Date Format in Angular
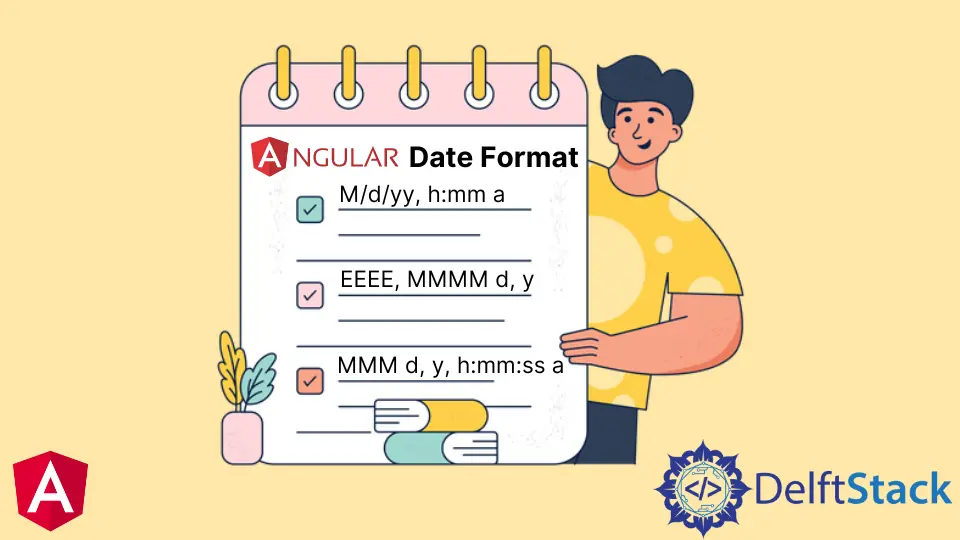
We will introduce how to use, change or add elements and display dates from a timezone
in date format using DatePipe
in Angular.
Date Pipe in Angular
Angular date pipe is used to format dates in Angular according to the provided date format, time zone, and country locale information.
Using the DatePipe
, we can easily convert a date object, a number, or an ISO date string according to given predefined Angular date formats or custom Angular date formats.
Angular DatePipe
accepts three parameters: format
, timezone
, and locale
.
format
We can pass predefined date formats or custom date formats in format parameters.
timezone
In the timezone
parameter, the default time zone is the local system time zone of the user machine. We can change the time zone via passing time zone offset
(‘0510’) or standard UTC/GMT (CET) or continental US time zone abbreviation, and it is an optional parameter.
locale
locale
represents locale format rules to use. The default value of the locale is our project locale (en_US
) if set or undefined. Locale is an optional parameter.
Angular Date Pipe Examples
We will discuss a few examples with different date formats to better understand Angular DatePipe
.
First of all, we will add this code in our app.component.ts
.
#angular
import { Component, OnInit } from '@angular/core';
import { DatePipe } from '@angular/common';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements OnInit {
today: Date = new Date();
pipe = new DatePipe('en-US');
todayWithPipe = null;
ngOnInit(): void {
this.todayWithPipe = this.pipe.transform(Date.now(), 'dd/MM/yyyy');
}
}
We imported DatePipe
from @angular/common
in the above code. We have exported a class AppComponent
implemented on Initial, inside which we have created a new DatePipe
with en-US
locale.
We will add code to app.component.html
to display the date with our predefined date format dd/MM/yyyy
.
<div>Today Date is: {{ todayWithPipe }}</div>
Output:
List of Predefined Date Formats in Angular
Date Format | Result |
---|---|
M/d/yy, h:mm a |
12/2/21, 4:38 PM |
MMM d, y, h:mm:ss a |
Dec 2, 2021, 4:39:12 PM |
MMMM d, y, h:mm:ss a z |
December 2, 2021, 4:39:35 PM GMT+5 |
EEEE, MMMM d, y, h:mm:ss a zzzz |
Thursday, December 2, 2021, 4:39:55 PM GMT+05:00 |
M/d/yy |
12/2/21 |
MMM d, y |
Dec 2, 2021 |
MMMM d, y |
December 2, 2021 |
EEEE, MMMM d, y |
Thursday, December 2, 2021 |
h:mm a |
4:42 PM |
h:mm:ss a |
4:43:52 PM |
h:mm:ss a z |
4:44:32 PM GMT+5 |
h:mm:ss a zzzz |
4:45:00 PM GMT+05:00 |
Angular DatePipe
has 12 predefined formats, as shown in the above table. But we can also create our custom date formats and include some more elements like Era, Week of year, Week of the month, Day of the month, etc.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn