How to Write to File in C
- Understanding File Operations in C
- Writing Multiple Lines to a File
- Appending Data to an Existing File
- Handling Errors While Writing to Files
- Conclusion
- FAQ
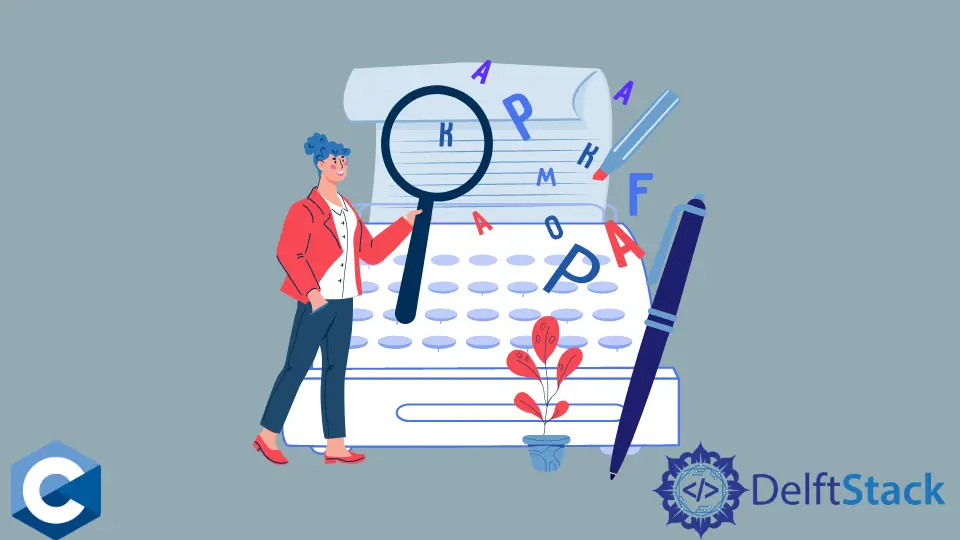
Writing to a file in C is a fundamental skill for anyone looking to deepen their understanding of programming and data management.
This article will guide you through the process of writing to files in C, providing you with practical examples and clear explanations. Whether you’re a beginner or an experienced programmer, mastering file operations is essential for tasks such as data logging, configuration management, and more. By the end of this article, you’ll be equipped with the knowledge to create, write, and manage files effectively in your C applications.
Understanding File Operations in C
In C, file operations are performed using the standard I/O library, which provides functions to handle files. The first step in writing to a file is to open it using the fopen
function. This function takes two parameters: the file name and the mode in which you want to open the file. For writing, you typically use modes like “w” for writing (which creates a new file or truncates an existing one) or “a” for appending (which adds data to the end of an existing file).
Here’s a simple example of how to write to a file in C:
#include <stdio.h>
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
printf("Error opening file!\n");
return 1;
}
fprintf(filePointer, "Hello, World!\n");
fclose(filePointer);
return 0;
}
In this code, we include the standard I/O library and declare a file pointer. We then open a file named “example.txt” in write mode. If the file opens successfully, we write “Hello, World!” to it using the fprintf
function. Finally, we close the file with fclose
to ensure all data is saved and resources are released.
Output:
File created successfully with the message "Hello, World!" written to it.
Opening a file for writing is straightforward, but always remember to check if the file pointer is NULL
. This check is crucial to handle any errors that might occur while opening the file, such as permission issues or non-existent directories.
Writing Multiple Lines to a File
Writing multiple lines to a file in C can be done in a loop or by calling the fprintf
function multiple times. This method is particularly useful when you want to log a series of events or write structured data. Here’s how you can achieve this:
#include <stdio.h>
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
printf("Error opening file!\n");
return 1;
}
for (int i = 1; i <= 5; i++) {
fprintf(filePointer, "This is line %d\n", i);
}
fclose(filePointer);
return 0;
}
In this example, we open the file “example.txt” in write mode and use a loop to write five lines to the file. Each line contains the line number, demonstrating how you can dynamically generate content. After writing, we close the file to save our changes.
Output:
Five lines written to example.txt, numbered from 1 to 5.
Using loops to write data to files is efficient and allows for scalability. You can easily adjust the loop’s range to write more or fewer lines, making this method versatile for various applications.
Appending Data to an Existing File
If you want to add data to an existing file without overwriting its current contents, you can use the append mode (“a”) when opening the file. This is particularly useful for logging applications or when you want to preserve previous entries. Here’s how to append data:
#include <stdio.h>
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "a");
if (filePointer == NULL) {
printf("Error opening file!\n");
return 1;
}
fprintf(filePointer, "Appending a new line.\n");
fclose(filePointer);
return 0;
}
In this code, we open “example.txt” in append mode. This means that any new data we write will be added to the end of the file. We use fprintf
to append a new line of text and close the file afterward.
Output:
New line appended to example.txt successfully.
Appending data is a common requirement in many applications, such as logging user actions or storing results from repeated operations. Using the append mode ensures that you don’t lose any existing data.
Handling Errors While Writing to Files
When working with file operations, it’s essential to handle errors gracefully. The previous examples included basic error checking when opening files, but you can also check for errors during writing. Here’s how you can do this:
#include <stdio.h>
int main() {
FILE *filePointer;
filePointer = fopen("example.txt", "w");
if (filePointer == NULL) {
printf("Error opening file!\n");
return 1;
}
if (fprintf(filePointer, "Writing data...\n") < 0) {
printf("Error writing to file!\n");
fclose(filePointer);
return 1;
}
fclose(filePointer);
return 0;
}
In this example, we check if fprintf
returns a negative value, which indicates a write error. If an error occurs, we print an error message, close the file, and exit the program gracefully.
Output:
Data written successfully or error occurred during writing.
Error handling is a crucial aspect of programming, especially when dealing with file operations. By incorporating error checks, you can ensure that your application behaves predictably and can recover from unexpected situations.
Conclusion
Writing to files in C is a vital skill that enables you to manage data efficiently. From creating files and writing multiple lines to appending data and handling errors, the techniques discussed in this article provide a solid foundation for file operations. As you practice these methods, you’ll find that they can be applied to various programming tasks, enhancing your overall coding capabilities. Remember to always check for errors and handle them appropriately to create robust applications.
FAQ
-
What is the difference between “w” and “a” modes in file operations?
“w” mode overwrites the file if it exists, while “a” mode appends data to the end of the file without deleting existing content. -
How do I check if a file opened successfully in C?
You can check if the file pointer isNULL
after callingfopen
. If it isNULL
, the file failed to open. -
Can I write different data types to a file in C?
Yes, you can use functions likefprintf
to write different data types, formatting them as needed in the output. -
What happens if I forget to close a file in C?
If you forget to close a file, it may lead to data loss or corruption, as changes might not be flushed to disk. -
How can I read data back from a file in C?
You can use thefscanf
orfgets
functions to read data from a file, depending on the format of the data you saved.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook