NumPy numpy.random.rand() Function
-
Syntax of
numpy.random.rand()
: -
Example Codes:
numpy.random.rand()
Method -
Example Codes: Specify Shape of Output Array
numpy.random.rand()
Method
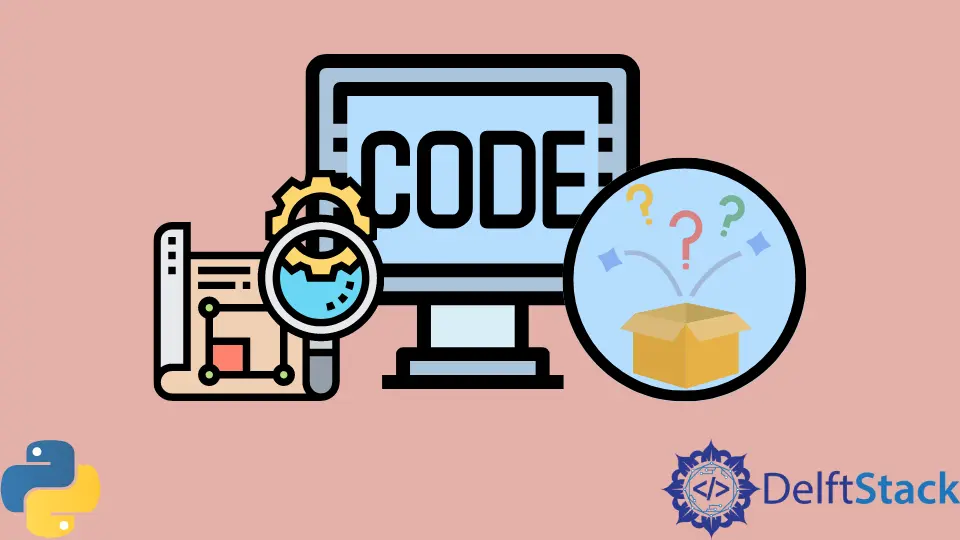
Python Numpynumpy.random.rand()
function generates an array of specified shape with random values.
Syntax of numpy.random.rand()
:
numpy.random.rand(d0, d1, ..., dn)
Parameters
d0, d1, ..., dn |
Integer. Represent the dimension of the output array from the random function. If no value is specified, a scalar value is returned. |
Return
It returns a random array of specified shapes with random values.
Example Codes: numpy.random.rand()
Method
import numpy as np
x = np.random.rand()
print(x)
Output:
0.6222151413197674
It generates a random number since no size is specified for the output array.
The range of generated output number lies between 0 and 1.
You might get different random numbers when you run the same code multiple times.
To generate the constant output, we fix the seed
of np.random()
function.
import numpy as np
np.random.seed(0)
x = np.random.rand()
print(x)
Output:
0.5488135039273248
It generates a constant output every time we run the function.
Example Codes: Specify Shape of Output Array numpy.random.rand()
Method
To generate arrays of fixed size and shapes, we specify parameters that determine the shape of the output array in numpy.random.rand()
function.
Generate 1-D Arrays With numpy.random.rand()
Method
import numpy as np
np.random.seed(0)
x = np.random.rand(5)
print(x)
Output:
[0.5488135 0.71518937 0.60276338 0.54488318 0.4236548 ]
It generates a random 1-dimensional array with the length 5
composed of random numbers.
The numbers here will also lie in the range (0,1)
.
Due to the fixed seed
, the same random numbers are generated every time we run it.
If we need to generate numbers greater than 1
, we can simply multiply the array by the desired range.
import numpy as np
np.random.seed(0)
x = np.random.rand(5)*10
print(x)
Output:
[5.48813504 7.15189366 6.02763376 5.44883183 4.23654799]
It generates random numbers ranging from 1 to 10.
Generate 2-D Arrays With numpy.random.rand()
Method
import numpy as np
np.random.seed(0)
x = np.random.rand(2,3)
print("Array x:")
print(x)
print("\n Shape of Array x:")
print(x.shape)
Output:
Array x:
[[0.5488135 0.71518937 0.60276338]
[0.54488318 0.4236548 0.64589411]]
Shape of Array x:
(2, 3)
This generates 2-dimensional random array with 2 rows and 3 columns using numpy.random.rand()
method.
Generate Higher Dimensional Arrays With numpy.random.rand()
Method
import numpy as np
np.random.seed(0)
x = np.random.rand(2,3,2,3)
print("Array x:")
print(x)
print("\n Shape of Array x:")
print(x.shape)
Output:
Array x:
[[[[0.5488135 0.71518937 0.60276338]
[0.54488318 0.4236548 0.64589411]]
[[0.43758721 0.891773 0.96366276]
[0.38344152 0.79172504 0.52889492]]
[[0.56804456 0.92559664 0.07103606]
[0.0871293 0.0202184 0.83261985]]]
[[[0.77815675 0.87001215 0.97861834]
[0.79915856 0.46147936 0.78052918]]
[[0.11827443 0.63992102 0.14335329]
[0.94466892 0.52184832 0.41466194]]
[[0.26455561 0.77423369 0.45615033]
[0.56843395 0.0187898 0.6176355 ]]]]
Shape of Array x:
(2, 3, 2, 3)
This generates 4-dimensional random array with shape (2, 3, 2, 3)
using numpy.random.rand()
method.
Similarly, we can also generate any random arrays of any size using the numpy.random.rand()
method.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn