在 C++ 中使用定時器
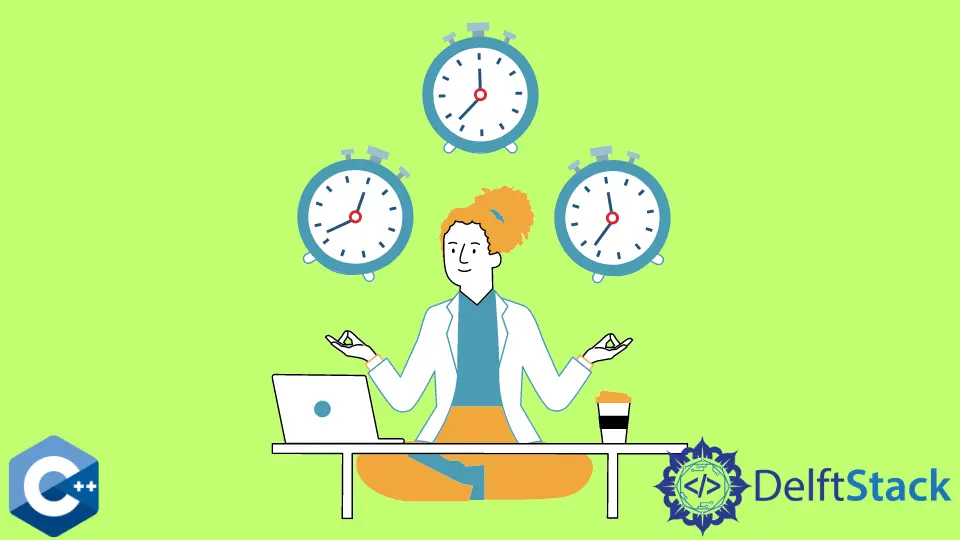
本文將演示如何在 C++ 中使用定時器的多種方法。
使用 clock()
函式在 C++ 中實現一個定時器
clock()
函式是一個符合 POSIX 標準的方法,用於檢索程式的處理器時間。該函式返回需要除以一個名為 CLOCKS_PER_SEC
的巨集定義常數的整數值,以轉換為幾秒鐘。
下面的示例程式碼實現了兩個函式,用於查詢 int
陣列中的最大值。max_index
函式是基於元素的索引進行搜尋,而 max_value
則是基於值進行搜尋。我們的目標是計算他們花了多少時間在充滿隨機整數的 1,000,000
元素陣列中尋找最大值。
注意,我們呼叫了兩次 clock()
函式-在呼叫 max_index
之前,然後在呼叫之後。通常可以採用這種時間測量方案,而不必考慮檢索時間的具體函式。在這種情況下,我們可能會看到(取決於硬體系統),max_value
比基於索引的搜尋更快地完成工作。不過要注意,這些最大搜尋演算法有 O(N)
的複雜度,不應該在任何專業的程式碼庫中採用,僅僅用於實驗中。
#include <chrono>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
constexpr int WIDTH = 1000000;
int main() {
clock_t start, end;
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
start = clock();
max = max_index(arr, WIDTH);
end = clock();
printf("max_index: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
start = clock();
max = max_value(arr, WIDTH);
end = clock();
printf("max_value: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
exit(EXIT_SUCCESS);
}
輸出:
max_value: 0.00131400 sec, max = 2147480499
max_value: 0.00089800 sec, max = 2147480499
使用 gettimeofday
函式在 C++ 中實現一個定時器
gettimeofday
是基於 Linux 系統的高精度時間檢索函式,也可以從 C++ 原始碼中呼叫。該函式的設計初衷是為了獲取時間和時區資料,但後者已經被棄用一段時間了,第二個引數應該是 nullptr
而不是有效的時區 struct
。gettimeofday
將時區資料儲存在名為 timeval
的特殊結構體中,它包含兩個資料成員:tv_sec
代表秒,tv_usec
代表微秒。
一般來說,在呼叫函式 gettimeofday
之前,我們先宣告並初始化這兩個 timeval
結構。一旦函式被呼叫,如果 gettimeofday
的返回值是 0
,那麼資料應該成功地儲存在相應的結構體中。否則,以返回 -1
值表示失敗。注意,結構體中充滿資料後,需要轉換為時間的通用單位值。本示例程式碼實現了 time_diff
函式,該函式以秒為單位返回時間,可以根據需要輸出到控制檯。
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
constexpr int WIDTH = 1000000;
int main() {
struct timeval start {};
struct timeval end {};
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
gettimeofday(&start, nullptr);
max = max_index(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_index: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
gettimeofday(&start, nullptr);
max = max_value(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_value: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
exit(EXIT_SUCCESS);
}
輸出:
max_value: 0.00126000 sec, max = 2147474877
max_value: 0.00093900 sec, max = 2147474877