Ottieni l'ID thread in C
-
Usa la funzione
pthread_self
per ottenere l’ID del thread in C -
Usa la funzione
gettid
per ottenere l’ID del thread in C -
Usa la funzione
thrd_current
per ottenere l’ID del thread in C
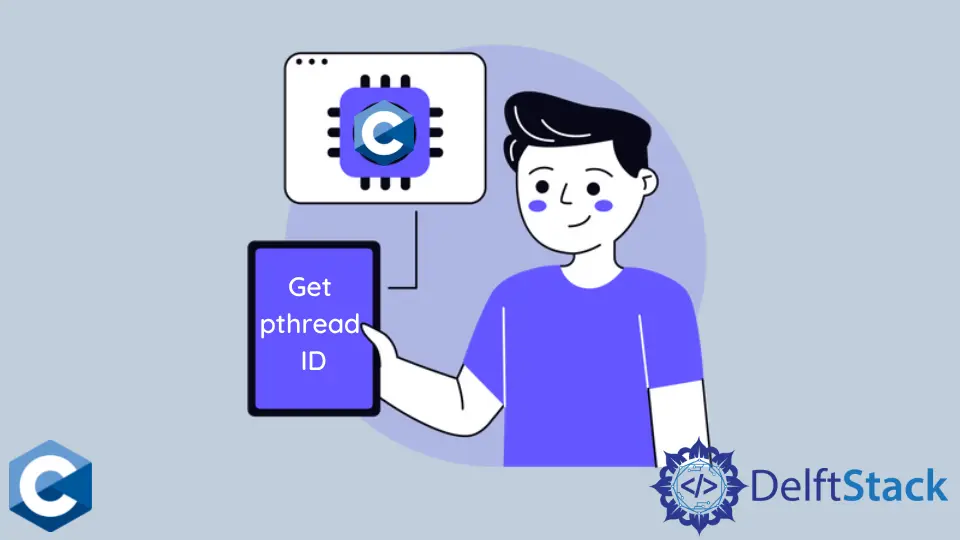
Questo articolo illustrerà più metodi su come ottenere l’ID thread in C.
Usa la funzione pthread_self
per ottenere l’ID del thread in C
I thread sono la pietra angolare delle prestazioni sulle CPU contemporanee poiché queste ultime tendono ad aggiungere più core virtuali o fisici al giorno d’oggi per supportare migliori flussi di lavoro multi-thread. Generalmente, un thread è indicato come un singolo flusso di controllo in un processo (cioè, programma in esecuzione). Pertanto, i thread vengono utilizzati per implementare più flussi logici che potrebbero essere eseguiti contemporaneamente e formare un programma che utilizza più core della CPU. Notare che i thread POSIX sono stati la più vecchia interfaccia standard per l’accesso alle funzioni di threading nei programmi C. pthread_self
è una delle funzioni fornite dall’API pthreads
che può recuperare l’ID del thread chiamante. Non richiede argomenti e restituisce il numero intero che denota l’ID del thread come variabile di tipo pthread_t
.
Nel seguente codice di esempio, abbiamo implementato lo scenario multi-thread di base, in cui il programma principale (thread) crea quattro thread aggiuntivi che eseguono la funzione printHello
e terminano utilizzando la chiamata alla funzione pthread_exit
. Notare che cinque thread sono in esecuzione durante questo programma e tutti terminano dopo che il percorso di codice specificato è stato eseguito. Nella funzione printHello
, tutti stampano il loro ID thread usando la funzione pthread_self
.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, pthread_self());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Produzione:
Hello There! thread 1, pthread ID - 140388002486016
Hello There! thread 0, pthread ID - 140388010878720
Hello There! thread 2, pthread ID - 140387994093312
Hello There! thread 3, pthread ID - 140387985700608
Usa la funzione gettid
per ottenere l’ID del thread in C
gettid
è una chiamata di sistema specifica di Linux fornita utilizzando il wrapper di funzione nel programma C e restituisce l’ID del thread del chiamante. La funzione non accetta argomenti simili a pthread_self
e restituisce il valore intero di tipo pid_t
. Notare che il valore restituito dalla chiamata gettid
non è lo stesso dell’ID recuperato dalla funzione pthread_self
, che è chiamata ID thread POSIX. Se il programma è a thread singolo, gettid
restituisce lo stesso valore di getpid
, uguale all’ID del processo.
#define _GNU_SOURCE
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, kthread ID - %d\n", tid, gettid());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Produzione:
Hello There! thread 0, kthread ID - 10389
Hello There! thread 1, kthread ID - 10390
Hello There! thread 2, kthread ID - 10391
Hello There! thread 3, kthread ID - 10392
Usa la funzione thrd_current
per ottenere l’ID del thread in C
thrd_current
fa parte dell’API dei thread ISO C aggiunta alla specifica del linguaggio standard nel 2011. Notare che questa API fornisce un’interfaccia standard per i sistemi operativi compatibili con POSIX e qualsiasi piattaforma che fornisce un compilatore C conforme allo standard. Pertanto, questo metodo è il modo consigliato per interagire con i thread nel linguaggio C. Usiamo le funzioni pthread
per creare ed uscire dai thread nell’esempio seguente, tuttavia, si dovrebbe scegliere una singola interfaccia (Pthreads o ISO C) quando si lavora su un progetto.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <threads.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, thrd_current());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Produzione:
Hello There! thread 0, pthread ID - 139727514998528
Hello There! thread 1, pthread ID - 139727506605824
Hello There! thread 2, pthread ID - 139727498213120
Hello There! thread 3, pthread ID - 139727489820416
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook