How to Get Thread ID in C
-
Use the
pthread_self
Function to Get Thread ID in C -
Use the
gettid
Function to Get Thread ID in C -
Use the
thrd_current
Function to Get Thread ID in C
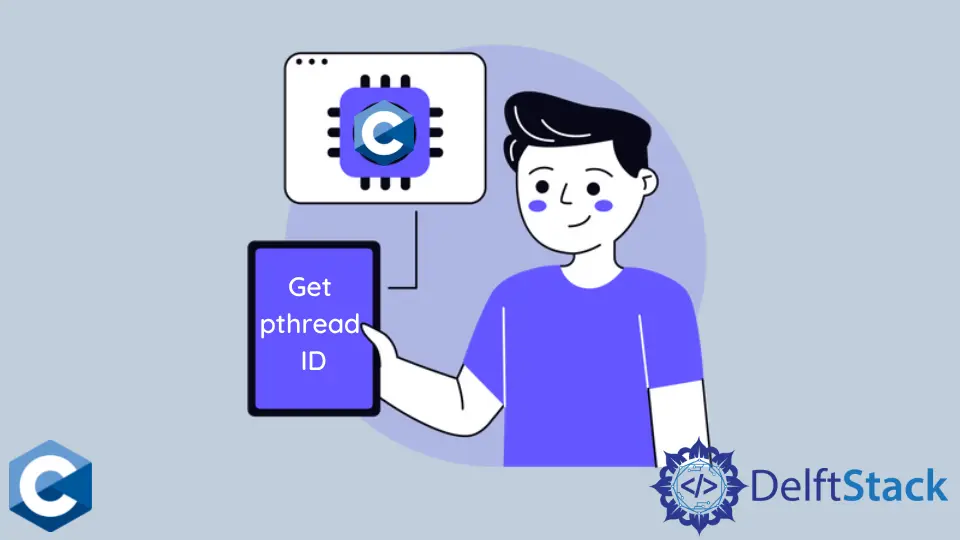
This article will demonstrate multiple methods about how to get thread ID in C.
Use the pthread_self
Function to Get Thread ID in C
Threads are the cornerstone of performance on contemporary CPUs as the latter tend to add more virtual or physical cores nowadays to support better multi-threaded workflows. Generally, a thread is denoted as a single flow of control in a process (i.e., running program). Thus, threads are used to implement multiple logic flows that would execute concurrently and form a program that utilizes multiple CPU cores. Note that POSIX threads have been the oldest standard interface for accessing threading facilities in C programs. pthread_self
is one of the functions provided by the pthreads
API that can retrieve the ID of the calling thread. It takes zero arguments and returns the integer denoting the thread ID as the pthread_t
type variable.
In the following example code, we implemented the basic multi-threaded scenario, where the main program(thread) creates additional four threads that execute the printHello
function and terminate using the pthread_exit
function call. Notice that five threads are running during this program, and all exit after the given code path is executed. In the printHello
function, all of them print their thread ID using the pthread_self
function.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, pthread_self());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Output:
Hello There! thread 1, pthread ID - 140388002486016
Hello There! thread 0, pthread ID - 140388010878720
Hello There! thread 2, pthread ID - 140387994093312
Hello There! thread 3, pthread ID - 140387985700608
Use the gettid
Function to Get Thread ID in C
gettid
is a Linux-specific system call that is provided using the function wrapper in the C program, and it returns the caller’s thread ID. The function takes no arguments similar to the pthread_self
and returns the pid_t
type integer value. Note that the value returned by the gettid
call is not the same as the ID retrieved from the pthread_self
function, which is called POSIX thread ID. If the program is single-threaded, gettid
returns the same value as the getpid
- equal to the process ID.
#define _GNU_SOURCE
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, kthread ID - %d\n", tid, gettid());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Output:
Hello There! thread 0, kthread ID - 10389
Hello There! thread 1, kthread ID - 10390
Hello There! thread 2, kthread ID - 10391
Hello There! thread 3, kthread ID - 10392
Use the thrd_current
Function to Get Thread ID in C
thrd_current
is part of ISO C threads API added to the standard language specification in 2011. Note that this API provides a standard interface for POSIX compatible operating systems and any platform that provides a standard-compliant C compiler. Thus, this method is recommended way of interacting with threads in the C language. We use the pthread
functions for creating and exiting the threads in the following example, though, one should choose a single interface (Pthreads or ISO C) when working on a project.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <threads.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, thrd_current());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Output:
Hello There! thread 0, pthread ID - 139727514998528
Hello There! thread 1, pthread ID - 139727506605824
Hello There! thread 2, pthread ID - 139727498213120
Hello There! thread 3, pthread ID - 139727489820416
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook