Ng-Repeat Filter in Angular
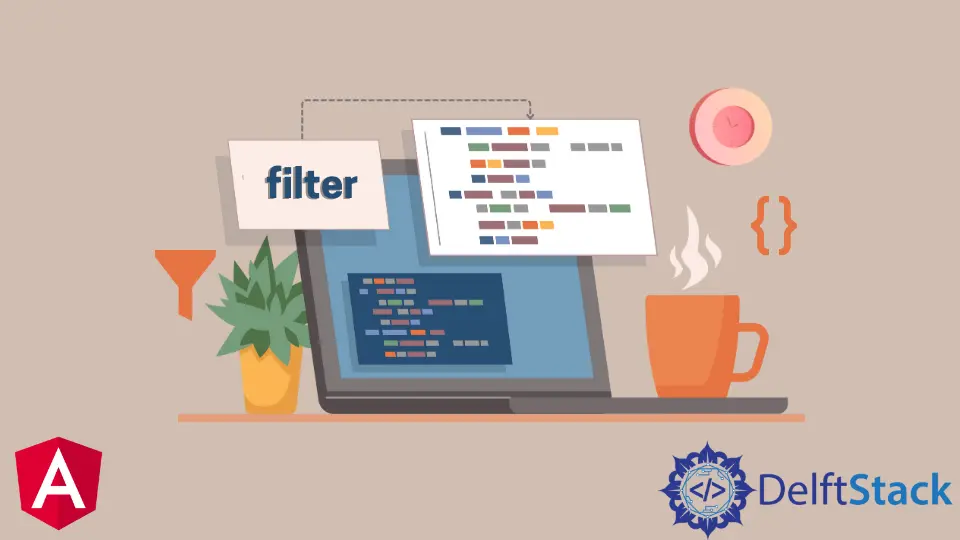
We will introduce how to use the filter
property in ng-repeat
in Angular.
Filter in Angular Ng-Repeat
Ng-repeat
is used to repeat a set of HTML until the items in the list are displayed. It is handy to display a list of data with the same design on a page, like in tables or blog posts.
While displaying a list of data in tables or blog posts, sometimes we want to filter specific data from the displayed result. To display data according to the category or any other specific tag, we can use the filter
in ng-repeat
, which will display data based on the query we want.
Let’s go through an example to understand filter
in ng-repeat
. First, we will create a list of books displayed using ng-repeat
.
Let’s create a list of four books that will be displayed.
# angular
var app = angular.module("myList", []);
app.controller("ListCtrl", function($scope) {
$scope.records = [
{
"Name" : "Book 1",
"Publisher" : "Germany"
},
{
"Name" : "Book 2",
"Publisher" : "Czeck"
},
{
"Name" : "Book 3",
"Publisher" : "Canada"
},
{
"Name" : "Book 4",
"Publisher" : "Australia"
}
]
});
Now that we have created a list, we will create a template to display our list using ng-repeat
. We will use Bootstrap
to create a table with this list of books.
# angular
<body ng-app="myList">
<table ng-controller="ListCtrl" border="1">
<tr ng-repeat="book in records">
<td>{{book.Name}}</td>
<td>{{book.Publisher}}</td>
</tr>
</table>
</body>
Output:
In the image, you can see we have displayed the books list into a table using ng-repeat
.
Now let’s filter our list using filter
in ng-repeat
.
# angular
<body ng-app="myList">
<table ng-controller="ListCtrl" border="1">
<tr ng-repeat="book in records | filter: {Name: 'Book 1'} ">
<td>{{book.Name}}</td>
<td>{{book.Publisher}}</td>
</tr>
</table>
</body>
Let’s check the output.
In the image, you can see that we can easily use the filter property in ng-repeat
to filter out the results as we like.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn