C++에서 범위 내 난수 생성
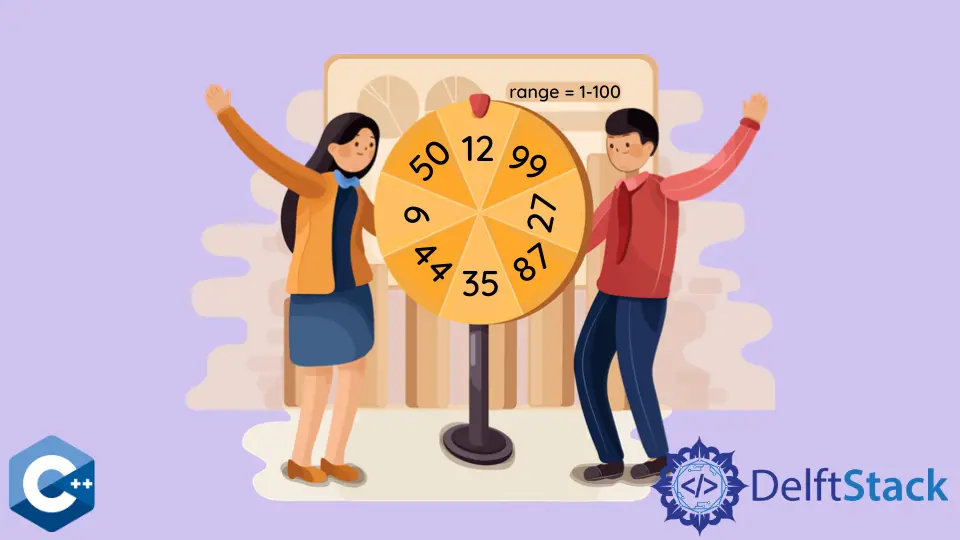
이 기사에서는 지정된 숫자 간격으로 난수를 생성하는 방법에 대한 여러 C++ 메서드를 소개합니다.
C++ 11<random>
라이브러리를 사용하여 범위 내 난수 생성
C++는 새로운 헤더<random>
아래에 C++ 11 릴리스로 난수 생성을위한 표준 라이브러리 기능을 추가했습니다. <random>
헤더에서 제공하는 RNG 워크 플로우 기능은 랜덤 엔진과 배포의 두 부분으로 나뉩니다. 랜덤 엔진은 예측할 수없는 비트 스트림을 반환합니다. 분포는 특정 확률 분포를 만족하는 난수 (사용자가 지정한 유형)를 반환합니다. 균일, 정상 또는 기타.
처음에 사용자는 시드 값으로 랜덤 엔진을 초기화해야합니다. 비 결정적 랜덤 비트에 대한 시스템 별 소스 인std::random_device
로 엔진을 시드하는 것이 좋습니다. 이를 통해 엔진은 각 실행에서 서로 다른 임의의 비트 스트림을 생성 할 수 있습니다. 반면에 사용자가 여러 프로그램 실행에서 동일한 시퀀스를 생성해야하는 경우 임의 엔진은 상수int
리터럴로 초기화해야합니다.
다음으로, 분포 객체는 난수가 생성되는 간격에 대한 최소/최대 값의 인수로 초기화됩니다. 다음 예에서는uniform_int_distribution
을 사용하고 임의로 콘솔에 10 개의 정수를 출력합니다.
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int n = 0; n < RAND_NUMS_TO_GENERATE; ++n) {
cout << distr(eng) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력 (* 무작위) :
57; 38; 8; 69; 5; 27; 65; 65; 73; 4;
<random>
헤더는 서로 다른 알고리즘 및 효율성 절충안을 가진 여러 임의 엔진을 제공합니다. 따라서 다음 코드 샘플과 같이 특정 임의 엔진을 초기화 할 수 있습니다.
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::random_device rd;
std::mt19937 eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int n = 0; n < RAND_NUMS_TO_GENERATE; ++n) {
cout << distr(eng) << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
출력 (* 무작위) :
59; 47; 81; 41; 28; 88; 10; 12; 86; 7;
rand
함수를 사용하여 범위 내 난수 생성
rand
함수는 C 표준 라이브러리의 일부이며 C++ 코드에서 호출 할 수 있습니다. 고품질 난수 생성을 위해rand
함수를 사용하는 것은 권장되지 않지만 다양한 목적을 위해 임의의 데이터로 배열 또는 행렬을 채우는 데 사용할 수 있습니다. 이 예에서 함수는 0과 MAX
숫자 간격 사이의 임의의 정수를 생성합니다. 이 함수는 std::srand
(바람직하게는 현재 시간을 std::time(nullptr)
로 전달)로 시드하여 여러 실행에 걸쳐 다른 값을 생성해야합니다. 그런 다음에 만 rand
를 호출 할 수 있습니다.
#include <ctime>
#include <iostream>
#include <random>
using std::cout;
using std::endl;
constexpr int MIN = 1;
constexpr int MAX = 100;
constexpr int RAND_NUMS_TO_GENERATE = 10;
int main() {
std::srand(std::time(nullptr));
for (int i = 0; i < RAND_NUMS_TO_GENERATE; i++) cout << rand() % MAX << "; ";
cout << endl;
return EXIT_SUCCESS;
}
출력 (* 무작위) :
36; 91; 99; 40; 3; 60; 90; 63; 44; 22;
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook