A Label Can Only Be Part of a Statement and a Declaration Is Not a Statement
- a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error in C
-
a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error When Using
switch
Statements in C - Ways to Avoid the a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error in C
- Conclusion
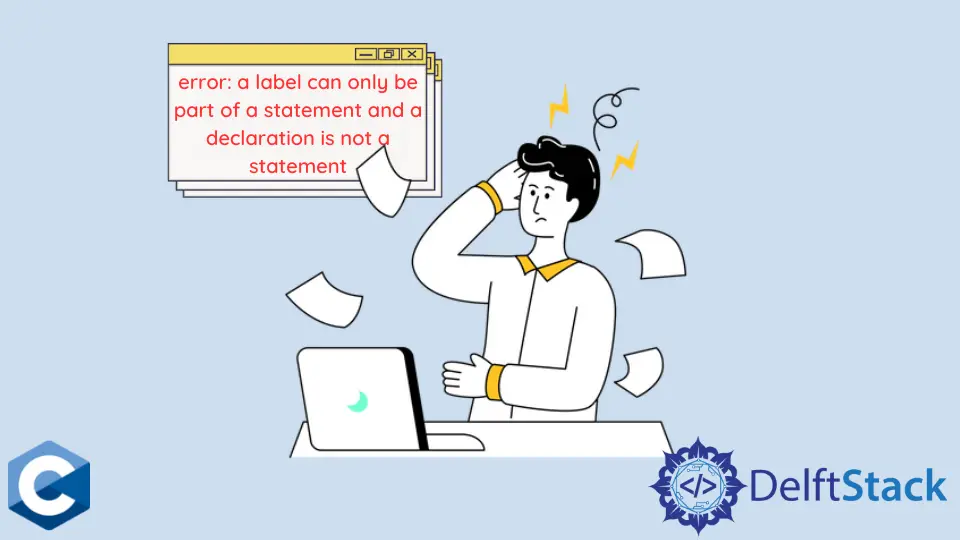
While programming in any programming language, we encounter errors that result in the abnormal working of our program or halt our program until the error is resolved.
This article will discuss an error known as a label can only be part of a statement and a declaration is not a statement
that occurs only in the C language.
a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error in C
Errors are just some unexpected situations or illegal actions made by the programmer that results in the program’s abnormal working. These errors are of various types based on their effects.
However, some errors are not common in all programming languages but occur only in a particular language. One such error is a label can only be part of a statement and a declaration is not a statement
that occurs only in the C language.
This error occurs when a declaration immediately follows a label. However, the C language standards only allow statements to follow a label, not a declaration.
A label in C language is a sequence of characters that refer to a particular location in the source code. A label is used with the goto
statement in a program.
It would be used something like the below code:
#include <stdio.h>
int main() {
goto TARGET;
TARGET:
printf("Hello World");
return 0;
}
Output:
Hello World
Whenever the goto
statement is reached, the program control jumps to the specified label in the program. As you can see in the above program, the output is successfully printed because the label TARGET
has been followed by a printf
statement.
However, if we try to declare a variable after the label, the same code would give us an error because the C language standards do not allow a declaration immediately after a label. Therefore, the program would throw the a label can only be part of a statement and a declaration is not a statement
error.
Let us see an example that throws such an error:
#include <stdio.h>
int main() {
goto TARGET;
TARGET:
char* s = "Hello World";
printf("%s", s);
return 0;
}
Output:
.ex1.c: In function 'main':
.ex1.c:7:5: error: a label can only be part of a statement and a declaration is not a statement
char * s = "Hello World";
^
As shown in the output, the error a label can only be part of a statement and a declaration is not a statement
is mentioned. Moreover, the specified problem line has also been shown, which is the declaration statement after the label TARGET
.
However, had the declaration not immediately followed the label, the program would have worked fine. Let us see an example of that as well.
#include <stdio.h>
int main() {
goto TARGET;
TARGET:
printf("%d", 2 + 5);
char* s = "Hello World";
printf("%s", s);
return 0;
}
Output:
7Hello World
Therefore, the error a label can only be part of a statement and a declaration is not a statement
only occurs when a declaration immediately follows a label in a program. No error was thrown when we added a printf
statement after the label and the declaration.
a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error When Using switch
Statements in C
The error that a label can only be part of a statement and a declaration is not a statement
also occurs while using the switch
statement in C. This is because the C language treats the cases similar to labels.
Therefore, whenever we try to declare anything just after a case, the error a label can only be part of a statement and a declaration is not a statement
is thrown to the user.
Let us see an example of the same:
#include <stdio.h>
int main() {
char ch = 'A';
switch (ch) {
case 'A':
char* s = "Case A";
printf("%s", s);
break;
}
}
Output:
.ex1.c: In function 'main':
.ex1.c:8:7: error: a label can only be part of a statement and a declaration is not a statement
char * s = "Case A";
^
Therefore, as you can see in the above output, the same error has been thrown when using the switch statements in C when we try declaring the variable s
immediately after case A
.
Ways to Avoid the a Label Can Only Be Part of a Statement and a Declaration Is Not a Statement Error in C
Now, we will discuss how this error a label can only be part of a statement and a declaration is not a statement
can be resolved.
We can avoid this error by simply putting a semi-colon after the label. The compiler will treat the semi-colon as a blank statement and will not throw an error.
Let us see the above solution with the help of an example.
#include <stdio.h>
int main() {
goto TARGET;
TARGET:;
char* s = "Hello World";
printf("%s", s);
return 0;
}
Output:
Hello World
Now, you can see in the above code that by adding a semi-colon after the label TARGET
, we can declare the variable s
immediately after the label. It would not throw the error a label can only be part of a statement and a declaration is not a statement
.
As discussed earlier, the same error also occurs while dealing with the switch
statements because the cases
are considered similar to labels in the C language.
Therefore, to resolve the error in the switch
statements, we can either add a semi-colon after a case
or put curly braces around the block of a case
in a switch
statement. Either of the ways would resolve our error.
Let us look at the code with a semi-colon after a case
:
#include <stdio.h>
int main() {
char ch = 'A';
switch (ch) {
case 'A':;
char* s = "Case A";
printf("%s", s);
break;
}
}
​Output:
Case A
Therefore, as you can see, just by adding a semi-colon, the error a label can only be part of a statement and a declaration is not a statement
is resolved.
Now, let us resolve the error by adding a pair of curly braces around the block of the label.
#include <stdio.h>
int main() {
char ch = 'A';
switch (ch) {
case 'A': {
char* s = "Case A";
printf("%s", s);
break;
}
}
}
Output:
Case A
We have resolved the error by adding a pair of curly braces around the statements of the case A
.
Conclusion
In this article, we have discussed the error a label can only be part of a statement and a declaration is not a statement
in detail. The C language standards only allow statements after a label and do not permit declarations immediately after a label which causes the error to be thrown.
To resolve the error, we could use a semi-colon after the label and a pair of curly braces around the block of the label in the case
of switch
statements in C.