Python Tutorial - File Directory Operation
- Create a Directory in Python
- Get Current Directory in Python
- List Directories in Python
- Change Working Directory
- Rename and Remove a Directory
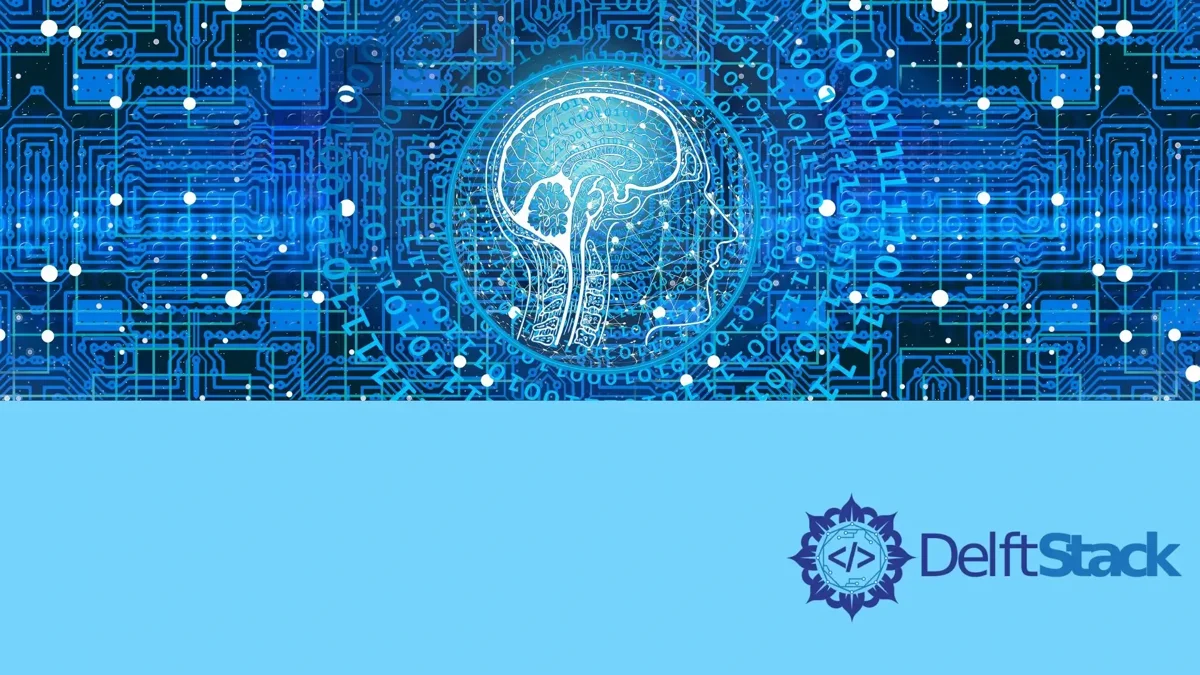
In Python, if you perform operations on directories, you need import the os
module. The functions of os
module can be used to do file and directory operations.
Create a Directory in Python
A new directory can be created by using mkdir()
method. You have to specify the path in which you want to create the directory. If the path is not specified, the directory will be created in current directory.
>>> import os
>>> os.mkdir("PythonTutorials")
A new directory named PythonTutorials
will be created in the current working directory.
Get Current Directory in Python
The getcwd()
method is used to get the current working directory,
>>> import os
>>> print(os.getcwd())
C:\Users\HP\AppData\Local\Programs\Python\Python36-32
List Directories in Python
To list the files and subdirectories, listdir()
method is used. It lists the files and subdirectories of the Python script file if there is no argument given. Otherwise, it lists the content of the given path.
>>> import os
>>> print(os.listdir())
['DLLs', 'Doc', 'get-pip.py', 'hello.py', 'include', 'Lib', 'libs', 'LICENSE.txt', 'NEWS.txt', 'python.exe', 'python3.dll', 'python36.dll', 'pythonw.exe', 'Scripts', 'tcl', 'Tools', 'vcruntime140.dll']
>>> print(os.listdir(r"C:\Program Files"))
['7-Zip', 'Common Files', 'Microsoft Office', 'Windows Sidebar']
Change Working Directory
To change the current working directory, chdir()
method is used.
>>> import os
>>> os.chdir("C:/Users/HP/Desktop/Code")
>>> print(os.getcwd())
C:\Users\HP\Desktop\Code
Rename and Remove a Directory
Rename a Directory
A file or directory can be renamed by using rename()
function.
>>> import os
>>> os.rename("PythonTutorials", "Python")
The new name of the directory is Python
now.
Remove a Directory
A directory can be removed by using rmdir()
method.
>>> import os
>>> os.rmdir('Python')
It removes the directory Python
from the system.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook