Angular subscribe() Method
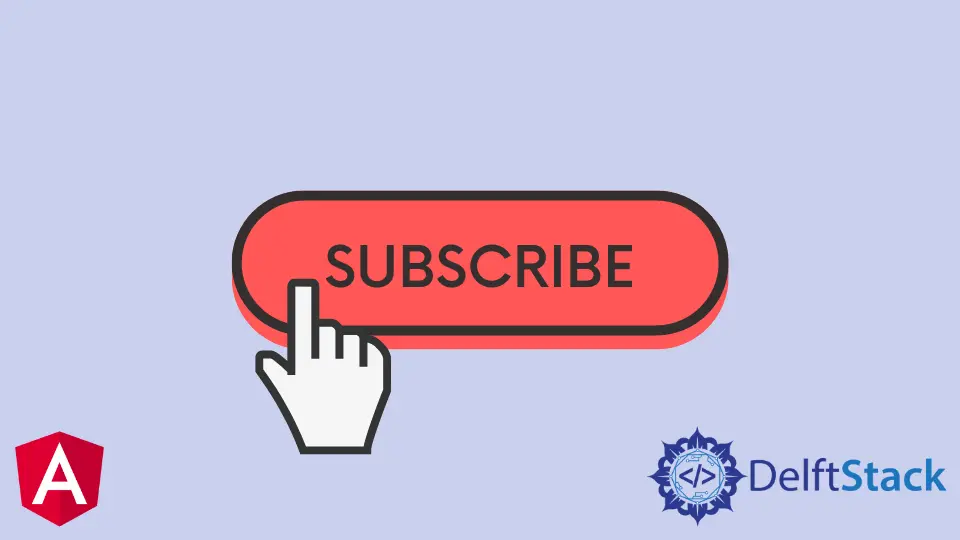
We will introduce Angular’s subscribe()
method and use it in our application.
Angular Subscribe
Subscribe()
is a method in Angular that connects the observer
to observable
events. Whenever any change is made in these observable, a code is executed and observes the results or changes using the subscribe
method. Subscribe()
is a method from the rxjs
library, used internally by Angular.
Newsletters are the most common part of websites now; let’s imagine them as an observable event and we as observers. When we enter our email to newsletter form on any website, we subscribe to an observable event. Whenever there is any new newsletter released from that website, we will receive it in our email until we unsubscribe to that event.
Similarly, we subscribe to that function if we have any function we want to observe on an Angular application. Whenever any change is made on that function, we can see the change using the subscribe()
method.
Subscribe()
takes three methods as a parameter: next
, error
, and complete
.
- Using the
next
method, we can pass every value emitted by theobservable
. - Using the
error
method, we can pass any error occurring somewhere in the stream. - Using the
complete
method, we can pass values once the whole observable event is complete.
Let’s go through an example to understand subscribe()
in detail. First, we will create a new service file as subscribe.service.ts
in which we will create the assignValue()
function that will pass a value to the observer using the next
method.
Our code in subsribe.service.ts
will look like this.
# angular
import { Injectable } from '@angular/core';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class SubscribeService {
assignValue() {
const SubscribeObservable = new Observable((observer) => {
observer.next('Angular');
});
return SubscribeObservable;
}
}
Next, we will import this service in app.module.ts
and inside @NgModule
we will define it as providers.
# angular
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { HelloComponent } from './hello.component';
import { SubscribeService } from './subscribe.service';
@NgModule({
imports: [BrowserModule, FormsModule],
declarations: [AppComponent, HelloComponent],
bootstrap: [AppComponent],
providers: [SubscribeService],
})
export class AppModule {}
In the next step inside the app.component.ts
file, we will create a constructor for our service and a function that will get value from the observer. And don’t forget to import our service in the app.component.ts
file.
# angular
import { Component, VERSION } from '@angular/core';
import { SubscribeService } from './subscribe.service';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
constructor(private SubscribeService: SubscribeService) {}
ngClick() {
this.SubscribeService.assignValue().subscribe((result) => {
console.log(result);
});
}
}
Now, we will create a template in app.component.html
.
# angular
<hello name="{{ name }}"></hello>
<button (click)="ngClick()">Click Here to see result</button>
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn