C++에서 타이머 사용
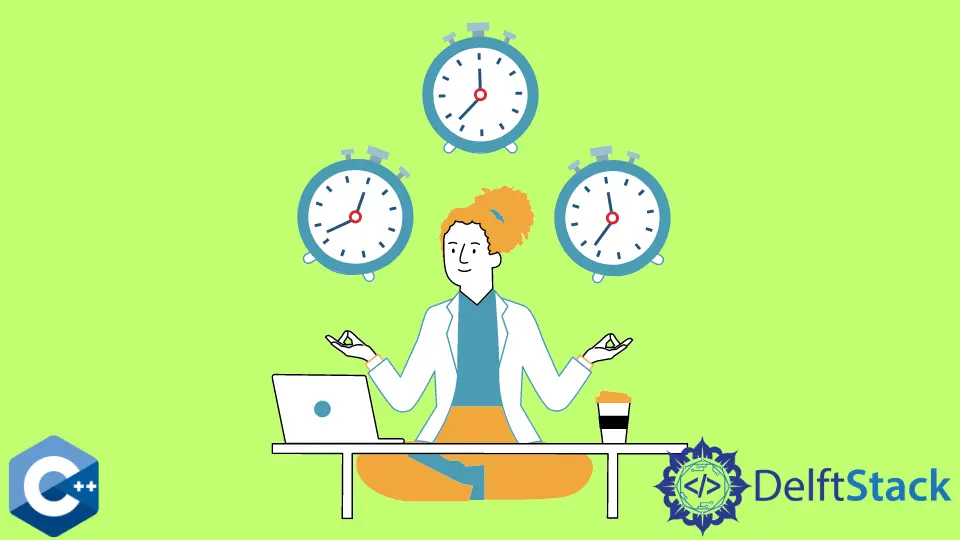
이 기사에서는 C++에서 타이머를 사용하는 방법에 대한 여러 방법을 보여줍니다.
clock()
함수를 사용하여 C++에서 타이머 구현
clock()
함수는 프로그램의 프로세서 시간을 검색하는 POSIX 호환 메소드입니다. 이 함수는 매크로 정의 상수CLOCKS_PER_SEC
로 나누어 몇 초로 변환해야하는 정수 값을 반환합니다.
다음 샘플 코드는int
배열에서 최대 값을 찾기위한 두 가지 함수를 구현합니다. max_index
함수는 요소의 색인을 기반으로 검색을 수행하는 반면max_value
는 값 기반입니다. 목표는 임의의 정수로 채워진1,000,000
요소 배열에서 최대int
값을 찾는 데 소요되는 시간을 계산하는 것입니다.
clock()
함수를max_index
호출 전과 호출 후 두 번 호출합니다. 이 시간 측정 방식은 일반적으로 시간을 검색하는 특정 기능에 관계없이 사용할 수 있습니다. 이 경우 (하드웨어 시스템에 따라)max_value
가 인덱스 기반 검색보다 약간 더 빠르게 작업을 수행하는 것을 볼 수 있습니다. 하지만 이러한 최대 검색 알고리즘은 복잡도가 O(N)
이므로 실험을 제외하고는 전문 코드베이스에서 사용해서는 안됩니다.
#include <chrono>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
constexpr int WIDTH = 1000000;
int main() {
clock_t start, end;
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
start = clock();
max = max_index(arr, WIDTH);
end = clock();
printf("max_index: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
start = clock();
max = max_value(arr, WIDTH);
end = clock();
printf("max_value: %0.8f sec, max = %d\n",
((float)end - start) / CLOCKS_PER_SEC, max);
exit(EXIT_SUCCESS);
}
출력:
max_value: 0.00131400 sec, max = 2147480499
max_value: 0.00089800 sec, max = 2147480499
gettimeofday
함수를 사용하여 C++에서 타이머 구현
gettimeofday
는 Linux 기반 시스템에서 매우 정확한 시간 검색 기능으로 C++ 소스 코드에서도 호출 할 수 있습니다. 이 함수는 시간 및 시간대 데이터를 가져 오도록 설계되었지만 후자는 한동안 감가 상각되었으며 두 번째 인수는 유효한 시간대 struct
대신 nullptr
이어야합니다. gettimeofday
는 초를 나타내는 두 개의 데이터 멤버tv_sec
와 마이크로 초를 나타내는tv_usec
를 포함하는timeval
이라는 특수struct
에 타이밍 데이터를 저장합니다.
일반적으로 gettimeofday
함수를 호출하기 전에 두 개의 timeval
구조를 선언하고 초기화합니다. 함수가 호출되면gettimeofday
의 반환 값이0
이면 해당struct
에 데이터가 성공적으로 저장되어야합니다. 그렇지 않으면 -1
값을 반환하여 실패를 표시합니다. 구조체가 데이터로 채워진 후에는 시간의 공통 단위 값으로 변환해야합니다. 이 샘플 코드는 시간을 초 단위로 반환하는 time_diff
함수를 구현하며, 필요에 따라 콘솔에 출력 할 수 있습니다.
#include <sys/time.h>
#include <ctime>
#include <iostream>
using std::cout;
using std::endl;
int max_index(int arr[], int size) {
size_t max = 0;
for (int j = 0; j < size; ++j) {
if (arr[j] > arr[max]) {
max = j;
}
}
return arr[max];
}
int max_value(int arr[], int size) {
int max = arr[0];
for (int j = 0; j < size; ++j) {
if (arr[j] > max) {
max = arr[j];
}
}
return max;
}
float time_diff(struct timeval *start, struct timeval *end) {
return (end->tv_sec - start->tv_sec) + 1e-6 * (end->tv_usec - start->tv_usec);
}
constexpr int WIDTH = 1000000;
int main() {
struct timeval start {};
struct timeval end {};
int max;
int *arr = new int[WIDTH];
std::srand(std::time(nullptr));
for (size_t i = 0; i < WIDTH; i++) {
arr[i] = std::rand();
}
gettimeofday(&start, nullptr);
max = max_index(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_index: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
gettimeofday(&start, nullptr);
max = max_value(arr, WIDTH);
gettimeofday(&end, nullptr);
printf("max_value: %0.8f sec, max = %d\n", time_diff(&start, &end), max);
exit(EXIT_SUCCESS);
}
출력:
max_value: 0.00126000 sec, max = 2147474877
max_value: 0.00093900 sec, max = 2147474877
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook