C++ Get Variable Type
- C++ Get Variable Type
-
Use the
typeid
Operator to Find the Type of a Variable in C++ -
Use the
decltype()
Specifier to Find the Type of a Variable in C++ - Conclusion
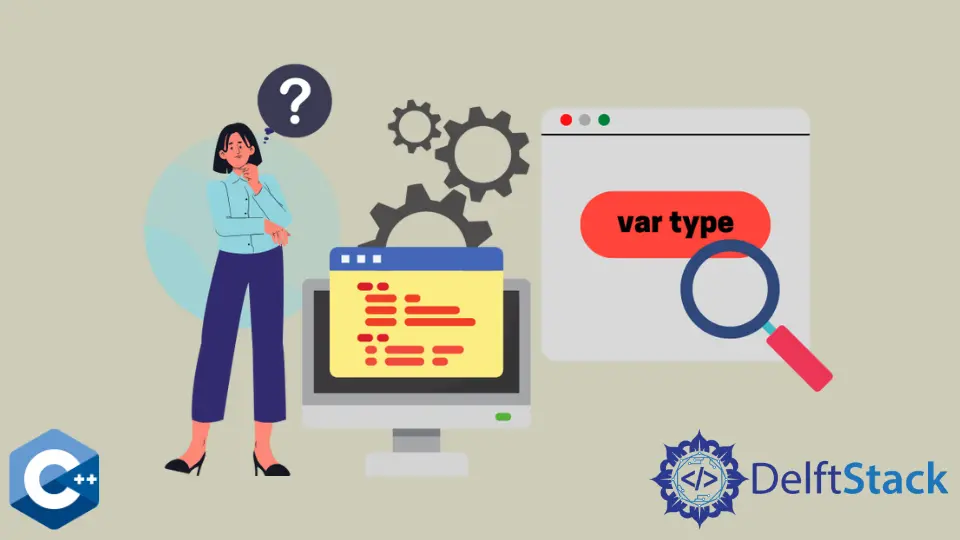
This article will explore the different methods of finding a variable’s data type in the standard C++.
C++ Get Variable Type
There are scenarios where we need to find the dynamic type or the type at the runtime of the variables in C++. Therefore, the C++ libraries have provided us with different methods for finding the type of a variable.
One of the methods is to use the typeid
operator, which is present in the <typeinfo>
library of C++. However, the other one has been introduced in C++11, known as the decltype(x)
, which converts an expression into a type of the result produced.
In the older versions of C++, each data type must be explicitly declared at the compile-time; however, in the newer versions, there is a facility for the automatic deduction of the data types in an expression which is known as type inference. The decltype()
operator also provides the facility of automatic deduction of the data types in an expression.
However, we will be discussing each of the methods in detail in this article.
Use the typeid
Operator to Find the Type of a Variable in C++
The typeid
operator is present in the <typeinfo>
library of C++ and is used to find the variable type provided at runtime. However, the complete function used to find the variable type is typeid(x).name()
, where x
is the variable whose type should be found.
The name()
member function used returns a character sequence that identifies the type of the variable.
However, it will return the initials of the data type as the output. For example, for int
, it will return i
, for float
f
, and for double
, it will return d
as the type.
Let us see the code using the typeid
operator in C++ to find the type of a variable.
#include <iostream>
#include <typeinfo>
using namespace std;
int main() {
int x = 200;
float y = 200.790;
cout << typeid(x).name() << endl;
cout << typeid(y).name() << endl;
cout << typeid(x * y).name() << endl;
return 0;
}
Output:
i
f
f
The output above shows that i
refers to the integer
type, whereas f
refers to the float
type. Moreover, the typeid
in C++ is also used to return the type of an expression.
In the above example, the expression passed in the typeid
operator is the multiplication of the two different data types, int
and float
; therefore, the result will be converted to the larger data type.
Use the decltype()
Specifier to Find the Type of a Variable in C++
The decltype(x)
specifier was introduced with C++11. It is an operator used to get the type of the resultant expression.
The auto
keyword is used to declare a variable with a particular type, whereas the decltype
extracts that variable type. Both the auto
and decltype
are based on the type inference that refers to the automatic deduction of the data types.
Let us see an example of using the decltype
operator to find the variable type in C++.
#include <bits/stdc++.h>
#include <cstring>
using namespace std;
float function1() { return 4.5; }
int function2() { return 34; }
int main() {
decltype(function1()) a;
decltype(function2()) b;
cout << typeid(a).name() << endl;
cout << typeid(b).name() << endl;
return 0;
}
Output:
f
i
The data types of the variables a
and b
in the above example will be the same as the return type of function1
and function2
.
Another example of the decltype
operator is below, in which it extracts the type of the expression in C++.
#include <bits/stdc++.h>
using namespace std;
int main() {
float ft = 4.8;
decltype(ft) a = ft + 9.8;
cout << typeid(a).name();
return 0;
}
Output:
f
The output f
refers to the float
data type. Therefore, after adding the expression ft+9.8
, the resultant data type will be float
.
However, a major difference between the typeid
and decltype
operators is that the typeid
provides the information about the type at runtime, whereas the decltype
provides the type information at the compile time.
Conclusion
In this article, we have discussed how to get the type of a variable in C++. We have discussed two different methods; one is to use the typeid(x).name()
, which provides the type at runtime, whereas the other is the decltype(x)
, which provides the type at compile time.
However, both these methods are efficient and easy to use.