How to Redirect to Another Page Using AngularJS
-
Use the
$location
Service in AngularJS to Redirect to Another Page -
Use the
$window
Service to Perform a Full-Page Redirect - Conclusion
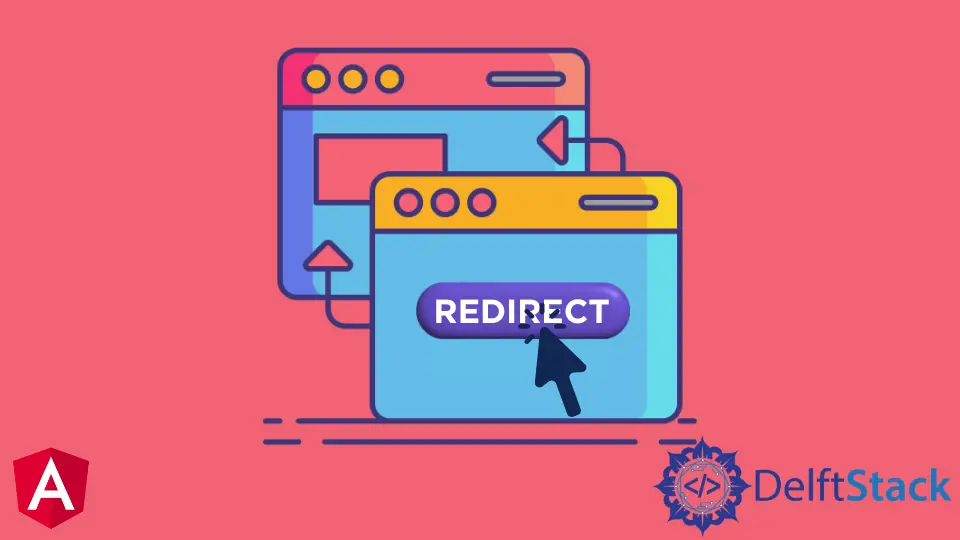
AngularJS is a powerful JavaScript framework that simplifies the process of building web applications by extending the HTML vocabulary for your application.
In this tutorial, we will delve into how to effectively utilize AngularJS to redirect to another page, providing a seamless user experience.
Use the $location
Service in AngularJS to Redirect to Another Page
The $location
service in AngularJS is a versatile tool that allows you to manage URLs within your web application. It serves the purpose of retrieving the current page’s URL and making modifications as needed, including redirecting to new pages.
Moreover, it assists in handling browser address bar changes, user-initiated link clicks, pop-ups, and bookmarking, ensuring a smooth navigation experience.
One of the primary advantages of using the $location
service is its ability to facilitate navigation within your application without requiring you to switch between controllers or write custom code to handle URL changes.
It simplifies the process of URL management through a variety of methods, such as $location.href()
, $location.absUrl()
, url()
, protocol()
, host()
, port()
, path([path])
, replace()
, state()
, search([paramValue])
, and hash()
. These methods allow you to redirect users to new URLs or modify the current URL with ease.
There are primarily two ways to apply the $location
service for URL redirection:
-
Using
$location.url(URL)
This method is used to change the URL and navigate to a different route by specifying the entire URL, including the protocol and hostname.
Syntax:
$location.url('/new-location');
This is suitable for navigating to different routes within your AngularJS application and can also be used to navigate to external websites by providing a full URL. However, it triggers a full page reload if the URL is from a different origin (cross-origin navigation).
-
Using
$location.path(URL)
This is used to change the route part of the URL (the part after the hostname) while keeping the protocol and hostname intact.
Syntax:
```javascript
$location.path('/new-location');
```
This is ideal for navigating between different routes within your AngularJS application without triggering a full page reload. This method is not suitable for navigating to external websites because it doesn't modify the hostname or protocol.
Use the $window
Service to Perform a Full-Page Redirect
After employing the $location
service for URL manipulation, you can utilize $window
to refresh the page. To do this with $window.location.href
, follow these steps:
Here’s a simplified example to illustrate how to redirect to another page or URL using AngularJS:
HTML Code:
<div ng-app="Demo" ng-controller="Sample">
<h3>Example of Redirecting to Another Page using AngularJS</h3>
<br>
<br>
<button ng-click="location()">Click here to experience AngularJS magic</button>
</div>
JavaScript Code:
var app = angular.module('Demo', []);
app.controller('Sample', function($scope, $window, $location) {
$scope.location = function(){
var url = "https://www.delftstack.com/";
$window.location.href = url;
}
});
In the HTML code above, the ng-click
directive binds an action to the button’s click event. In this case, when the button is clicked, it will invoke the $scope.location
function defined in the controller.
Then, in the JavaScript code, we use the $window
service to set the location.href
property to the URL defined. This action triggers a full-page redirect to the specified URL, effectively taking us to https://www.delftstack.com/
when the button is clicked.
Output:
Click here to run the sample code.
Conclusion
In a nutshell, AngularJS’s $location
service allows us to effortlessly manage URLs and implement smooth redirection within web applications. By combining this service with the $window
object, we can efficiently refresh pages and guide users to specific URLs with ease.
This streamlined approach enhances user experiences and simplifies the development of dynamic, user-friendly web applications.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook