getElementById Replacement in Angular Using TypeScript
-
the
document.getElementById()
Method in TypeScript -
Use
ElementRef
asgetElementById
Replacement in Angular Using TypeScript
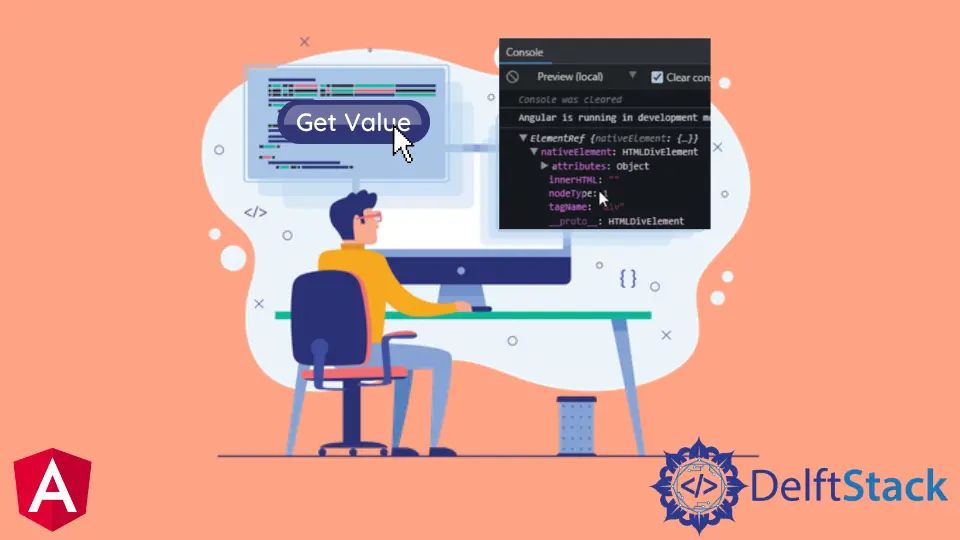
This tutorial guideline provides a brief explanation of the document.getElementById
replacement in Angular using TypeScript.
This also provides the best methods used to getElementById
in Angular with code examples. We’ll also learn the purpose of the DOM querySelector in TypeScript.
First, let us learn the document.getElementById
method in TypeScript and why it is popular among the developer community.
the document.getElementById()
Method in TypeScript
The document.getElementById()
is a predefined JavaScript (as well as TypeScript) method available for the manipulation of the document
object. It returns an element with a specified value and null
if the element does not exist.
The document.getElementById()
is one of the most common and popular method among developer community for the HTML DOM.
The one vital point to keep in mind is that if two or more elements have the same id, document.getElementById()
will return the first element.
Now, let’s look at some of the coding examples to better understand their purpose and usage.
Consider the h1
tag with some text with a unique demo
id. Now, in the scripts
section, if we want to target that particular tag, we use the getElementById()
method available in the document
object.
<!DOCTYPE html>
<html>
<body>
<h1 id="demo">The Document Object</h1>
<h2>The getElementById() Method</h2>
<script>
document.getElementById("demo").style.color = "red";
</script>
</body>
</html>
Output:
Now let’s consider one more dynamic example: an input field takes a number and returns its cube value.
<!DOCTYPE html>
<html>
<body>
<form>
Enter No:<input type="text" id="number" name="number"/><br/>
<input type="button" value="cube" onclick="getcube()"/>
</form>
<script>
function getcube(){
var number=document.getElementById("number").value;
alert(number*number*number);
}
</script>
</body>
</html>
Output:
Now, let us look at the getElementById
replacement in Angular by using TypeScript.
Use ElementRef
as getElementById
Replacement in Angular Using TypeScript
The ElementRef
of AngularJs is a wrapper around an HTML element, containing the property nativeElement
and holding the reference to the underlying DOM object. Using ElementRef
, we can manipulate the DOM in AngularJs using TypeScript.
By using ViewChild
, we get the ElementRef
of an HTML element inside the component class. Angular allows injecting ElementRef
of the directive element of the component when it is being requested in the constructor.
With the @ViewChild
decorator, we use the ElementRef
interface inside the class to get the element reference.
Consider the following example of code in AngularJs
in the file of main.component.html
. We have a button with an event and a div having a unique id myName
:
# angular
<div #myName></div>
<button (click)="getData()">Get Value</button>
Output:
Example 1 (app.component.ts
):
#angular
import { Component, VERSION, ViewChild, ElementRef } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./main.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent {
name = "Angular " + VERSION.major;
@ViewChild("myName") myName: ElementRef;
getData() {
console.log(this.myName);
this.myName.nativeElement.innerHTML = "I am changed by ElementRef & ViewChild";
}
}
Output:
Using the @ViewChild
decorator, we get the reference of the input with the help of the ElementRef
interface inside the class. We then change its value using the getData()
function with this reference.
Example 2 (app.component.ts
):
import { Component, ViewChild, ElementRef, AfterViewInit } from "@angular/core";
@Component({
selector: "my-app",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"]
})
export class AppComponent implements AfterViewInit {
name = "Angular";
@ViewChild("ipt", { static: true }) input: ElementRef;
ngAfterViewInit() {
console.log(this.input.nativeElement.value);
}
onClick() {
this.input.nativeElement.value = "test!";
}
}
Output:
This gets the reference of the input field and changes its inside text on the button click.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn