How to Format Date using Pipe in Angular
- How to use Angular Date Pipe?
- Time zone example using an angular date pipe
- Example of an angular date pipe with a country location
- Create a custom date pipe in Angular:
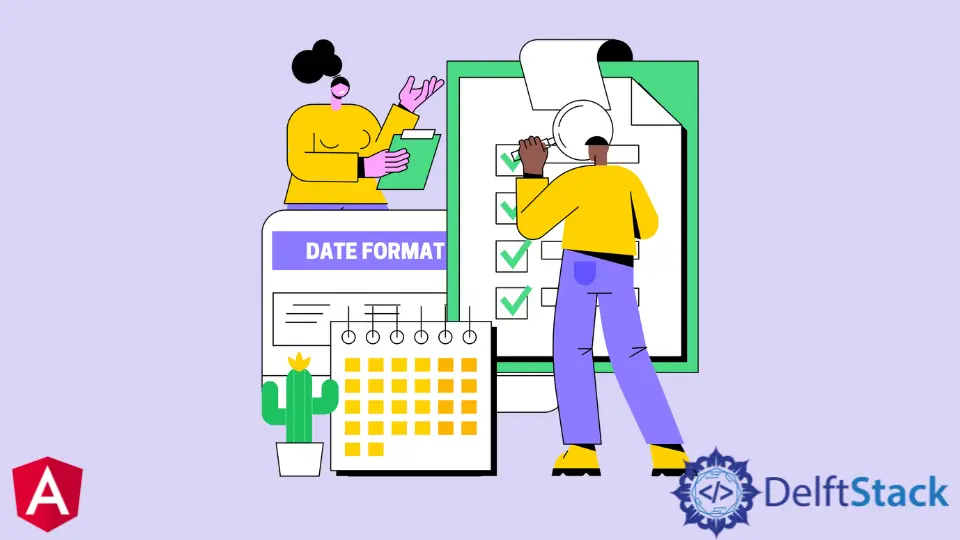
Angular Date Pipe lets us format dates in Angular using the specified format, time zone, and specific details. It has pre-defined formats and Custom format strings. In Custom format strings, we can easily customize the date format, time zone, country locale, and so on; with the help of some essential steps, we will discuss all of these steps in detail.
This article will clear all of your doubts and demonstrate Format Date Using Pipe in Angular with the help of examples. Let’s Begin.
How to use Angular Date Pipe?
The @angular/common component introduces the Date Pipe. Pipes can be used to format data in Angular, including values, percent, dates, and much more. Mainly, three parameters are considered when designing an angular date pipe.
Format
Time zone
Locale
Before discussing the parameters mentioned above, it’s essential to know the syntax of the angular date pipe by using the format, timezone, and locale.
{{ date_Value | date [ : format [ : timeZone [ : locale ] ] ] }}
Parameters and Description of Angular Date Pipe
Format
: As a format parameter, we can give standard angular date formats or custom date format. mediumDate
is the default value.
Time zone
: The standard Time zone is the user machine’s local system time zone. We can give time zone offset (0530
), conventional UTC/GMT (IST), or the eastern US time zone acronym as an additional parameter to adjust the Time zone.
Locale
: It indicates the locale format rules that should be used. If undefined or configured, the default value is our project locale (en-US
).
Moreover, you need to know that Angular comes with only the en-US locale data out of the box. You must import relevant locale data to localize the dates in any language.
List of all predefined date formats in Angular
Angular date pipe has 12 predefined formats. Let’s discuss the list of all these built-in date formats in-detail.
short
:M/d/yy, h:mm a
,1/01/22, 11:45 PM
. Its Angular datepipe code is{{todayDate | date:'short'}}
medium
:MMM d, y, h:mm:ss a
,Jan 01, 2022, 11:45:13 PM
. Its Angular datepipe code is{{todayDate | date:'medium'}}
long
:MMMM d, y, h:mm:ss a z
,Jan 01, 2022 at 11:45:13 PM GMT+5
. Its Angular datepipe code is{{todayDate | date:'long'}}
longDate
:MMMM d, y, Jan 01, 2022
. Its Angular datepipe code is{{todayDate | date:'longDate'}}
fullDate
:EEEE, MMMM d, y, Saturday
,Jan 01, 2022
. Its Angular datepipe code is{{todayDate | date:'fullDate'}}
shortTime
:h:mm a
,11:45 PM
. Its Angular datepipe code is{{todayDate | date:'shortTime'}}
mediumTime
:h:mm:ss a
,11:45:13 PM
. Its Angular datepipe code is{{todayDate | date:'mediumTime'}}
longTime
:h:mm:ss a z
,11:45:13 PM GMT+5
. Its Angular datepipe code is{{todayDate | date:'longTime'}}
fullTime
:h:mm:ss a zzzz
,11:45:13 PM
. Its Angular datepipe code is{{todayDate | date:'fullTime'}}
full
:EEEE, MMMM d, y, h:mm:ss a zzzz
,Saturday, Jan 01, 2021 at 11:45:13 PM GMT+05:30
. Its Angular datepipe code is{{todayDate | date:'full'}}
shortDate
:M/d/yy
,1/01/22
. Its Angular datepipe code is{{todayDate | date:'shortDate'}}
mediumDate
:MMM d, y
,Jan 01, 2022
. Its Angular datepipe code is{{todayDate | date:'mediumDate'}}
Click here to check the format of date and time for further clarification.
Time zone example using an angular date pipe
We can give the time zone as a parameter to the date pipe and the date format to show the date in a specific UTC. Time zone deviation (‘0530’), conventional UTC/GMT (IST), or continental US time zone acronym are all options for the time zone parameter.
For instance, to show the time in the IST timezone.
Today is {{todayDate | date:'short':'IST'}}
Today is {{todayDate | date:'short':'+05:00'}}
Output:
Today is 1/02/19, 3:30 PM
Example of an angular date pipe with a country location
It is necessary to give country locale code as a third argument to angular date pipe to display date according to country locale format standards, as seen below.
Germany, for instance, uses German Standard Time
and has a timezone offset of +01:00
. Use the locale code de
as a parameter in Angular to display date and time in the Germany locale, as demonstrated below.
<p>The current German date time is {{todayDate | date:'full':'+01:00':'de'}}</p>
Output:
The current German date time is Mittwoch, 5. Januar 2022 um 12:50:38 GMT+01:00
Create a custom date pipe in Angular:
Angular uses the mediumDate
date format by default. What if we wish to alter it and use our own unique format instead of built-in formats, such as MMM d, y, h:mm: ss a
?
This shows the time as January 01, 2022, 11:45:13 PM
.
We’ll be showing dates a lot in our angular applications, and we’ll need to pass the format argument every time. As seen below, we may create our custom date pipe and use it throughout the application to circumvent this.
{{ todayDate | customDate }}
Output:
January 01, 2022, 11:45:13 PM
Follow the instructions below to make a custom date pipe. Add the following code to a file called custom.datepipe.ts.
import { Pipe, PipeTransform } from '@angular/core';
import { DatePipe } from '@angular/common';
@Pipe({
name: 'customDate'
})
export class CustomDatePipe extends
DatePipe implements PipeTransform {
transform(value: any, args?: any): any {
return super.transform(value, " MMM d, y, h:mm:ss a "); } }
After doing this, the next step is to import CustomDatePipe and add it to the AppModule declaration array.
import {CustomDatePipe} from './custom.datepipe';
@NgModule({
declarations: [
CustomDatePipe
]);
Now, we are on the stage where we can utilize our custom date pipe in the component file.
{{todayDate | customDate}}
Output:
Jan 5, 2022, 5:25:36 PM
Custom Date Formats:
In Angular, you can create your own date formats. The following is a comprehensive list of all possible custom date formats.
Type | Format | Description | Examples |
---|---|---|---|
Era |
G , GG & GGG |
Abbreviated | AD |
GGGG |
Wide | Anno Domini |
|
GGGGG |
Narrow | A |
|
Year: | y |
Numeric: minimum digits | 2022 |
yy |
Numeric: 2 digits and zero padded | 22 |
|
yyy |
Numeric: 3 digits and zero padded | 2022 |
|
yyyy |
Numeric: 4 digits or more and zero padded | 2022 |
|
Month | M |
Numeric: 1 digit | 1 |
MM |
Numeric: 2 digits and zero padded | 01 |
|
MMM |
Abbreviated | Jan |
|
MMMM |
Wide | January |
|
MMMMM |
Narrow | J |
|
Month standalone | L |
Numeric: 1 digit | 8 |
LL |
Numeric: 2 digits + zero padded | 08 |
|
LLL |
Abbreviated | Aug |
|
LLLL |
Wide | August |
|
LLLLL |
Narrow | A |
|
Day of month: | d |
Numeric: minimum digits | 7,8 |
dd |
Numeric: 2 digits + zero padded | 13,08,19 |
|
Week day | E , EE & EEE |
Abbreviated | Thu |
EEEE |
Wide | Thursday |
|
EEEEE |
Narrow | T |
|
EEEEEE |
Short | Th |
|
Week of month | W |
Numeric: 1 digit | 2,6 |
Week of year | w |
Numeric: minimum digits | 7,34 |
ww |
Numeric: 2 digits | 43, 09 |
|
Period | a , aa & aaa |
Abbreviated | am , pm |
aaaa |
Wide | pm |
|
aaaaa |
Narrow | a |
|
Period* | B , BB & BBB |
Abbreviated | mid |
BBBB |
Wide | am , pm , midnight , afternoon , noon |
|
BBBBB |
Narrow | md |
|
Period standalone* | b , bb & bbb |
Abbreviated | mid |
bbbb |
Wide | midnight , afternoon , evening , night |
|
bbbbb |
Narrow | mid |
|
Fractional seconds | S |
Numeric: 1 digit | 5,7,2 |
SS |
Numeric: 2 digits and zero padded | 96,87,47 |
|
SSS |
Numeric: 3 digits and zero padded | 435,789,670 |
|
Second | s |
Numeric: minimum digits | 3,9,56 |
ss |
Numeric: 2 digits and zero padded | 09,26,13 |
|
Minute | m |
Numeric: minimum digits | 40,6,47,54 |
mm |
Numeric: 2 digits and zero padded | 04,51,23,28 |
|
Hour 0 to 23 | H |
Numeric: minimum digits | 16 |
HH |
Numeric: 2 digits and zero padded | 21,19,17 |
|
Hour 1 to 12 | h |
Numeric: minimum digits | 11 |
hh |
Numeric: 2 digits and zero padded | 12 |
|
Zone | z , zz & zzz |
Short specific non location format | GMT-6 |
zzzz |
Long specific non location format | GMT-06:00 |
|
Z , ZZ & ZZZ |
ISO8601 basic format | -0600 |
|
ZZZZ |
Long localized GMT format | GMT-06:00 |
|
ZZZZZ |
ISO8601 extended format | -06:00 |
|
O , OO & OOO |
Short localized GMT format | GMT-6 |
|
OOOO |
Long localized GMT format | GMT -06:00 |
|
Custom Format example:
Here, we mentioned a very simple example of a custom format.
{{todayDate | date:'dd/MM/yy HH:mm'}}
Output:
05/01/22 17:25
If you want to learn more about the Angular date pipe, click here
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook