How to Add Conditional Classes With ngClass in Angular
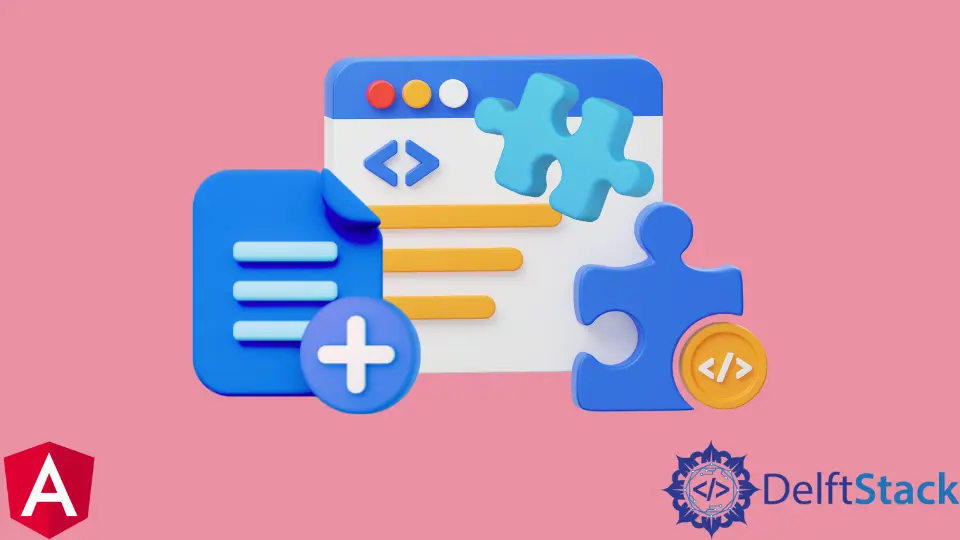
We will introduce how to add conditional classes or use an if-else
condition to display dynamic classes with ngClass
in our Angular applications.
Add Conditional Classes With ngClass
in Angular
Classes are the central part of designing and creating the UI of our applications. We write designs based on the classes assigned to different parts of our applications.
Sometimes we have to add dynamic classes or change classes based on conditions.
This tutorial will discuss different ways to achieve an if-else
statement inside ngClass
in Angular.
Let’s create a new application using the following command.
# angular
ng new my-app
After creating our new application in Angular, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# angular
ng serve --open
Let’s start with a simple example in which we will try to add a class to an HTML element if the condition message is set as shown below in app.component.ts
.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
message = 'warning';
}
Now, let’s add an HTML element with ngClass
with the condition using the variable message in app.component.html
.
# angular
<div [ngClass]="{'warning': message}">
Hello this is a warning message
</div>
Then, let’s add some CSS code to the class we are adding in the div based on the condition below.
# angular
.warning {
font-family: Lato;
background: red;
color: white;
padding: 10px;
}
Output:
As you can see from the example, it is easy to use the if
statement inside ngClass
. We straightforwardly added a class based on the condition.
Now, we will go through an example in which we will add a class based on the true condition. If the condition is false, we will add different classes.
So, first, we will add a condition in app.component.html
and add a button with a click event that will change the condition, as shown below.
# angular
<div [ngClass]="Warnmessage ? 'warning' : 'info'">
Hello this is a warning message
</div>
<button (click)=changeClass()>Click this</button>
Now, let’s create a variable Warnmessage
and a function that will change the condition when clicking the button. Our code in app.component.ts
will look like the below snippet.
# angular
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent {
name = 'Angular ' + VERSION.major;
Warnmessage = true;
changeClass() {
this.Warnmessage = false;
console.log('Button Clicked');
}
}
Let’s run this code and check how it works.
Output:
As you can see, we used the if-else
statement as well inside ngClass
, and it works on conditions. But if you want to add more than one class based on a single condition, it can also be done by adding a space and writing a new class name after it.
# angular
<div [ngClass]="Warnmessage ? 'warning message-box' : 'info message-box'">
Hello this is a warning message
</div>
<button (click)=changeClass()>Click this</button>
We can also add multiple classes by adding a space after the first class name and writing the second class name. We can even add triple classes or as many we want.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn