How to Convert Radians to Degrees in C++
- Using a Conversion Factor to Convert Radians to Degrees in C++
- Using a Function to Convert Radians to Degrees in C++
- Using a Function with Math Library to Convert Radians to Degrees in C++
- Using a Preprocessor Directive to Convert Radians to Degrees in C++
- Conclusion
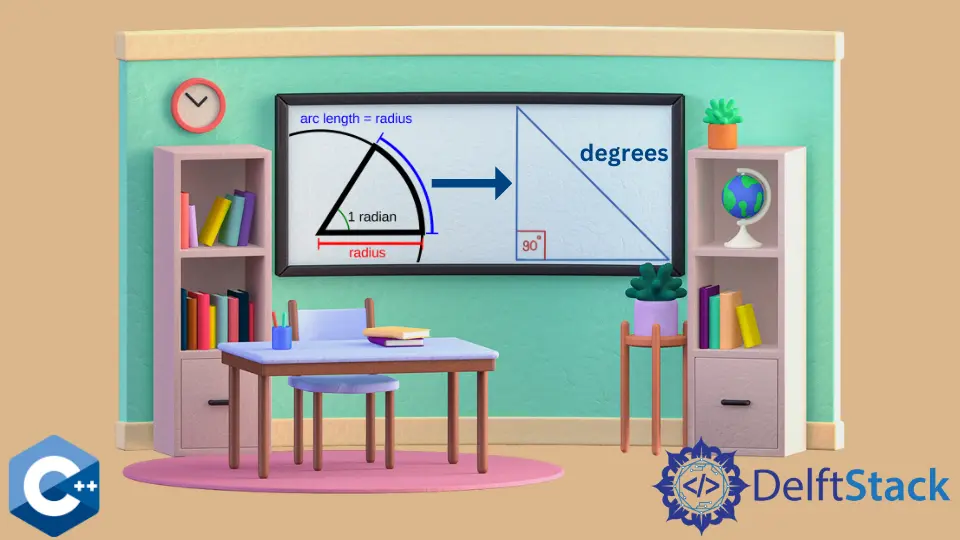
Radian and degree are two different units of measurement for angles. Radians measure the angle in terms of the arc length on a circle, while degree measures it as 360/2π
.
Radians are more convenient when working with small angles since they appear as a fraction of π
. Degrees, on the other hand, are easier to use when dealing with large angles.
In this article, we will discuss the conversion of radians into degrees in C++.
Using a Conversion Factor to Convert Radians to Degrees in C++
The concept of this method is based on the relationship between radians and degrees.
There are 180
degrees in π
radians. Therefore, to convert radians to degrees, you multiply the radians by a conversion factor of (180.0 / π)
.
To understand better, let’s look at an example.
#include <iostream>
#include <cmath>
int main() {
// Define the value of pi
const double pi = 3.14159265358979323846;
// Input value of the radians
double radians = 1;
// Conversion using the conversion factor
double degrees = radians * (180.0 / pi);
// Output the result
std::cout << "Radians: " << radians << std::endl;
std::cout << "Degrees: " << degrees << std::endl;
return 0;
}
Output:
Radians: 1
Degrees: 57.2958
In the example, the program starts by defining the value of pi
(π
) using a constant (const double pi
). Next, the radians
variable that is to be converted is initialized and is set with a value of 1
.
Then, the conversion is done by multiplying the radians
by the conversion factor of (180.0 / pi)
. Lastly, the result is printed to the console using std::cout
.
This method is straightforward and easy to understand. It explicitly incorporates the conversion factor in the code, making the relationship between radians and degrees clear.
Using a Function to Convert Radians to Degrees in C++
Using a function to convert radians to degrees in C++ involves encapsulating the conversion logic into a reusable function.
The idea is to create a function that takes a value in radians as an input parameter and returns the corresponding value in degrees. This encapsulation of logic into a function promotes code modularity and reusability.
Example:
#include <iostream>
#include <cmath>
// Function to convert radians to degrees
double radiansToDegrees(double radians) {
const double pi = 3.14159265358979323846;
return radians * (180.0 / pi);
}
int main() {
// Input: Value in radians
double radians = 1;
// Call the function for conversion
double degrees = radiansToDegrees(radians);
// Output the result
std::cout << "Radians: " << radians << std::endl;
std::cout << "Degrees: " << degrees << std::endl;
return 0;
}
Output:
Radians: 1
Degrees: 57.2958
In this example, the program first declares a function named radiansToDegrees
that takes a double
parameter radians
. Inside the function, it calculates and returns the equivalent value in degrees using the conversion factor in the previous method discussed.
In the main
function, the radiansToDegrees
function is called to convert the radians
value, and the result is assigned to the degrees
variable. Finally, the program outputs the value of radians
and degrees
to the console using std::cout
.
This method makes the conversion logic reusable, allowing you to easily convert radians to degrees by calling the function with different values. It enhances code readability and maintainability.
Using a Function with Math Library to Convert Radians to Degrees in C++
The concept of this method is similar to using a function, but instead of defining a custom constant for pi
, this method uses the M_PI
constant provided by the cmath
library. This constant represents the mathematical constant π
(pi
) and simplifies the code by avoiding the need to define pi
manually.
Here’s an example code showing how it is used:
#include <iostream>
#include <cmath>
// Function to convert radians to degrees using M_PI constant
double radiansToDegrees(double radians) {
return radians * (180.0 / M_PI);
}
int main() {
// Input: Value in radians
double radians = 1;
// Call the function for conversion
double degrees = radiansToDegrees(radians);
// Output the result
std::cout << "Radians: " << radians << std::endl;
std::cout << "Degrees: " << degrees << std::endl;
return 0;
}
Output:
Radians: 1
Degrees: 57.2958
In the example, a function named radiansToDegrees
takes a double
parameter radians
. Inside the function, it calculates and returns the equivalent value in degrees using the M_PI
constant and the conversion factor (180.0 / π)
.
In the main function, the radiansToDegrees
is called, and its result is stored inside the degrees
variable. Then, the values of both radians
and degrees
are printed to the console using std::cout
.
This method simplifies the code by using the M_PI
constant, making it more concise and expressive. It still offers the benefits of encapsulating the conversion logic into a function for reusability.
Using a Preprocessor Directive to Convert Radians to Degrees in C++
Using a preprocessor directive to convert radians to degrees in C++ involves defining a constant value for pi
using #define
and then using it in a function or expression. The defined constant can then be used throughout the program to simplify calculations involving pi
.
Example:
#include <iostream>
#define pi 3.14159265358979323846
// Function to convert radians to degrees using preprocessor-defined pi
float Convert(float radian) {
return (radian * (180 / pi));
}
int main() {
// Input: Value in radians
float radians = 1;
// Call the function for conversion
float degrees = Convert(radians);
// Output the result
std::cout << "Radians: " << radians << std::endl;
std::cout << "Degrees: " << degrees << std::endl;
return 0;
}
Output:
Radians: 1
Degrees: 57.2958
In the example code, a preprocessor directive is used to define a constant named pi
with the value of the mathematical constant π
.
Next, a function named Convert
that takes a float
parameter radian
is declared. Inside the function, it calculates and returns the equivalent value in degrees using the preprocessor-defined pi
constant and the conversion factor (180 / pi)
.
In the main
function, the Convert
function is called, and its result is stored in the degrees
variable. Then, the values of radians
and degrees
variables are printed to the console using std::cout
.
This method provides a way to use a preprocessor directive to define a constant (pi
), making the code more readable and reducing the need for manual pi
value input in multiple places. However, it’s important to use such directives judiciously to avoid potential issues with code maintainability.
Conclusion
This article delves into practical ways to convert radians to degrees in C++. The four main methods of converting radians to degrees in C++ are then discussed in detail.
Throughout the article, clear code examples and outputs are provided, making it accessible to readers with different coding preferences. The diverse methods presented offer a well-rounded guide for efficiently handling the common task of converting radians to degrees in C++.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook