C++ テンプレートの複数のタイプ
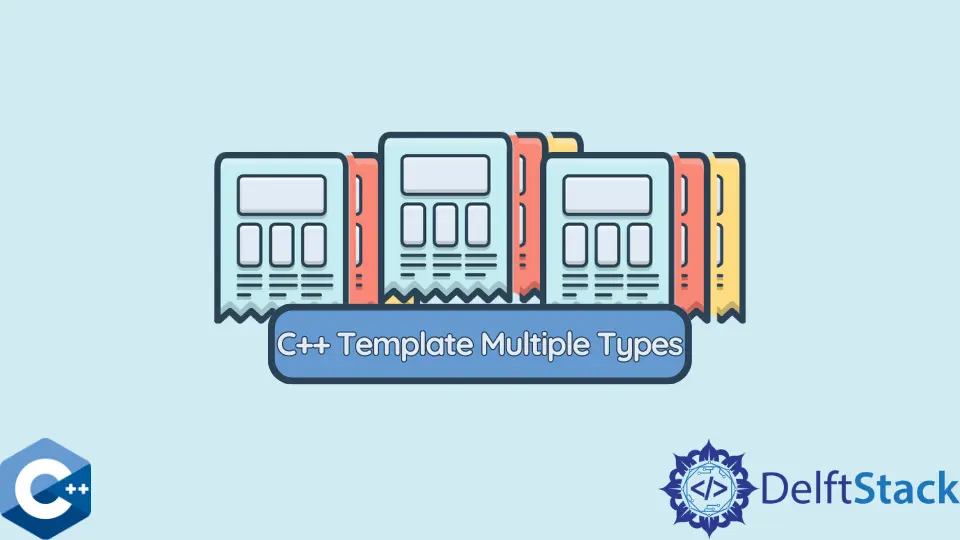
このチュートリアルでは、C++ で複数型のテンプレートを使用する方法を示します。
C++ テンプレートの複数のタイプ
C++ のテンプレートは、一般的な関数とクラスを作成するための数式の青写真として定義できます。 テンプレートは単純ですが、C++ の強力なツールであり、データ型をパラメーターに渡すことを目的としているため、異なるデータ型に対して同じコードを再度記述する必要はありません。
テンプレートは、コンパイル時にマクロのように展開されます。 唯一の違いは、コンパイラがテンプレートを展開する前にデータ型をチェックすることです。
テンプレートは、ソース コードにクラスまたは関数のみが含まれている場所として定義できますが、コンパイラ コードにはこれらのクラスまたは関数の複数のコピーが含まれます。
複数型テンプレートは、テンプレートでもあり、そのクラス テンプレート定義の外で定義されるクラスのメンバー テンプレートとして定義できるようになりました。 このクラス定義は、クラス テンプレートのテンプレート パラメータの後にメンバ テンプレート パラメータを続けて指定する必要があります。
C++ には 2 種類のテンプレートがあります。
関数テンプレート
関数テンプレートは複数のデータ型を同時に処理できますが、標準関数はできません。 関数テンプレートの構文は次のとおりです。
template <class DataType>
Returntype FunctionName(parameter list) {
// function body
}
関数テンプレートの簡単な例を試してみましょう。
#include <iostream>
using namespace std;
// This template function will work for all data types/ it will return the
// minimum value from given parameters
template <typename Delftstack>
Delftstack MinValue(Delftstack a, Delftstack b) {
return (a < b) ? a : b;
}
int main() {
cout << MinValue<int>(78, 354) << endl; // Call the template function for int
cout << MinValue<double>(336.23, 4673.23)
<< endl; // Call the template function for double
cout << MinValue<char>('s', 'y')
<< endl; // Call the template function for char
return 0;
}
上記には、すべてのデータ型で機能し、指定された値から最小値を返すテンプレート関数 MinValue
が含まれています。 出力を参照してください。
78
336.23
s
クラス テンプレート
クラス テンプレートは、汎用テンプレートが関数テンプレートと同様に機能することも認識しています。 クラス テンプレートは、C++ でクラスのファミリを定義します。 クラス テンプレートの構文は次のとおりです。
template <class Ttype>
class ClassName {
// class body;
}
それでは、クラス テンプレートを使用した例を試してみましょう。
#include <iostream>
using namespace std;
template <typename Delftstack>
class DemoArray {
private:
Delftstack* ptr;
int arraysize;
public:
DemoArray(Delftstack arr[], int number);
void print();
};
template <typename Delftstack>
DemoArray<Delftstack>::DemoArray(Delftstack arr[], int number) {
ptr = new Delftstack[number];
arraysize = number;
for (int x = 0; x < arraysize; x++) ptr[x] = arr[x];
}
template <typename Delftstack>
void DemoArray<Delftstack>::print() {
for (int x = 0; x < arraysize; x++) cout << " " << *(ptr + x);
cout << endl;
}
int main() {
int arr[7] = {23, 12, 43, 74, 55, 36, 67};
DemoArray<int> demo(arr, 7);
demo.print();
return 0;
}
上記のコードは、配列のメンバーを出力するテンプレート配列クラスと template
メソッドを作成します。 template
クラスの外でメンバー テンプレートを定義しました。 出力を参照してください。
23 12 43 74 55 36 67
複数の型を持つクラス テンプレート
複数の型を持つクラス テンプレートは、複数のパラメーターを持つクラス テンプレートを定義する必要があることを意味します。これは C++ で可能です。 複数の型を持つクラス テンプレートを定義する構文は次のとおりです。
template <class Delft1, class Delft2, class Delft3 = char>
class DemoClassTemplate {
// class body
}
例にジャンプして、複数の型を持つクラス テンプレートを理解しましょう。
#include <iostream>
using namespace std;
// Class template containing multiple type parameters
template <class Delft1, class Delft2, class Delft3 = char>
class DemoClassTemplate {
private:
Delft1 value1;
Delft2 value2;
Delft3 value3;
public:
DemoClassTemplate(Delft1 v1, Delft2 v2, Delft3 v3)
: value1(v1), value2(v2), value3(v3) {} // constructor
void printVar() {
cout << "The Value 1 = " << value1 << endl;
cout << "The Value 2 = " << value2 << endl;
cout << "The Value 3 = " << value3 << endl;
}
};
int main() {
// object with int, double and char types using the template class
DemoClassTemplate<int, double> DemoObject1(57, 34.7, 'Delftstack');
cout << "DemoObject 1 Values: " << endl;
DemoObject1.printVar();
// object with int, double and boolean types using the template class
DemoClassTemplate<double, char, bool> DemoObject2(789.8, 'Delft', true);
cout << "\nDemoObject 2 values: " << endl;
DemoObject2.printVar();
return 0;
}
ご覧のとおり、上記のコードは、デフォルト パラメーター を含む複数の型パラメーターを持つテンプレート クラスを作成します。 これで、この template
クラスのオブジェクトを作成して、必要なデータ型を使用できます。
出力:
DemoObject 1 Values:
The Value 1 = 57
The Value 2 = 34.7
The Value 3 = k
DemoObject 2 values:
The Value 1 = 789.8
The Value 2 = t
The Value 3 = 1
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook