()
function in C++ generates a pseudo-random integer in the range [0, RAND_MAX]
. It is essential to note that without seeding, the sequence of numbers generated by rand()
will be the same every time the program runs.
This lack of randomness can be problematic in scenarios where diverse and unpredictable values are required.
The syntax of srand()
is as follows:
void srand(unsigned int seed);
The srand()
function is part of the <cstdlib>
header in C++. It takes an unsigned integer, seed
, as its argument, which serves as the initial value for the random number generator algorithm.
If the same seed is used in subsequent executions, the generator will produce the same sequence of pseudo-random numbers. This deterministic behavior is often useful for testing and debugging scenarios.
Now, let’s explore a complete working example to illustrate the usage of srand()
.
#include <cstdlib> // For srand() and rand() functions
#include <iostream>
int main() {
unsigned int seed;
std::cout << "Enter seed value: ";
std::cin >> seed;
srand(seed); // Seed the random number generator with user-provided value
// Generate and print a sequence of pseudo-random numbers
for (int i = 0; i < 5; ++i) {
std::cout << rand() << " ";
}
return 0;
}
In this example, we start by including the necessary header <cstdlib>
to access the srand()
and rand()
functions. We then declare an unsigned integer variable, seed
, to store the user’s input for seeding the random number generator.
The user is prompted to enter a seed value using std::cin
, and the provided value is used as an argument for the srand()
function, initializing the random number generator.
Next, we use a simple loop to generate and print a sequence of five pseudo-random numbers using the rand()
function. The sequence is determined by the seeded algorithm, ensuring reproducibility when the same seed is used.
Assuming the user enters the seed value as 42
, the output might look like this:
Enter seed value: 42
71876166 708592740 1483128881 907283241 442951012
Each execution with the same seed value will produce the same sequence of pseudo-random numbers. This level of control is valuable when predictable randomness is required for testing or simulations.
By understanding and utilizing the srand()
function effectively, developers can introduce determinism into their random number generation processes, offering both reliability and predictability as needed.
Seed Random Number Generator in C++ Using the time()
Function
Another popular method to seed the random number generator is by utilizing the time()
function. Unlike manually inputting a seed, using the time()
function provides a dynamic and often unique seed value based on the current system time.
This approach ensures a different seed for each program execution, promoting variability in the generated random sequences.
Here is the syntax of time()
:
time_t time(time_t* timer);
The time()
function, found in the <ctime>
header, returns the current UNIX timestamp, representing the number of seconds elapsed since 00:00 hours on January 1, 1970, UTC
. It takes a pointer to a time_t
object as an optional argument, allowing storage of the timestamp value.
When used for seeding, time()
provides a constantly changing seed based on the current time, ensuring a different seed value with each program execution. This dynamic nature contributes to generating diverse sequences of pseudo-random numbers.
Now, let’s proceed with a comprehensive working example to illustrate how to seed the random number generator using the time()
function.
#include <cstdlib> // For srand() and rand() functions
#include <ctime> // For time() function
#include <iostream>
int main() {
srand(time(NULL)); // Seed the random number generator with current time
// Generate and print a sequence of pseudo-random numbers
for (int i = 0; i < 5; ++i) {
std::cout << rand() << " ";
}
return 0;
}
In this example, we include the <cstdlib>
and <ctime>
headers to access the required functions. We then use srand(time(NULL))
to seed the random number generator. By passing NULL
to the time()
function, we instruct it to return the current timestamp.
The subsequent loop generates and prints a sequence of five pseudo-random numbers using the rand()
function. The use of the time()
function for seeding ensures a different seed value for each program execution, leading to distinct random number sequences.
The output of the program will vary each time it is executed due to the dynamic seed provided by the time()
function. An example output might look like this:
1608637542 3421126067 411672537 284972843 2645257605
By leveraging the time()
function, developers introduce an element of unpredictability into their random number generation, making it suitable for scenarios where variability is crucial, such as simulations or games. This method ensures that each run of the program generates a unique sequence of pseudo-random numbers based on the current system time.
Seed Random Number Generator in C++ Using std::random_device
and std::mt19937
The <random>
header in C++ introduces powerful tools for random number generation. Using std::random_device
in conjunction with std::mt19937
allows developers to create a robust and unpredictable seeding mechanism.
This method provides high-quality random numbers and is often preferred over other approaches for its reliability.
Here’s the basic structure of the usage of std::random_device
and std::mt19937
:
#include <random>
int main() {
std::random_device rd;
std::mt19937 gen(rd());
// Use 'gen' to generate random numbers
return 0;
}
The <random>
header introduces std::random_device
, which is a hardware-based random number generator if available, and std::mt19937
, a versatile and high-quality pseudo-random number generator (Mersenne Twister). The std::random_device
is used to seed the std::mt19937
generator.
std::random_device
provides a source of entropy, ensuring a high-quality seed for random number generation. This source can be hardware-based, making it more reliable than some other seeding methods.
The std::mt19937
generator, initialized with the value from std::random_device
, then produces sequences of pseudo-random numbers with excellent statistical properties.
Now, let’s proceed with a comprehensive working example to illustrate how to seed the random number generator using std::random_device
and std::mt19937
.
#include <iostream>
#include <random>
int main() {
std::random_device rd;
std::mt19937 gen(rd());
// Generate and print a sequence of pseudo-random numbers
for (int i = 0; i < 5; ++i) {
std::cout << gen() << " ";
}
return 0;
}
In this example, we include the <random>
header to access the necessary classes. We declare a std::random_device
object named rd
to provide a source of entropy. We then initialize an std::mt19937
generator, named gen
, with the value from the random device.
The subsequent loop utilizes the operator()
of the std::mt19937
generator to generate and print a sequence of five pseudo-random numbers. This method ensures high-quality randomness due to the combination of a reliable entropy source and the Mersenne Twister algorithm.
The output of the program will vary each time it is executed, as the std::random_device
provides a new seed based on available entropy. An example output might look like this:
1608637542 3421126067 411672537 284972843 2645257605
By using std::random_device
and std::mt19937
, we can achieve a robust and unpredictable seeding mechanism, ideal for scenarios where high-quality randomness is critical, such as cryptography or scientific simulations. This method provides a balance between performance and randomness quality, making it a preferred choice in many applications.
Avoid Seed Random Number Generator Mistakes in C++
While seeding random number generation in C++ is a crucial step, it is equally essential to be aware of common mistakes that can lead to unintended outcomes. Mistakes in seeding can result in repeated sequences, lack of unpredictability, or compromise the statistical properties of generated numbers.
Seeding Inside Loops
One common mistake is seeding the random number generator inside a loop.
This can lead to the generator using the same seed repeatedly, resulting in the generation of identical sequences in each iteration. This defeats the purpose of having a varied and unpredictable sequence.
In order to avoid this, it’s crucial to seed the generator outside the loop, ensuring that a new seed is used only once.
Incorrect Example:
#include <cstdlib>
#include <ctime>
#include <iostream>
int main() {
for (int i = 1; i <= 10; i++) {
srand(time(NULL));
std::cout << rand() << " ";
}
std::cout << std::endl;
return 0;
}
In this example, the random number generator is seeded inside the loop, resulting in the same sequence being produced ten times.
Output:
1239757040 1239757040 1239757040 1239757040 1239757040 1239757040 1239757040 1239757040 1239757040 1239757040
Corrected Example:
#include <cstdlib>
#include <ctime>
#include <iostream>
int main() {
srand(time(NULL));
for (int i = 1; i <= 10; i++) {
std::cout << rand() << " ";
}
std::cout << std::endl;
return 0;
}
By seeding the generator outside the loop, a different sequence is generated in each iteration.
Output:
1135248420 562980222 782646998 2015263389 709668959 500269040 1111655425 1529064613 1904669997 1278773416
Using Time as Seed Too Quickly
When using the time()
function for seeding, be cautious about seeding too quickly, especially within loops. The time()
function returns the number of seconds since a fixed point in the past, and if called rapidly, it may return the same value in consecutive loop iterations.
To mitigate this, consider incorporating a delay or using a more dynamic seed source.
Incorrect Example:
#include <cstdlib>
#include <ctime>
#include <iostream>
int main() {
for (int i = 1; i <= 10; i++) {
srand(time(NULL));
std::cout << rand() << " ";
}
std::cout << std::endl;
return 0;
}
Output:
1432308063 1432308063 1432308063 1432308063 1432308063 1432308063 1432308063 1432308063 1432308063 1432308063
In this example, the loop executes quickly, and the same value is passed to time()
in each iteration, resulting in the same seed.
Corrected Example:
#include <cstdlib>
#include <ctime>
#include <iostream>
int main() {
srand(time(NULL));
for (int i = 1; i <= 10; i++) {
std::cout << rand() << " ";
}
std::cout << std::endl;
return 0;
}
Output:
94070436 949985211 314309839 1911538714 1965606815 72137843 1527691791 2114167525 1850311534 590655915
Seeding the generator outside the loop addresses this issue, ensuring a different seed for each iteration.
By being mindful of these common mistakes, you can enhance the robustness and unpredictability of your random number generation in C++. Paying attention to where and how you seed your generators ensures that the generated sequences meet the desired characteristics for your specific application.
Conclusion
Seeding the random number generator in C++ is a significant step to ensure the generation of diverse and unpredictable sequences of pseudo-random numbers.
We explored three methods for seeding random number generation in C++:
- Using
srand()
with manual input - Using the
time()
function for dynamic seeding - Using
std::random_device
in combination withstd::mt19937
for high-quality randomness
Each method comes with its advantages and use cases, providing developers with flexibility and control over the randomness of their programs.
When using srand()
, developers can manually input seed values, enabling reproducibility for testing scenarios. However, caution is needed to avoid common pitfalls, such as seeding within loops, which can lead to repeating sequences.
The time()
function offers dynamic seeding by utilizing the current system time. This method ensures a different seed for each program execution, introducing an element of unpredictability into the generated sequences.
For applications requiring high-quality randomness, the combination of std::random_device
and std::mt19937
is a robust choice. The hardware-based entropy source provided by std::random_device
ensures a reliable seed for the Mersenne Twister algorithm in std::mt19937
, resulting in sequences with excellent statistical properties.