How to Generate Random Number in C
-
Use the
rand
andsrand
Functions to Generate Random Number in C -
Use the
random
andsrandom
Functions to Generate Random Number in C -
Use the
getrandom
Function to Generate Random Number in C
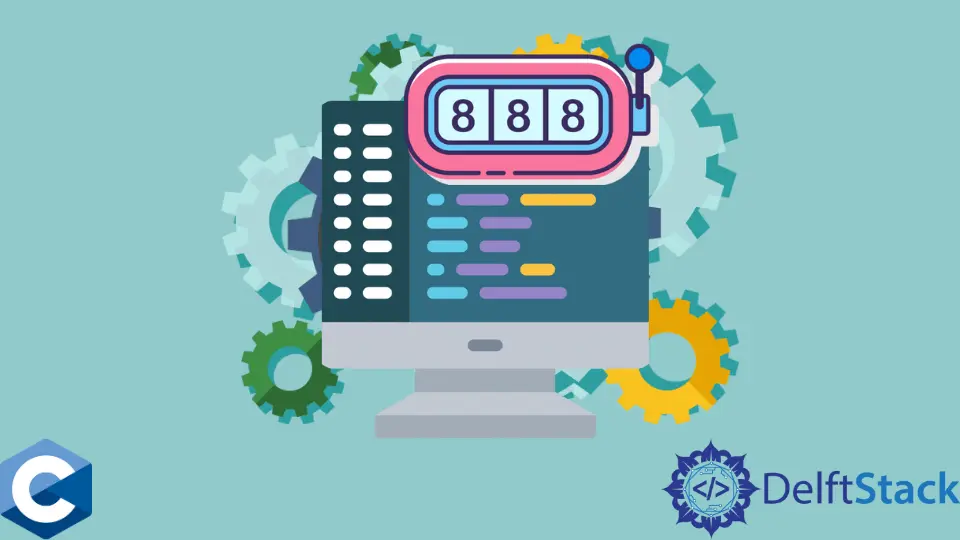
This article will introduce several methods of how to generate random numbers in C.
Use the rand
and srand
Functions to Generate Random Number in C
The rand
function implements a pseudo-random number generator that can provide an integer in the range of [0, RAND_MAX]
, where RAND_MAX
is 231-1 on modern systems. Note that the generator algorithm behind the rand
function is deterministic. Thus it should be seeded with random bits.
The srand
function is used to seed the pseudo-random number generator, and subsequent calls to rand
will produce random integer sequences. On the downside, rand
implementations are not expected to produce uniformly random bits.
Thus, the rand
function is not recommended to be utilized in cryptographically highly sensitive applications. The following example seeds the generator with the value of current time, which is not a good source of randomness.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
srand(time(NULL));
for (int i = 0; i < NUMS_TO_GENERATE; i++) {
printf("%d\n", rand() % MAX);
}
exit(EXIT_SUCCESS);
}
Output:
85084
91989
85251
85016
43001
54883
8122
84491
6195
54793
Use the random
and srandom
Functions to Generate Random Number in C
Another pseudo-random pseudo-random number generator available in the C standard library is implemented under the random
function. This method is the preferred method compared to the rand
, but cryptographic applications should not utilize the random
function in sensitive code.
random
takes no arguments and returns long int
type integer in the range of [0, RAND_MAX]
. The function should preferably be seeded with the srandom
function to generate relatively good quality random numbers.
Note that, like the previous example, we use the time
function to pass the current time value as seed, which is not recommended in security-sensitive applications.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/random.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
srandom(time(NULL));
for (int i = 0; i < NUMS_TO_GENERATE; i++) {
printf("%ld\n", random() / MAX);
}
printf("\n");
exit(EXIT_SUCCESS);
}
Output:
91
2019
2410
11784
9139
5858
5293
17558
16625
3069
Use the getrandom
Function to Generate Random Number in C
getrandom
is a Linux specific function to obtain random bits that are of far better quality than two previous methods provided. getrandom
takes three arguments - void
pointer that points to the buffer where random bits should be stored, the size of the buffer in bytes, and flags for special features.
In the following example, we generate a single unsigned
integer, the address of which &tmp
is passed as the buffer to store random bits, and the size is calculated with the sizeof
operator.
The source of randomness from where the getrandom
retrieves the bits can be uninitialized in rare scenarios. The call to the getrandom
function will block the program execution. Thus, the GRND_NONBLOCK
macro definition is passed as the third argument for the function to return the error value -1 immediately in these cases.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/random.h>
#include <time.h>
#define MAX 100000
#define SIZE 100
#define NUMS_TO_GENERATE 10
int main() {
unsigned int tmp;
getrandom(&tmp, sizeof(unsigned int), GRND_NONBLOCK) == -1
? perror("getrandom")
: "";
printf("%u\n", tmp);
exit(EXIT_SUCCESS);
}
934103271
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook