C++ Run Command Line
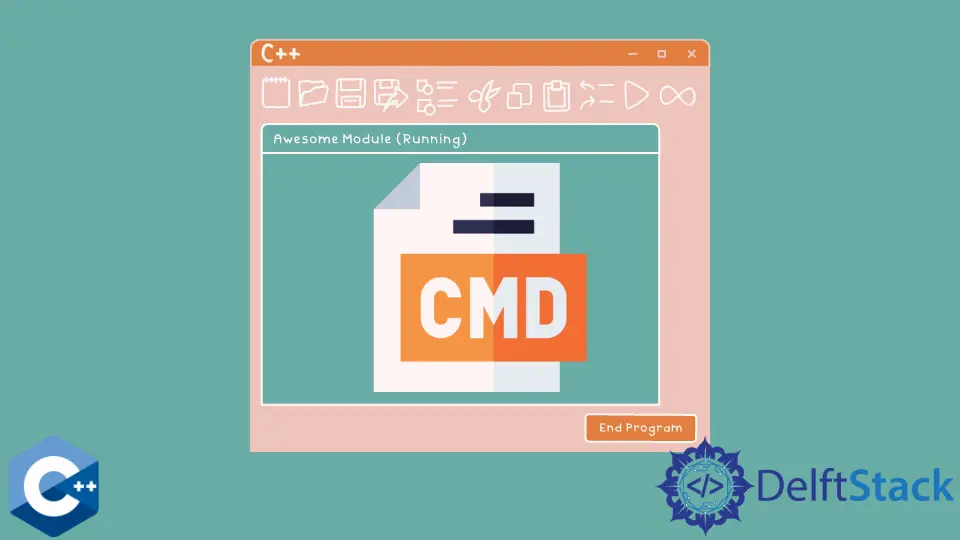
This article will briefly discuss the system()
method to run commands in a command processor through a C++ program.
C/C++ provides a function that can do this without taking the burden of spawning another process that can run the command line processor to run the CMD commands.
Use the system()
Method to Run Command-Line Commands in C++
The system()
function has long been a part of the C standard library, and we can also include it in C++ code without requiring additional libraries. This function runs a shell command from the calling process.
It should be noted, however, that the system()
is designed for specific use cases in which the calling program needs to generate a single child process and quickly start waiting for it to get terminated.
As a result, the function is implemented using multiple system calls available as part of the system API interface.
In the Linux environment, the programmer doesn’t need to take the headache of the fork
, exec
, and wait
functions that control the creation and deletion of new child processes.
Syntax, Parameters & Return Values of the system()
Method in C++
The syntax of this function is:
int system(const char* cmd_text);
The sole parameter passed to the system()
function is a char
array containing the command text. The command processor executes this command text.
Remember that we can pass a Null
pointer as a parameter to this function to check the availability of the command processor.
If a command is passed as a parameter to this function, the system()
usually returns that command’s exit
status. Still, sometimes the return value depends on the command and utility.
Suppose a Null
pointer is passed to check the availability of the command processor. In that case, it returns a 0
if the command processor is unavailable and a non-zero value otherwise.
Example Codes to Demonstrate C++ Run Command Line
The following example shows the most basic application of the system()
function, which runs the date
command-line utility to print the current system date.
#include <stdlib.h>
#include <iostream>
using namespace std;
int main() {
system("date");
return 0;
}
It will generate the following output:
Sat Sep 10 09:17:19 PM UTC 2022
Let us look at another example, in which we will first check if the command processor is present or not and then will run the ls -l
command using the system()
function.
#include <stdlib.h>
#include <iostream>
using namespace std;
int main() {
if (system(NULL)) {
cout << "Command Processor OK" << endl;
cout << "Running ls command" << endl;
system("ls -l");
} else
cout << "Command Processor not found!!" << endl;
return 0;
}
It will give the following output:
Command Processor OK
Running ls command
total 24
-rwxr-xr-x 1 root root 16744 Sep 10 21:18 jdoodle
-rw-r--r-- 1 root root 315 Sep 10 21:18 jdoodle.cpp