Difference Between Angular Service and Angular Factory
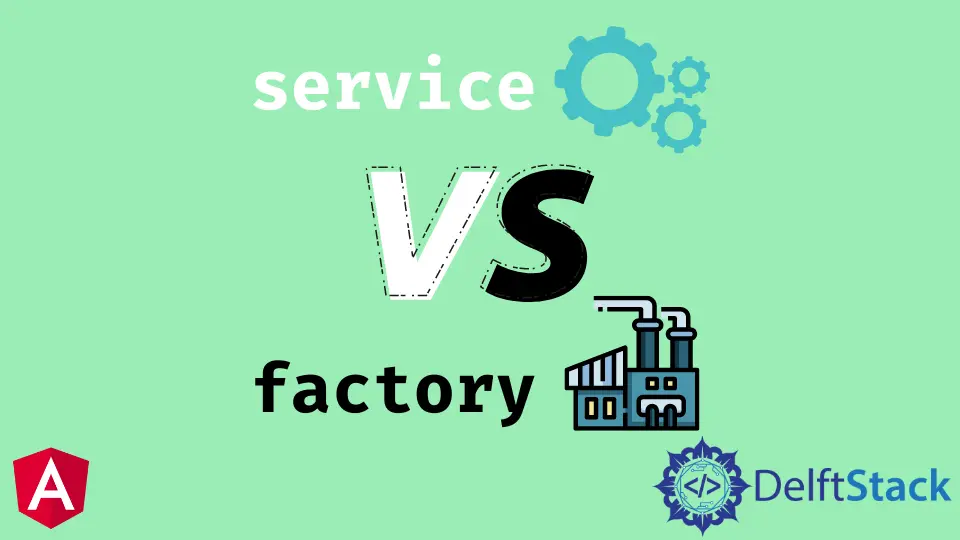
We will introduce the differences between the Angular service
and Angular factory
services.
We will also introduce the reasons to choose the Angular service
over the Angular factory
and vise versa.
Difference Between Angular Service
and Angular Factory
There appears to be a bit of confusion between the different service types that Angular provides, specifically the difference between the Angular service
and Angular factory
services.
The best way to differentiate between them is to create a service in both services and understand their differences.
We will create an Angular module called todoList
and assign it to the variable list
.
var list = angular.module('todoList', []);
Now, we will create the Angular service
called todoList
using both Angular service
and Angular factory
to see the difference. To create the Angular service
service, call the service
method on the module.
The first parameter of the service method is the service name. Let’s call it the todoListService
.
The second parameter to the service method is a function. Let’s pass an anonymous function that needs no arguments.
So, our code will look like below.
list.service("todoListService", function(){
})
Let’s implement the todoListService
.When we inject a service into a controller for the first time, Angular uses the new
keyword on the function reference we passed in a service method.
In other words, it treats our function as a constructor function. Two things happen automatically with a new
keyword; first, we get an empty object assigned to the this
variable.
Second, our function will return this object. The rest of the function is our definition of the service.
So, let us set up an array to store our list of to-dos. Our code will look like below.
list.service("todoListService", function(){
this.todoList = []
})
Let’s create two methods, addTodo
and removeTodo
, which we will not implement. Our code will be like below.
list.service("todoListService", function(){
this.todoList = []
this.addTodo = function(todo){
//Just to give idea
}
this.removeTodo = function(todo){
//Just to give idea
}
})
Therefore, our Angular service
is complete, which has the addTodo
function and removeTodo
function, which is all built as a service.
When we inject this, the variable this
gets returned to us and becomes our service. To create the Angular factory
service, call the factory method on the module.
The first parameter of the service method is the service name, and let’s call it the todoListFactory
. The second parameter to the service method is a function.
Let’s pass an anonymous function that needs no arguments.
list.factory("todoListFactory", function(){
})
Let’s implement the factory service.
The difference between the Angular service
and Angular factory
is that the Angular service
is nude as a constructor function. In contrast, the Angular factory
is just called, and whatever is returned from the function will be our service.
To implement our todoListFactory
, we have to create an empty object and return that object. The rest of our implementation is the same.
We will create the todoList
array and two functions addTodo
and removeTodo
. So our code will look like below.
list.factory("todoListFactory", function(){
var obj = {}
this.todoList = []
this.addTodo = function(todo){
//Just to give idea
}
this.removeTodo = function(todo){
//Just to give idea
}
return obj
})
We have two services, a factory
service and a service
service, the same. We can create a controller, inject our services, and be used identically; they both have the same methods and properties.
list.controller("todoController", function(todoListService, todoListFactory){
todoListService.addTodo(/*todo*/)
todoListFactory.addTodo(/*todo*/)
})
The main question is why would you choose to use a factory
over a service
since we can do the same thing with both of them. The answer is that if we want to return anything other than an object like a function, we must use a factory
.
Let’s say we wanted to have a service that allows us to create a to-do; to do this, we can create a factory
, and this factory
could return a function, which takes a description of a to-do and name.
That function will return a new object with the description and name set. And it will have a function to mark the to-do complete.
Our code will look like below.
list.factory("todoListF", factory(){
return function(description, name){
return{
description: description,
name: name,
complete: function(){
//to-do completed
}
}
}
})
So, we can inject our new todoList
factory
service into our controller and use it to create new to-dos, such as a task to-do and an updated to-do.
list.controller("todoFController", function(todoListF){
var task = new todoListF("Push code to github", "Github Push Code")
var update = new todoListF("Update code on github repository", "Update Repository")
})
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn