How to Use Timer in C++
-
Use the
clock()
Function to Implement a Timer in C++ -
Use the
gettimeofday
Function to Implement a Timer in C++
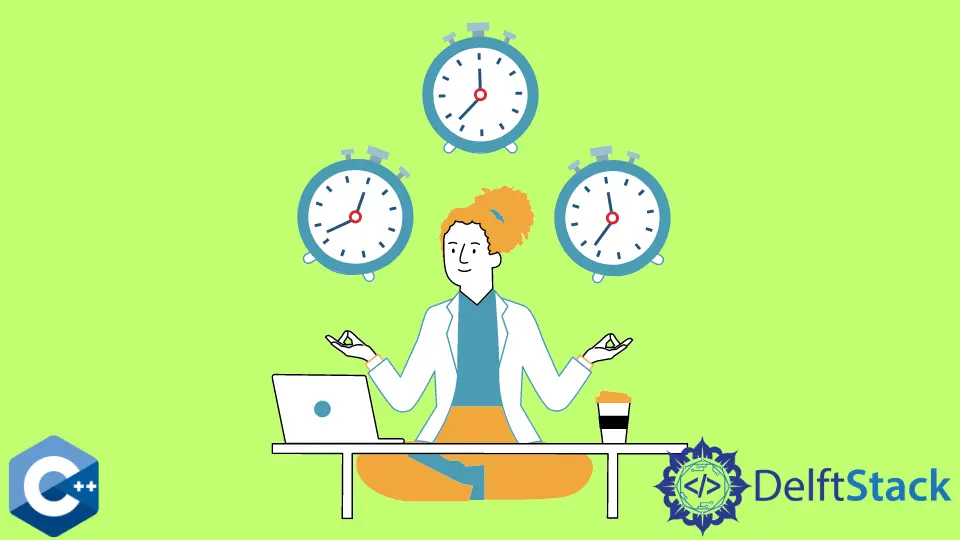
This article will demonstrate multiple methods of how to use a timer in C++.
Use the clock()
Function to Implement a Timer in C++
The clock()
function is a POSIX compliant method to retrieve the program’s processor time. The function returns the integer value that needs to be divided by a macro-defined constant called CLOCKS_PER_SEC
to convert to several seconds.
The following sample code implements two functions for finding the maximum value in an int
array. The max_index
function does the searching based on the index of the elements, whereas the max_value
is value-based. The goal is to calculate how much time they spend on finding the maximum int
value in the 1,000,000
element array filled with random integers.
Notice that we call the clock()
function twice - before the max_index
call and then after the call. This time-measuring scheme can be employed generally without any regard to the specific function that retrieves the time. In this case, we may see (depending on the hardware system) that max_value
gets the job done slightly faster than index-based search. Mind though, these maximum search algorithms have O(N)
complexity and should not be employed in any professional codebase except for maybe experimentation.
#include <chrono>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
constexpr int WIDTH = 1000000;
int main() {
clock_t start, end;
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
start = clock();
max = max_index(arr, WIDTH);
end = clock();
printf("max_index: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
start = clock();
max = max_value(arr, WIDTH);
end = clock();
printf("max_value: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
exit(EXIT_SUCCESS);
}
Output:
max_value: 0.00131400 sec, max = 2147480499
max_value: 0.00089800 sec, max = 2147480499
Use the gettimeofday
Function to Implement a Timer in C++
gettimeofday
is a highly accurate time retrieval function in Linux based systems, which can be called from the C++ source code as well. The function was designed to get the time and timezone data, but the latter has been depreciated for some time, and the second argument should be nullptr
instead of the valid timezone struct
. gettimeofday
stores the timing data in the special struct
called timeval
, that contains two data members tv_sec
representing seconds and tv_usec
for microseconds.
As a general rule, we declare and initialize the two timeval
structures before calling the function gettimeofday
. Once the function gets called, the data should be stored successfully in the corresponding struct
if the return value of gettimeofday
is 0
. Otherwise, the failure is indicated by returning -1
value. Notice that, after the structs are filled with data, it needs conversion to time’s common unit value. This sample code implements the time_diff
function that returns the time in seconds, which can be outputted to the console as needed.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
constexpr int WIDTH = 1000000;
int main() {
struct timeval start {};
struct timeval end {};
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
gettimeofday(&start, nullptr);
max = max_index(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_index: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
gettimeofday(&start, nullptr);
max = max_value(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_value: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
exit(EXIT_SUCCESS);
}
Output:
max_value: 0.00126000 sec, max = 2147474877
max_value: 0.00093900 sec, max = 2147474877
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook