Division in C++
- Precedence and Associativity Rules in C++
- Integer Division Peculiarity in C++
- Typecast and Solve the Integer Division Peculiarity in C++
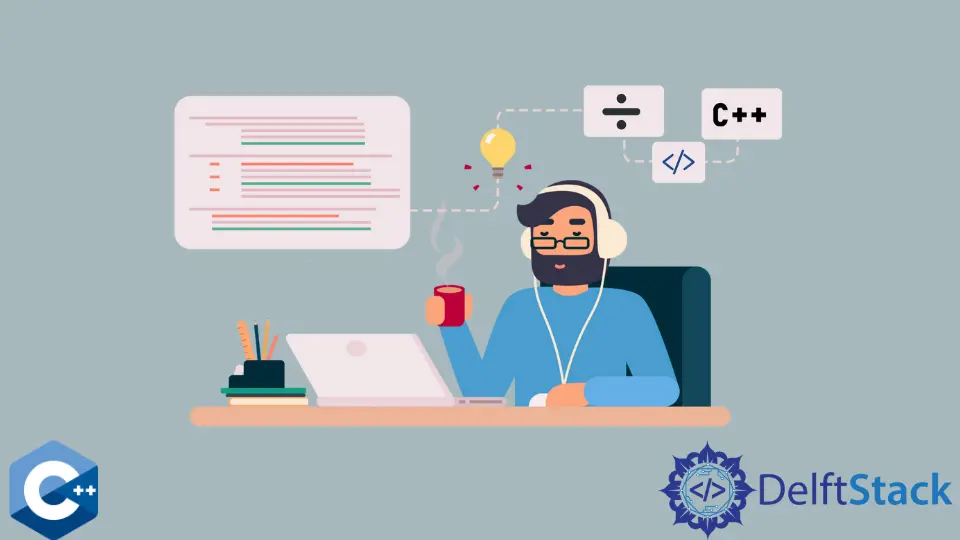
This article is about division rules in C++.
First, we will look into precedence and typecasting rules for division. Later, we will discuss a common integer division peculiarity known as the cut-off cast.
Precedence and Associativity Rules in C++
The term precedence specifies the evaluation order of operators in an expression. For example, some of the precedence rules for C++ operators from the highest level to the lowest level are as follows:
- Expressions written in the parenthesis are evaluated first.
- The operators
*
and\
have the same precedence level. - Similarly, the operators
+
and-
have the same precedence level. - If two operators with the same precedence level occur in an expression, then the operators are evaluated from left to right (except for the assignment operator (
=
). It is also known as operator associativity.
For example, consider the following mixed expression:
(1*2)+3/3*2-1
The above expression is evaluated in the following way:
-
In the first pass, the
*
operator is evaluated first because this is enclosed in parenthesis with the highest precedence level. After the first pass, the expression becomes like this2+3/3*2-1
. -
The
/
operator is first evaluated in the second pass. Although the operators*
and/
have the same precedence level, the operators with equal precedence levels are evaluated from left to right.After the second pass, the expression looks like
2+1*2-1
. -
In the third pass, the multiplication operator is evaluated as it has the highest precedence among the other operators in the expression. After the third pass, the expression would reduce to
2+2-1
. -
The
+
operator is first evaluated in the fourth pass. Although the operators+
and-
have the same precedence level, the operators with equal precedence levels are evaluated from left to right.After the fourth pass, the expression becomes
4-1
. -
Finally,
-
the operator is evaluated, and we get the expression reduced to a single value of3
.
Now, we have sufficient background on operator precedence and associativity. Let’s look into some of the peculiarities of the /
operator in C++.
Integer Division Peculiarity in C++
When we divide two integer numbers in C++, the division operator returns only the integer part of the answer. The fractional part of the answer is cut-off.
The main reason is the implicit typecasting by the compiler. Before jumping into typecasting, let us look at an example of integer division.
#include <iostream>
using namespace std;
int main() {
int m = 10;
int n = 7;
float a = m / n;
cout << "The answer after division is:" << a << endl;
}
Output:
The answer after division is:1
The output confirms that the C++ compiler skips the fractional part of the answer. Let us first understand typecasting to understand the root cause of this peculiarity and possible solutions.
Typecast and Solve the Integer Division Peculiarity in C++
Typecasting means changing the type of a variable. The typecasting can either be implicit or explicit.
Explicit Typecasting
This is performed by the programmers explicitly. The keyword static_cast
is used for explicit typecasting.
This type of casting is done at compile time.
The following code solves the integer division issue by using explicit typecasting. Line 6 of the below code statically casts the values of m
and n
into floating point values; therefore, the result of the division will also be a floating number.
#include <iostream>
using namespace std;
int main() {
int m = 10;
int n = 7;
float a =
static_cast<float>(m) / static_cast<float>(n); // static explicit casting
cout << "The answer after explicit typecasting is: " << a << endl;
}
Output:
The answer after explicit typecasting is: 1.42857
Implicit Typecasting
Implicit typecasting is performed automatically by the compiler. The compiler evaluates the expression according to the data types used in an expression.
The compiler always evaluates the results in a higher data type among all the data types used in the given expression.
Implicit casting is the root cause of the integer division peculiarity. When two integer operands are used with the arithmetic division, the evaluation is implicitly converted to the int data type causing the decimal part of the result to be trimmed off.
We can solve the integer division issue by changing the type of one division operand with some floating point type, as demonstrated in the code below:
#include <iostream>
using namespace std;
int main() {
float a = 10.0 / 7;
cout << "The answer after implicit typecasting is: " << a << endl;
}
Output:
The answer after implicit typecasting is: 1.42857
In the above example, the numerator 10.0
is the float value, and the denominator is an integer value. As the float data type is higher than the integer data type, the compiler implicitly converts the resultant value in the float data type.
There are a few other peculiarities associated with the division operator in C++, such as Division by Zero Error and Division of Pointers which the geeks can explore further.