JavaScript String.charCodeAt() Method
-
Syntax of JavaScript
string.charCodeAt()
: -
Example Codes: Find the ASCII of Character in a String Using JavaScript
string.charCodeAt()
Method -
Example Codes: Get the Unicode of a Character With JavaScript
string.charCodeAt()
Method -
Example Codes: Out of Bound Parameter in JavaScript
string.charCodeAt()
Method
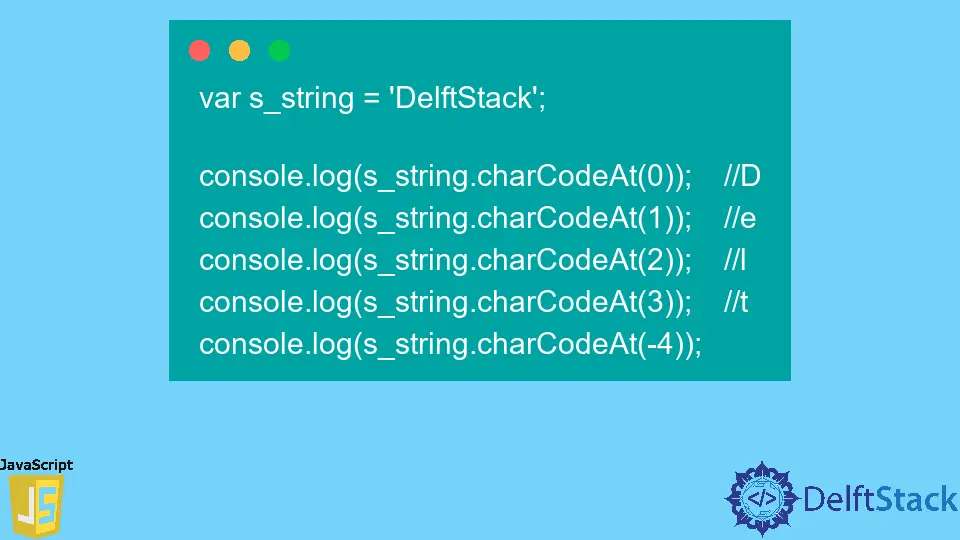
The string.charCodeAt()
is a JavaScript method that gives the UTF-16 Unicode unit of the specified i
-th character of the calling string object. The index of the i
-th character is passed as a parameter to the method.
Syntax of JavaScript string.charCodeAt()
:
string.charCodeAt(i);
Parameter
i |
This is the integer specifying the i -th character of the string. The value of i ranges from 0 to len -1 of the string, where len is the length of the string. |
If the i parameter is not passed to the function, by default, its value is 0. |
Return
The JavaScript string.charCodeAt()
method returns the UTF-16 unit code of the specified i
-th character of the string as an integer-type object. If the i
parameter of the string.charCodeAt()
method is not in the range of 0 to string length, it will return NaN
.
- The UTF-16 unit code is a 16-bit number between 0 and 65535. The
string.charCodeAt()
will always return an integer value less than 65535. - When a Unicode value (values greater than
0xFFFF
), the method returns the first component of a pair of the code.
Example Codes: Find the ASCII of Character in a String Using JavaScript string.charCodeAt()
Method
The first 128 UTF-16 multi-byte codes of characters are the same as the ASCII single-byte codes of those characters. If the returned number is between 0 and 128, then it is the ASCII of that character.
const string = 'welcome to hello world';
const i = 4;
console.log(`The ASCII code of character at index ${i} in the string is ${string.charCodeAt(i)}.`);
Here, i
is the position of the character. In the above example, the string.charCodeAt()
method returns an integer number that indicates the UTF-16 code of the 4th character of the string.
Output:
The ASCII code of character at index 4 in the string is 111.
As the character at index 4 of the string is o
, the string.charCodeAt()
method returns the Unicode of o
.
Example Codes: Get the Unicode of a Character With JavaScript string.charCodeAt()
Method
The string.charCodeAt()
can also be used to get the Unicode of a character in a string.
const character='a';
console.log(character.charCodeAt());
console.log(character.charCodeAt(0));
Here, passing the i
parameter as 0
or passing no parameter to the method gives a similar result as the JavaScript string.charCodeAt()
parameter is set to 0
at default.
Output:
97
97
Example Codes: Out of Bound Parameter in JavaScript string.charCodeAt()
Method
In this example, to get the Unicode value for a character at a certain point, we called the charCodeAt()
method of the s_string
variable.
var s_string = 'DelftStack';
console.log(s_string.charCodeAt(0)); //D
console.log(s_string.charCodeAt(1)); //e
console.log(s_string.charCodeAt(2)); //l
console.log(s_string.charCodeAt(3)); //t
console.log(s_string.charCodeAt(-4));
Output:
68
101
108
102
NaN
The console prints NaN
as the i
parameter was out of range.