How to Calculate the Average in C++
- Understanding the Average Calculation
- Method 1: Basic Average Calculation
- Method 2: Using Functions for Average Calculation
- Method 3: Handling User Input for Average Calculation
- Conclusion
- FAQ
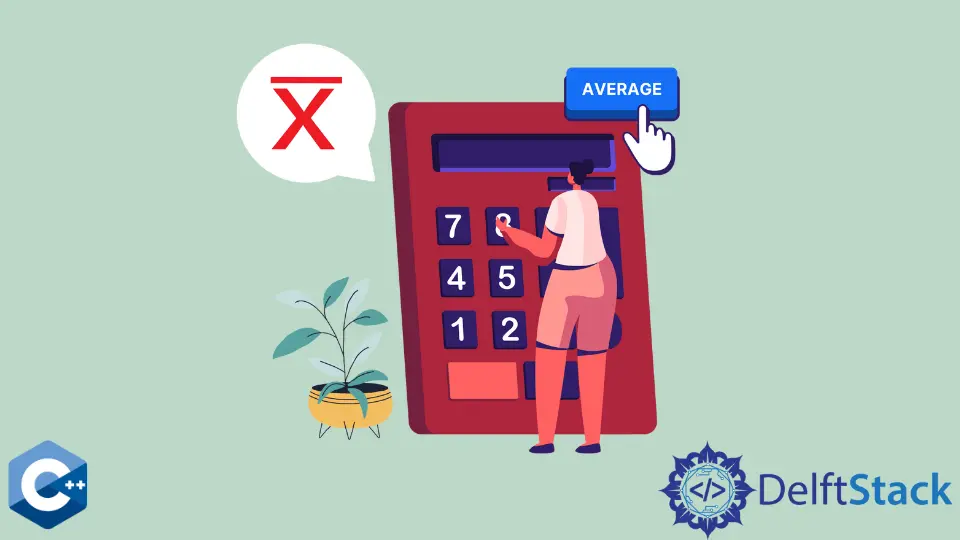
Calculating the average of numbers is a fundamental task in programming. Whether you’re analyzing data or simply trying to understand a set of values, knowing how to effectively compute the average is essential.
In this article, we’ll walk you through the process of calculating the average of numbers stored in an array using C++. This guide will provide you with clear code examples, detailed explanations, and practical tips to ensure you grasp the concept fully. By the end of this article, you’ll be equipped with the knowledge to implement average calculations in your own C++ projects.
Understanding the Average Calculation
Before diving into the code, let’s clarify what we mean by “average.” The average, or mean, is calculated by summing all the numbers in a dataset and then dividing that sum by the total count of numbers. In C++, we can store our numbers in an array, iterate through the array to calculate the sum, and then compute the average. This process not only helps in understanding basic programming concepts but also strengthens your grasp of array manipulations in C++.
Method 1: Basic Average Calculation
The simplest way to calculate the average in C++ involves using a loop to sum the elements of an array and then dividing by the number of elements. Here’s a straightforward example:
#include <iostream>
using namespace std;
int main() {
int numbers[] = {10, 20, 30, 40, 50};
int sum = 0;
int count = sizeof(numbers) / sizeof(numbers[0]);
for (int i = 0; i < count; i++) {
sum += numbers[i];
}
double average = static_cast<double>(sum) / count;
cout << "The average is: " << average << endl;
return 0;
}
Output:
The average is: 30
In this example, we first declare an array of integers named numbers
. We calculate the number of elements in the array using sizeof(numbers) / sizeof(numbers[0])
. This gives us the total count of elements, which is crucial for our average calculation. We then use a for
loop to iterate through the array, adding each element to the sum
variable. Finally, we compute the average by dividing the total sum by the count of elements. The result is displayed using cout
.
Method 2: Using Functions for Average Calculation
To make your code more modular and reusable, you can create a function that calculates the average. This approach is particularly useful when you need to calculate averages for different datasets. Here’s how you can implement this:
#include <iostream>
using namespace std;
double calculateAverage(int arr[], int size) {
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
return static_cast<double>(sum) / size;
}
int main() {
int numbers[] = {15, 25, 35, 45, 55};
int size = sizeof(numbers) / sizeof(numbers[0]);
double average = calculateAverage(numbers, size);
cout << "The average is: " << average << endl;
return 0;
}
Output:
The average is: 35
In this code, we define a function called calculateAverage
that takes an array and its size as parameters. Inside the function, we calculate the sum of the array elements just like before. However, by placing the logic in a function, we can easily call it from main
or any other part of our program. This makes our code cleaner and more organized. The calculated average is then printed out in the main
function.
Method 3: Handling User Input for Average Calculation
In real-world applications, you might want to allow users to input their own numbers. This method enhances interactivity and usability. Here’s how to implement dynamic user input for average calculation:
#include <iostream>
using namespace std;
double calculateAverage(int arr[], int size) {
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
return static_cast<double>(sum) / size;
}
int main() {
int size;
cout << "Enter the number of elements: ";
cin >> size;
int* numbers = new int[size];
cout << "Enter the numbers: ";
for (int i = 0; i < size; i++) {
cin >> numbers[i];
}
double average = calculateAverage(numbers, size);
cout << "The average is: " << average << endl;
delete[] numbers; // Free up memory
return 0;
}
Output:
Enter the number of elements: 5
Enter the numbers: 10 20 30 40 50
The average is: 30
In this example, we first ask the user how many numbers they want to input. We then dynamically allocate an array of that size using new
. The user is prompted to enter their numbers, which are stored in the array. Once the numbers are collected, we call the calculateAverage
function to compute the average. Finally, we free the dynamically allocated memory using delete[]
to prevent memory leaks. This method showcases how to make your program more interactive and user-friendly.
Conclusion
Calculating the average in C++ is a fundamental skill that can be applied in various programming scenarios. Whether you’re using basic loops, creating reusable functions, or allowing user input, the methods outlined in this article provide a solid foundation for averaging numbers in an array. As you practice these techniques, you’ll become more comfortable with C++ programming and array manipulation. Remember, the key to mastering programming is consistent practice and exploration. Happy coding!
FAQ
-
What is an average in programming?
An average, or mean, is a statistical measure that summarizes a set of numbers by dividing the total sum by the count of numbers. -
Can I calculate the average of floating-point numbers in C++?
Yes, you can calculate the average of floating-point numbers using similar methods as shown in the examples, just ensure to usefloat
ordouble
data types. -
How do I handle large datasets for average calculations?
For large datasets, consider using data structures like vectors or lists that can dynamically resize, or implement file handling to read data from files. -
What are some common errors when calculating averages?
Common errors include dividing by zero (if the dataset is empty) and integer overflow when summing large numbers. Always check your inputs before calculations. -
Is it necessary to use functions for average calculations in C++?
While not strictly necessary, using functions improves code readability and reusability, making it easier to maintain and understand.