How to Overload Subscript Operator in C++
- Understanding Operator Overloading
- Overloading Subscript Operator for Constant Objects
- Benefits of Overloading the Subscript Operator
- Conclusion
- FAQ
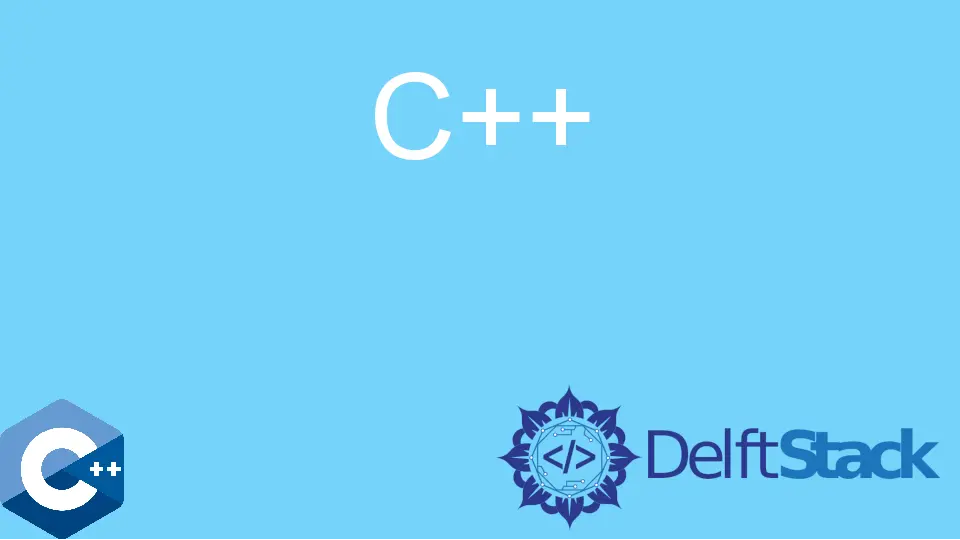
Overloading the subscript operator in C++ can significantly enhance the usability of your classes, especially when you want to create custom data structures. This operator allows you to access elements using the familiar array syntax, making your code cleaner and more intuitive.
In this article, we will explore how to overload the subscript operator, providing practical examples and explanations to help you understand the concept thoroughly. Whether you’re developing a custom container or simply want to enhance an existing class, mastering this technique will elevate your C++ programming skills. Let’s dive into the details of operator overloading and see how you can implement it in your projects.
Understanding Operator Overloading
Operator overloading in C++ allows you to define how operators work with user-defined types. The subscript operator, represented by operator[]
, is particularly useful for classes that need to behave like arrays. By overloading this operator, you can enable array-like access to your class objects, which can make them more intuitive to use.
The syntax for overloading the subscript operator is straightforward. You define a member function within your class that returns a reference to the data type you want to access. This function takes an integer (or another suitable type) as an argument, which represents the index.
Here’s a basic example of how to overload the subscript operator:
#include <iostream>
#include <vector>
class MyArray {
private:
std::vector<int> arr;
public:
MyArray(int size) : arr(size) {}
int& operator[](int index) {
return arr[index];
}
};
int main() {
MyArray myArray(5);
myArray[0] = 10;
myArray[1] = 20;
std::cout << myArray[0] << ", " << myArray[1] << std::endl;
return 0;
}
In this example, we create a class MyArray
that contains a vector of integers. The overloaded operator[]
function returns a reference to the element at the specified index. This allows us to use the familiar array syntax to access and modify the elements of MyArray
.
Output:
10, 20
The MyArray
class can now be used like a regular array, making it easier to work with. The overloaded operator provides a clear and concise way to access elements, enhancing code readability.
Overloading Subscript Operator for Constant Objects
In C++, it’s often necessary to access elements of a class in a read-only manner, especially when dealing with constant objects. To achieve this, you can overload the subscript operator for constant objects as well. This involves creating a second version of the operator[]
function that returns a constant reference.
Here’s how you can implement this:
#include <iostream>
#include <vector>
class MyArray {
private:
std::vector<int> arr;
public:
MyArray(int size) : arr(size) {}
int& operator[](int index) {
return arr[index];
}
const int& operator[](int index) const {
return arr[index];
}
};
int main() {
MyArray myArray(5);
myArray[0] = 10;
myArray[1] = 20;
const MyArray constArray = myArray;
std::cout << constArray[0] << ", " << constArray[1] << std::endl;
return 0;
}
In this code, we added a second operator[]
function that is marked as const
. This allows constant instances of MyArray
to access its elements without modifying them. The first version of the operator allows modification, while the second ensures that constant objects can still be accessed.
Output:
10, 20
By implementing both versions of the subscript operator, you can provide flexibility in how your class is used, accommodating both mutable and immutable contexts.
Benefits of Overloading the Subscript Operator
Overloading the subscript operator provides several benefits that can enhance your C++ programming experience. Here are some of the key advantages:
-
Improved Readability: Using the subscript operator allows for cleaner and more intuitive code. Instead of calling methods to access elements, you can use the familiar array syntax.
-
Flexibility: By overloading the operator, you can define custom behavior for accessing elements, such as bounds checking or returning default values for out-of-bounds indices.
-
Enhanced Functionality: You can extend the operator to work with different types, enabling complex data structures like matrices or custom collections.
-
Ease of Use: Developers familiar with array syntax will find it easier to work with your custom classes, reducing the learning curve.
Here’s an example that demonstrates bounds checking in the overloaded operator:
#include <iostream>
#include <vector>
#include <stdexcept>
class MyArray {
private:
std::vector<int> arr;
public:
MyArray(int size) : arr(size) {}
int& operator[](int index) {
if (index < 0 || index >= arr.size()) {
throw std::out_of_range("Index out of bounds");
}
return arr[index];
}
};
int main() {
MyArray myArray(5);
myArray[0] = 10;
try {
std::cout << myArray[10] << std::endl; // This will throw an exception
} catch (const std::out_of_range& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
In this example, the subscript operator checks if the index is within bounds before accessing the array. If the index is invalid, it throws an exception, enhancing the robustness of your code.
Output:
Index out of bounds
By incorporating such features into your overloaded operators, you can create more reliable and user-friendly classes.
Conclusion
Overloading the subscript operator in C++ is a powerful technique that can greatly improve the usability of your classes. By allowing array-like access, you make your code more intuitive and easier to read. Whether you are implementing custom data structures or simply enhancing existing ones, understanding how to overload this operator is essential for any C++ programmer. With the examples and explanations provided in this article, you should now have a solid foundation to implement operator overloading in your own projects.
FAQ
-
What is operator overloading in C++?
Operator overloading allows you to define custom behavior for operators in user-defined types, enabling intuitive operations on objects. -
Why would I want to overload the subscript operator?
Overloading the subscript operator allows you to access elements of a class using array syntax, improving code readability and usability. -
Can I overload the subscript operator for constant objects?
Yes, you can overload the subscript operator for constant objects by providing a separateconst
version of the operator. -
What happens if I access an out-of-bounds index?
If you implement bounds checking in your overloaded operator, you can throw an exception or handle the error gracefully when an out-of-bounds index is accessed. -
Is operator overloading mandatory in C++?
No, operator overloading is not mandatory. It is a feature that can enhance the usability of your classes but should be used judiciously.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook