C++ Template Multiple Types
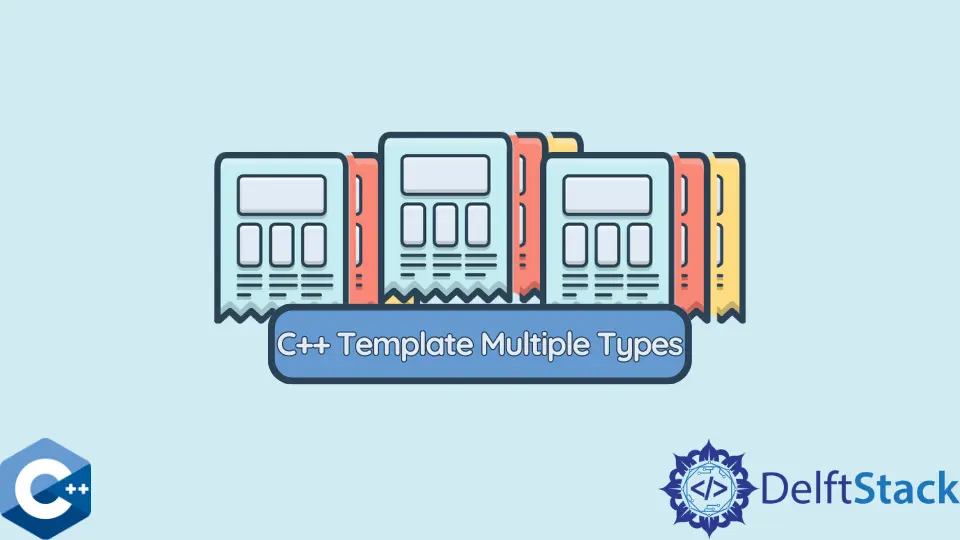
This tutorial demonstrates the use of multiple-type templates in C++.
C++ Template Multiple Types
The templates in C++ can be defined as the blueprint of the formulas to create generic functions and classes. The templates are simple but powerful tools in C++, where the idea is to pass the data type to the parameters so we may not write the same code again for different data types.
The templates are expanded like macros at the compile time. The only difference is that the compiler will check the data types before expanding the templates.
The templates can be defined as where the source code only contains classes or functions, but compiler code will contain multiple copies of these classes or functions.
Now the multiple-type template can be defined as a member template of a class which is also a template and is defined outside its class template definition; this class definition should be specified with the template parameters of the class template followed by member template parameters.
There are two types of templates in C++.
Function Template
A function template can work with multiple data types simultaneously, whereas a standard function cannot. The syntax for the function template is below.
template <class DataType>
Returntype FunctionName(parameter list) {
// function body
}
Let’s try a simple example of a function template.
#include <iostream>
using namespace std;
// This template function will work for all data types/ it will return the
// minimum value from given parameters
template <typename Delftstack>
Delftstack MinValue(Delftstack a, Delftstack b) {
return (a < b) ? a : b;
}
int main() {
cout << MinValue<int>(78, 354) << endl; // Call the template function for int
cout << MinValue<double>(336.23, 4673.23)
<< endl; // Call the template function for double
cout << MinValue<char>('s', 'y')
<< endl; // Call the template function for char
return 0;
}
The above contains a template function, MinValue
, which will work for all data types and return the minimum value from given values. See the output:
78
336.23
s
Class Template
The class template also knows the generic templates work similarly to the function templates. The class templates define a family of classes in C++; the syntax for the class template is below.
template <class Ttype>
class ClassName {
// class body;
}
Now let’s try an example using the class template.
#include <iostream>
using namespace std;
template <typename Delftstack>
class DemoArray {
private:
Delftstack* ptr;
int arraysize;
public:
DemoArray(Delftstack arr[], int number);
void print();
};
template <typename Delftstack>
DemoArray<Delftstack>::DemoArray(Delftstack arr[], int number) {
ptr = new Delftstack[number];
arraysize = number;
for (int x = 0; x < arraysize; x++) ptr[x] = arr[x];
}
template <typename Delftstack>
void DemoArray<Delftstack>::print() {
for (int x = 0; x < arraysize; x++) cout << " " << *(ptr + x);
cout << endl;
}
int main() {
int arr[7] = {23, 12, 43, 74, 55, 36, 67};
DemoArray<int> demo(arr, 7);
demo.print();
return 0;
}
The code above creates a template array class and a template
method to print the members of an array; we defined the member template outside the template
class. See the output:
23 12 43 74 55 36 67
Class Template With Multiple Types
A class template with multiple types means we have to define a class template with multiple parameters, which is possible with C++. The syntax to define a class template with multiple types is below.
template <class Delft1, class Delft2, class Delft3 = char>
class DemoClassTemplate {
// class body
}
Let’s jump to the example to understand the class template with multiple types.
#include <iostream>
using namespace std;
// Class template containing multiple type parameters
template <class Delft1, class Delft2, class Delft3 = char>
class DemoClassTemplate {
private:
Delft1 value1;
Delft2 value2;
Delft3 value3;
public:
DemoClassTemplate(Delft1 v1, Delft2 v2, Delft3 v3)
: value1(v1), value2(v2), value3(v3) {} // constructor
void printVar() {
cout << "The Value 1 = " << value1 << endl;
cout << "The Value 2 = " << value2 << endl;
cout << "The Value 3 = " << value3 << endl;
}
};
int main() {
// object with int, double and char types using the template class
DemoClassTemplate<int, double> DemoObject1(57, 34.7, 'Delftstack');
cout << "DemoObject 1 Values: " << endl;
DemoObject1.printVar();
// object with int, double and boolean types using the template class
DemoClassTemplate<double, char, bool> DemoObject2(789.8, 'Delft', true);
cout << "\nDemoObject 2 values: " << endl;
DemoObject2.printVar();
return 0;
}
As we can see, the code above creates a template class with multiple type parameters that contain default parameters. Now, we can create objects of this template
class and use any data types we want.
Output:
DemoObject 1 Values:
The Value 1 = 57
The Value 2 = 34.7
The Value 3 = k
DemoObject 2 values:
The Value 1 = 789.8
The Value 2 = t
The Value 3 = 1
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook