Stack Smashing Detected Error in C
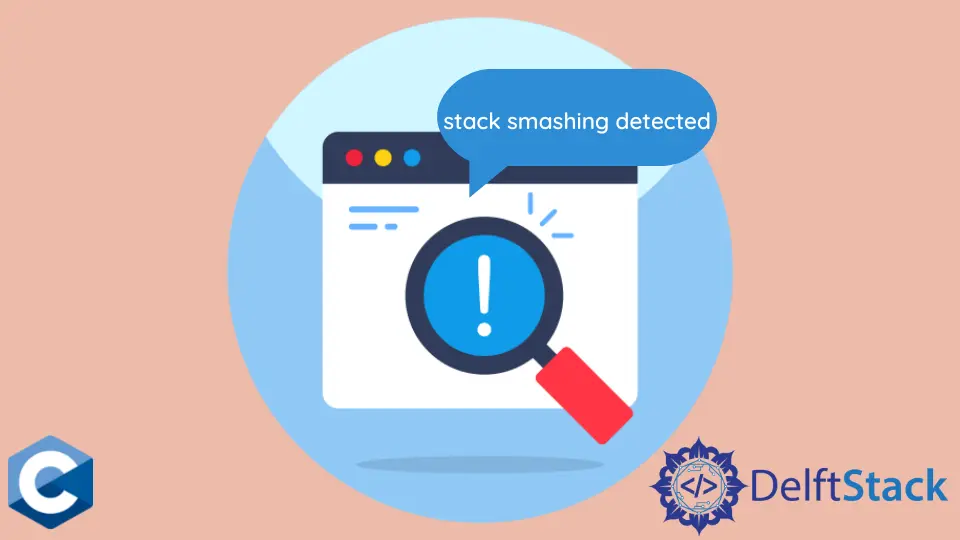
This tutorial highlights the cause and solves the stack smashing detected
error in C.
Anyone facing this issue should understand the division of the memory and stack layout. It will help identify the causes of this error while coding in C programming.
the stack smashing detected
Error in C
Usually, the compiler (we are talking about the GCC compiler here) generates this error to defend against the buffer overflow. The buffer overflow happens whenever the user input exceeds the capacity of the buffer (a temporary storage area).
In that case, the compiler throws an error saying stack smashing detected
. See the following code snippet where we get the stack smashing detected
error if the user inputs more than 10 characters.
Example Code:
#include <stdio.h>
int main(int argc, char **argv) {
char array[10];
printf("Please enter your username.");
gets(array);
return 0;
}
As this error surrounds the buffer overflow and stack concepts, it is crucial to understand the division of the memory and stack layout here.
The buffer overflow is a dangerous process, so the compiler gets the advantage of different protection mechanisms to guard against this error. One of the mechanisms is known as a canary, a randomly generated value.
The compiler (GCC) adds the protection variables (called canaries) that have known values. The canary is overwritten if the buffer overflows.
Further, the compiler identifies by comparing with known values that the stack is compromised and generates an error saying: stack smashing detected
.
To prevent the buffer overflow protection variable and have some insights, we can disable the GCC’s protection using the -fno-stack-protector
while compiling. For instance, $ gcc -o filename -fno-stack-protector
.
In this way, we most likely will get the segmentation fault
error because we will be trying to access the illegal memory location. Remember that the fstack-protector
must be turned on for the release builds because it’s a security feature.
Fix the stack smashing detected
Error in C
Now, how do we rectify this error and execute the program smoothly?
We can not say that preventing the stack protector is a solution to this problem, but it helps find some insights that we can use to rectify this error. We can also get some details about the cause of overflow by executing the program with a debugger.
The Valgrind will not work well with the stack-related issues and errors, but a debugger may help us pinpoint the reason and location for the crash. Find that buggy code and fix it to avoid severe security vulnerabilities.