C로 파일에 쓰기
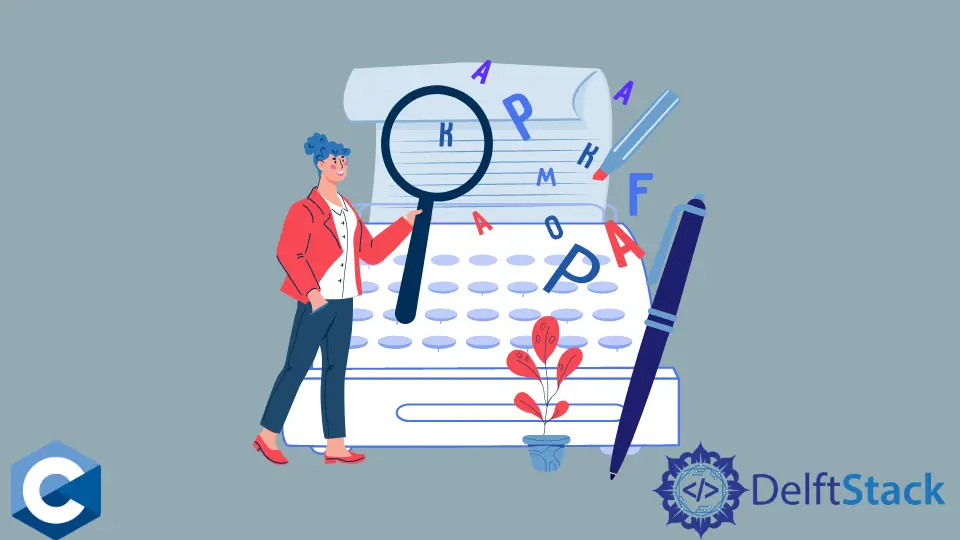
이 기사에서는 C로 파일에 쓰는 방법에 대한 여러 가지 방법을 보여줍니다.
fwrite
함수를 사용하여 C 파일에 쓰기
C의 표준 I/O
라이브러리는 파일 읽기 / 쓰기를위한 핵심 기능인fread
및fwrite
를 제공합니다. fwrite
는 4 개의 인수, 데이터를 가져와야하는 void
포인터, 지정된 포인터의 데이터 요소 크기 및 개수, 출력 파일 스트림에 대한 FILE *
포인터를 취합니다.
파일 스트림은 원하는 모드로 파일 이름을 가져 와서FILE *
을 반환하는fopen
함수로 먼저 열어야합니다. 이 경우w+
모드를 사용하여 읽기 및 쓰기를 위해 파일을 엽니 다. 그러나 파일이 존재하지 않으면 주어진 이름으로 새 파일이 생성됩니다. 다음으로 반환 된 파일 스트림 포인터가 유효한지 확인합니다. 그렇지 않은 경우 해당 오류 메시지를 인쇄하고 프로그램을 종료합니다. 파일 스트림 포인터가 확인되면fwrite
함수를 호출 할 수 있습니다. 프로그램이 종료되기 전에 fclose
함수로 파일을 닫아야합니다.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
const char* str = "Temporary string to be written to file!";
int main(void) {
const char* filename = "out.txt";
FILE* output_file = fopen(filename, "w+");
if (!output_file) {
perror("fopen");
exit(EXIT_FAILURE);
}
fwrite(str, 1, strlen(str), output_file);
printf("Done Writing!\n");
fclose(output_file);
exit(EXIT_SUCCESS);
}
write
함수를 사용하여 C 파일에 쓰기
또는 파일 기술자가 참조하는 파일에 주어진 바이트 수를 쓰는 POSIX 호환 함수 호출 인write
를 사용할 수 있습니다. 파일 설명자는 열린 파일 스트림과 연관된 정수입니다. 디스크립터를 검색하려면 파일 이름 경로와 함께 open
함수를 호출해야합니다. write
함수는 파일 설명자를 첫 번째 인자로,void *
가 가리키는 데이터의 버퍼를 두 번째 인자로 취합니다. 세 번째 인수는 파일에 쓸 바이트 수이며 다음 예제에서strlen
함수로 계산됩니다.
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
const char* str = "Temporary string to be written to file!";
int main(void) {
const char* filename = "out.txt";
int fd = open(filename, O_WRONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
write(fd, str, strlen(str));
printf("Done Writing!\n");
close(fd);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook