Logical XOR Operator in C
-
an Overview of XOR (
^
) Operator in C -
Use
^
to Apply XOR in C Programming Language - Apply XOR in C Programming Language
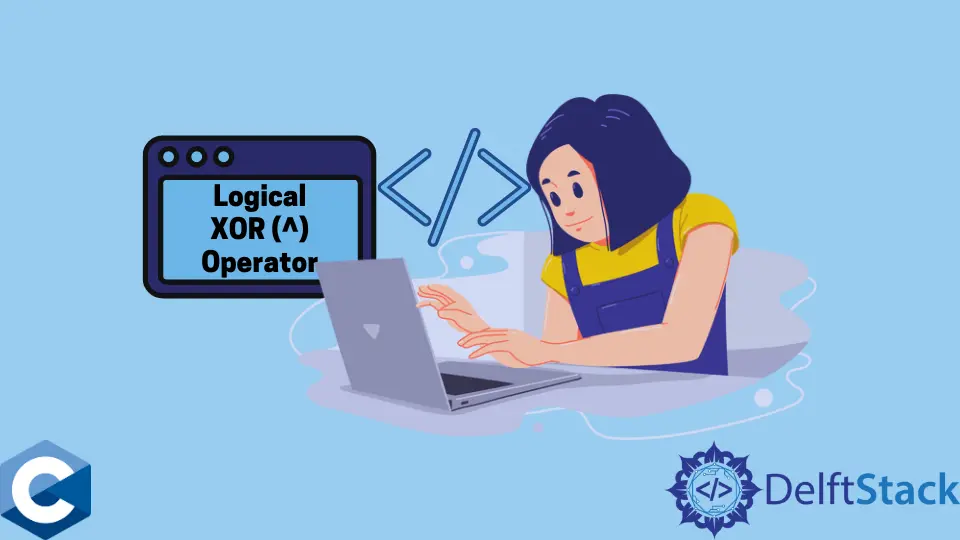
In this article, we will understand about logical XOR (^
) operator in the C programming language.
an Overview of XOR (^
) Operator in C
Exclusive OR
, also known as XOR, is a logical operator that returns a true
result when either of the operands is true (one is true, and the other is false), but not when both are true nor when both are false. When both operands are true, using the basic or
in logical condition creation might lead to confusion.
Because under such circumstances, it is challenging to comprehend what it is that precisely meets the criteria. This issue has been resolved by adding the qualifier exclusive
to the word or
, which also clarifies its meaning.
Use ^
to Apply XOR in C Programming Language
The ^
operator, also known as bitwise XOR, takes two integers as its operands and performs XOR on every bit of both numbers.
If both bits are different, the XOR operation will provide a value of 1
. If the bits are identical, it will return the value 0.
Let us take an example to apply the bitwise XOR in C. Firstly, import the libraries and create a main()
function.
#include <stdio.h>
int main() {}
Inside the main function, establish two variables named firstValue
and secondValue
with the int datatype and set integer values for each. The firstValue
is equal to 0101,
and the secondValue
is equal to 1001
in binary format.
int firstValue = 5;
int secondValue = 9;
Now that we have these two variables, we need to apply XOR to them, so let us create a new variable called applyXOR
and use the symbol ^
on both of these variables.
int applyXOR = firstValue ^ secondValue;
In the above expression, the XOR operation will be performed on both binary values shown as follows.
firstValue |
secondValue |
firstValue ^ secondValue |
---|---|---|
0 | 1 | 1 |
1 | 0 | 1 |
0 | 0 | 0 |
1 | 1 | 0 |
Now we get the number 1100
in binary format, which, converted to decimal, is the same as the value 12
. Then we print the value that was obtained.
printf("The value obtained after applying XOR is: %d", applyXOR);
Apply XOR in C Programming Language
Code Example:
#include <stdio.h>
int main() {
int firstValue = 5;
int secondValue = 9;
int applyXOR = firstValue ^ secondValue;
printf("The value obtained after applying XOR is: %d", applyXOR);
return 0;
}
Output:
The value obtained after applying XOR is: 12
Let’s take another example to demonstrate the working of the XOR (^
) operator.
Given a collection of numbers in which every item occurs an even number of times apart from one number, locate the odd occurring number. Simply applying the XOR(^
) operator to each integer to solve this issue is an effective way to tackle it.
Code Example:
#include <stdio.h>
int getOddOccurringNumber(int arr[], int number) {
int value = 0, i;
for (i = 0; i < number; i++) value ^= arr[i];
return value;
}
int main(void) {
int array[] = {12, 12, 14, 90, 14, 16, 16};
int sizeNumber = sizeof(array) / sizeof(array[0]);
printf("The value that occurred odd times in the list is: %d ",
getOddOccurringNumber(array, sizeNumber));
return 0;
}
Output:
The value that occurred odd times in the list is: 90
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn