How to Use Exponential Functions of STL in C++
-
Use the
std::exp
Function to Calculate Powers of Euler’s Number -
Use the
std::exp2
Function to Calculate Powers of Two -
Use the
std::pow
Function to Calculate Powers of the Given Number -
Use
std::log
Function to Calculate Natural Logarithm of the Given Number
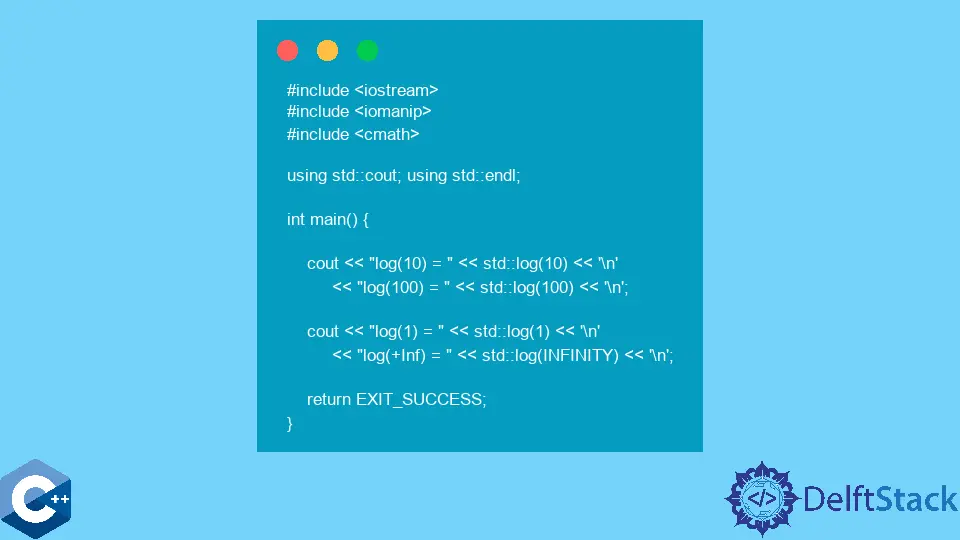
This article will demonstrate STL functions for calculating the exponential in C++.
Use the std::exp
Function to Calculate Powers of Euler’s Number
The std::exp
function is part of the <cmath>
header along with many common mathematical functions. The former calculates Euler’s number raised to the given power, which is passed as the only argument.
The std::exp
function has multiple overloads for float
, double
, long double
, and even integral type, but the latter one still returns a double
floating-point value. If the overflow occurs, one of the following values is returned +HUGE_VAL
, +HUGE_VALF
, or +HUGE_VALL
.
Note that there are special return values for several arguments like +-0
, +-INFINITY
, and NaN
. All these cases are shown in the following example code.
#include <cmath>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
int main() {
cout << "exp(1) = e¹ = " << std::setprecision(16) << std::exp(1) << '\n'
<< "exp(10) = " << std::exp(10) << '\n'
<< "exp(100) = " << std::exp(100) << '\n';
cout << "exp(-0) = " << std::exp(-0.0) << '\n'
<< "exp(+Inf) = " << std::exp(+INFINITY) << '\n'
<< "exp(NaN) = " << std::exp(NAN) << '\n'
<< "exp(-Inf) = " << std::exp(-INFINITY) << '\n';
return EXIT_SUCCESS;
}
Output:
exp(1) = e¹ = 2.718281828459045
exp(10) = 22026.46579480672
exp(100) = 2.688117141816136e+43
exp(-0) = 1
exp(+Inf) = inf
exp(NaN) = nan
exp(-Inf) = 0
Use the std::exp2
Function to Calculate Powers of Two
On the other hand, we have the std::exp2
function to calculate two’s powers. The overloads of this function return floating-point values but can accept integral types as well. Notice that std::exp2
has similar special values for arguments like +-0
, +-INFINITY
, and NaN
.
#include <cmath>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
int main() {
cout << "exp2(4) = " << std::exp2(4) << '\n'
<< "exp2(0.5) = " << std::exp2(0.5) << '\n'
<< "exp2(10) = " << std::exp2(10) << '\n';
cout << "exp2(-0) = " << std::exp2(-0.0) << '\n'
<< "exp2(+Inf) = " << std::exp2(+INFINITY) << '\n'
<< "exp2(NaN) = " << std::exp2(NAN) << '\n'
<< "exp2(-Inf) = " << std::exp2(-INFINITY) << '\n';
return EXIT_SUCCESS;
}
Output:
exp2(4) = 16
exp2(0.5) = 1.41421
exp2(10) = 1024
exp2(-0) = 1
exp2(+Inf) = inf
exp2(NaN) = nan
exp2(-Inf) = 0
Use the std::pow
Function to Calculate Powers of the Given Number
The std::pow
function is used to compute the value of the number raised to the given power. Both the base number and power value are specified as the first and the second arguments, respectively.
std::pow
has multiple overloads for floating-point types as well as integral values, but the latter ones are cast to double
type and can even be promoted to the long double
if one of the arguments is also long double
. Also, note that std::pow
can not be used to calculate a root of a negative number.
#include <cmath>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
int main() {
cout << "pow(2, 10) = " << std::pow(2, 10) << '\n'
<< "pow(10, 0.5) = " << std::pow(10, 0.5) << '\n'
<< "pow(-25, -2) = " << std::pow(-25, -2) << '\n';
cout << "pow(-1, NAN) = " << std::pow(-1, NAN) << '\n'
<< "pow(+1, NAN) = " << std::pow(+1, NAN) << '\n'
<< "pow(INFINITY, 2) = " << std::pow(INFINITY, 2) << '\n'
<< "pow(INFINITY, -1) = " << std::pow(INFINITY, -1) << '\n';
return EXIT_SUCCESS;
}
Output:
pow(2, 10) = 1024
pow(10, 0.5) = 3.16228
pow(-25, -2) = 0.0016
pow(-1, NAN) = nan
pow(+1, NAN) = 1
pow(INFINITY, 2) = inf
pow(INFINITY, -1) = 0
Use std::log
Function to Calculate Natural Logarithm of the Given Number
std::log
family of functions are also provided in <cmath>
to calculate various logarithms for the given numerical values. std::log
function computes the natural logarithm, and similar to the previous functions, it has multiple overloads for floating-point and integral types.
#include <cmath>
#include <iomanip>
#include <iostream>
using std::cout;
using std::endl;
int main() {
cout << "log(10) = " << std::log(10) << '\n'
<< "log(100) = " << std::log(100) << '\n';
cout << "log(1) = " << std::log(1) << '\n'
<< "log(+Inf) = " << std::log(INFINITY) << '\n';
return EXIT_SUCCESS;
}
Output:
log(10) = 2.30259
log(100) = 4.60517
log(1) = 0
log(+Inf) = inf
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook