POD Type in C++
- What are POD Types?
- Characteristics of POD Types
- How to Define a POD Type
- Advantages of Using POD Types
- When to Use POD Types
- Conclusion
- FAQ
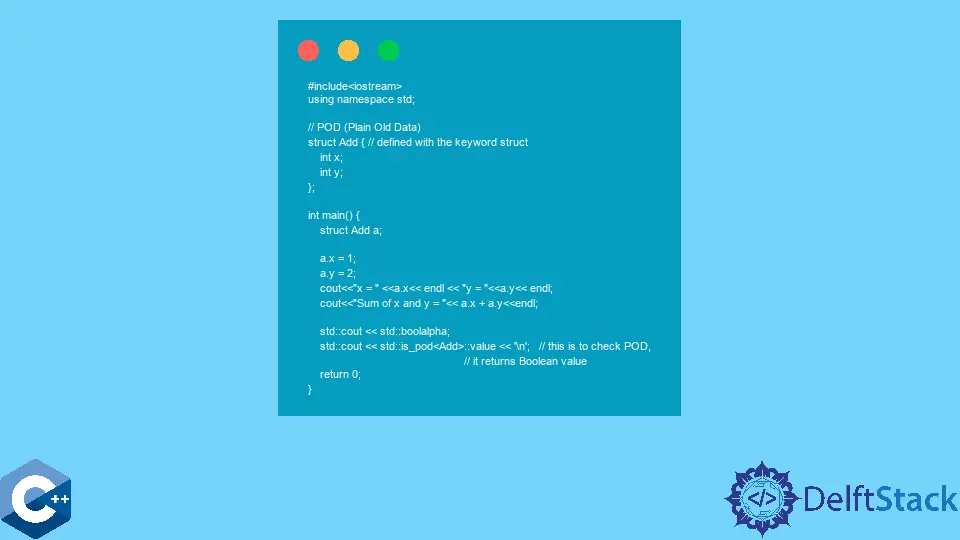
When diving into the world of C++, one term that often surfaces is POD, which stands for Plain Old Data. This concept is crucial for developers looking to understand how data structures work in a straightforward manner. POD types are essentially classes or structures defined using the struct
or class
keywords, but they lack constructors, destructors, and virtual functions. This simplicity allows for efficient memory usage and straightforward data manipulation.
In this article, we will explore POD types in C++, their significance, and how they can be effectively utilized in your coding practices.
What are POD Types?
POD types are a special category of data types in C++. They are characterized by their simplicity and lack of complex features. A POD type can be defined using either a struct
or a class
, but it must adhere to specific rules to qualify as a POD. Notably, POD types cannot have user-defined constructors, destructors, or virtual functions. This makes them similar to C-style structs, where the focus is solely on data representation rather than behavior.
The primary advantage of using POD types is their compatibility with C-style data manipulation, which can lead to performance gains. They are also easier to use in scenarios involving low-level memory operations, such as interfacing with hardware or optimizing data storage. Understanding POD types is essential for any C++ developer, especially when performance and memory management are critical.
Characteristics of POD Types
To fully grasp the concept of POD types, let’s break down their defining characteristics:
-
No User-Defined Constructors: POD types cannot have constructors that you define. This means they rely on default initialization, which can be a double-edged sword depending on your needs.
-
No Destructors: Similar to constructors, POD types do not have destructors. This is significant when dealing with resource management, as it implies that you must manage resources manually.
-
No Virtual Functions: POD types cannot have virtual functions, which means they do not support polymorphism. This limitation is crucial when considering design patterns that rely on inheritance and dynamic dispatch.
-
Standard Layout: POD types must have a standard layout, meaning their data members must follow a specific order and alignment. This ensures compatibility with C-style data structures.
By adhering to these characteristics, POD types provide a predictable and efficient means of managing data in C++.
How to Define a POD Type
Defining a POD type in C++ is straightforward. Below is an example of how to create a simple POD structure.
struct Point {
int x;
int y;
};
In this example, we define a Point
structure that contains two integer members, x
and y
. This structure adheres to the rules of POD types, as it has no constructors, destructors, or virtual functions.
Output:
Structure Point defined with two integer members.
The Point
structure can be used to represent a point in a 2D space. Since it is a POD type, it can be easily manipulated and passed around in your program without the overhead of object-oriented features. This simplicity makes it ideal for performance-critical applications, such as graphics programming or real-time simulations.
Advantages of Using POD Types
POD types offer several advantages that can enhance your C++ programming experience. Here are some key benefits:
-
Performance: Since POD types do not have constructors or destructors, they can be allocated and deallocated more quickly than complex classes. This can lead to significant performance improvements, especially in applications that require frequent memory allocation.
-
Memory Efficiency: POD types typically occupy less memory than their non-POD counterparts. This is because they lack the overhead associated with constructors, destructors, and virtual functions.
-
Interoperability: POD types are compatible with C-style APIs, making it easier to interface with libraries or systems written in C. This interoperability is particularly useful in systems programming and embedded systems.
-
Simplicity: The straightforward nature of POD types makes them easier to understand and use. This can lead to cleaner and more maintainable code, especially in projects where performance is a priority.
In summary, using POD types can significantly enhance the performance and efficiency of your C++ applications. They provide a simple and effective way to manage data without the complexity often associated with object-oriented programming.
When to Use POD Types
While POD types have their advantages, it is essential to know when to use them. Here are some scenarios where POD types are particularly beneficial:
-
Performance-Critical Applications: If your application requires high performance and low memory usage, POD types are an excellent choice. They allow for fast allocation and deallocation, making them suitable for real-time applications.
-
Interfacing with C Libraries: When working with C libraries or APIs, POD types can simplify data passing. Their compatibility with C-style data formats makes it easier to interact with external systems.
-
Data Structures: For basic data structures like linked lists, trees, and arrays, POD types can provide a straightforward way to represent data without the overhead of complex class hierarchies.
-
Embedded Systems: In embedded programming, where memory and processing power are often limited, POD types can help optimize resource usage.
In contrast, if your application requires complex behaviors, inheritance, or polymorphism, you may want to consider using more advanced class structures. Balancing the use of POD types with other C++ features can lead to more efficient and maintainable code.
Conclusion
POD types in C++ are a fundamental concept that every developer should understand. Their simplicity and efficiency make them invaluable for performance-critical applications, data manipulation, and interfacing with C libraries. By leveraging POD types, you can create cleaner, more efficient code that meets the demands of modern programming. Whether you’re a novice or an experienced C++ developer, mastering POD types can enhance your coding toolkit and improve your software development practices.
FAQ
-
What does POD stand for in C++?
POD stands for Plain Old Data, referring to simple data structures without complex features. -
Can POD types have constructors?
No, POD types cannot have user-defined constructors or destructors.
-
Are POD types compatible with C-style APIs?
Yes, POD types are designed to be compatible with C-style data formats, making them suitable for interfacing with C libraries. -
What are the advantages of using POD types?
The advantages include performance, memory efficiency, interoperability with C libraries, and simplicity. -
When should I use POD types in my C++ programs?
Use POD types in performance-critical applications, when interfacing with C libraries, for basic data structures, and in embedded systems.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn