Obtenha o ID do thread em C
-
Use a função
pthread_self
para obter o ID do thread em C -
Use a função
gettid
para obter o ID do thread em C -
Use a função
thrd_current
para obter o ID do thread em C
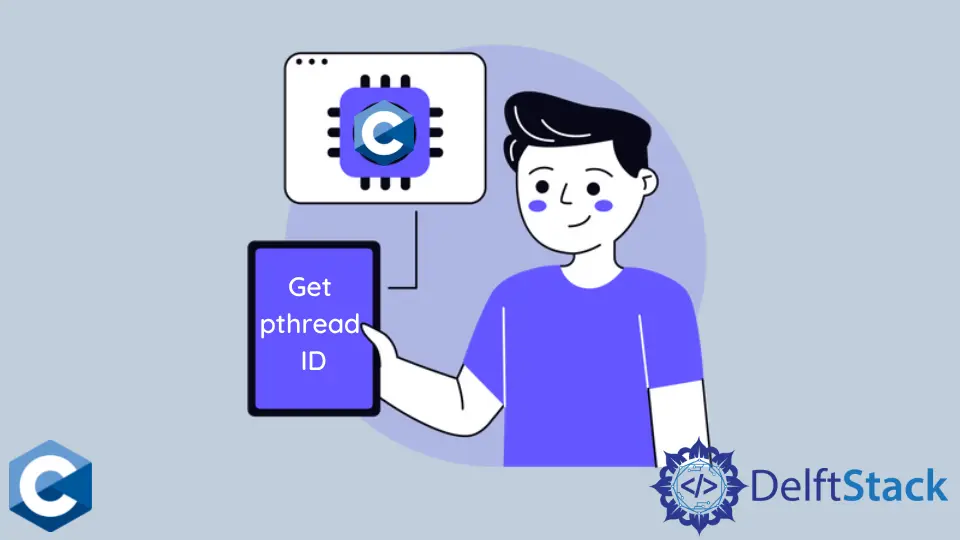
Este artigo demonstrará vários métodos sobre como obter a ID do thread em C.
Use a função pthread_self
para obter o ID do thread em C
Threads são a base do desempenho em CPUs contemporâneas, já que as últimas tendem a adicionar mais núcleos virtuais ou físicos hoje em dia para suportar melhores fluxos de trabalho multi-threaded. Geralmente, um thread é denotado como um único fluxo de controle em um processo (ou seja, programa em execução). Assim, os threads são usados para implementar vários fluxos lógicos que seriam executados simultaneamente e formariam um programa que utiliza vários núcleos de CPU. Observe que os threads POSIX têm sido a interface padrão mais antiga para acessar recursos de threading em programas C. pthread_self
é uma das funções fornecidas pela API pthreads
que pode recuperar o ID do thread de chamada. Leva zero argumentos e retorna o inteiro denotando o ID do thread como a variável do tipo pthread_t
.
No código de exemplo a seguir, implementamos o cenário multi-threaded básico, onde o programa principal (thread) cria quatro threads adicionais que executam a função printHello
e terminam usando a chamada de função pthread_exit
. Observe que cinco threads estão em execução durante este programa e todas saem depois que o caminho de código fornecido é executado. Na função printHello
, todos eles imprimem seu ID de thread usando a função pthread_self
.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, pthread_self());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Resultado:
Hello There! thread 1, pthread ID - 140388002486016
Hello There! thread 0, pthread ID - 140388010878720
Hello There! thread 2, pthread ID - 140387994093312
Hello There! thread 3, pthread ID - 140387985700608
Use a função gettid
para obter o ID do thread em C
gettid
é uma chamada de sistema específica do Linux que é fornecida usando o wrapper de função no programa C e retorna o ID de thread do chamador. A função não recebe argumentos semelhantes a pthread_self
e retorna o valor inteiro do tipo pid_t
. Observe que o valor retornado pela chamada gettid
não é o mesmo que o ID recuperado da função pthread_self
, que é chamada de ID de thread POSIX. Se o programa for de thread único, gettid
retorna o mesmo valor que getpid
- igual ao ID do processo.
#define _GNU_SOURCE
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, kthread ID - %d\n", tid, gettid());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Resultado:
Hello There! thread 0, kthread ID - 10389
Hello There! thread 1, kthread ID - 10390
Hello There! thread 2, kthread ID - 10391
Hello There! thread 3, kthread ID - 10392
Use a função thrd_current
para obter o ID do thread em C
thrd_current
é parte da API ISO C threads adicionada à especificação da linguagem padrão em 2011. Observe que esta API fornece uma interface padrão para sistemas operacionais compatíveis com POSIX e qualquer plataforma que forneça um compilador C compatível com o padrão. Assim, este método é uma forma recomendada de interagir com threads na linguagem C. Usamos as funções pthread
para criar e sair das threads no exemplo a seguir, entretanto, deve-se escolher uma única interface (Pthreads ou ISO C) ao trabalhar em um projeto.
#include <pthread.h>
#include <stdio.h>
#include <stdlib.h>
#include <threads.h>
#include <unistd.h>
#ifndef NUM_THREADS
#define NUM_THREADS 4
#endif
void *printHello(void *threadid) {
long tid;
tid = (long)threadid;
printf("Hello There! thread %ld, pthread ID - %lu\n", tid, thrd_current());
pthread_exit(NULL);
}
int main(int argc, char const *argv[]) {
pthread_t threads[NUM_THREADS];
int rc;
long t;
for (t = 0; t < NUM_THREADS; t++) {
rc = pthread_create(&threads[t], NULL, printHello, (void *)t);
if (rc) {
printf("ERORR; return code from pthread_create() is %d\n", rc);
exit(EXIT_FAILURE);
}
}
pthread_exit(NULL);
}
Resultado:
Hello There! thread 0, pthread ID - 139727514998528
Hello There! thread 1, pthread ID - 139727506605824
Hello There! thread 2, pthread ID - 139727498213120
Hello There! thread 3, pthread ID - 139727489820416
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook