Python Tutorial - Exception Handling
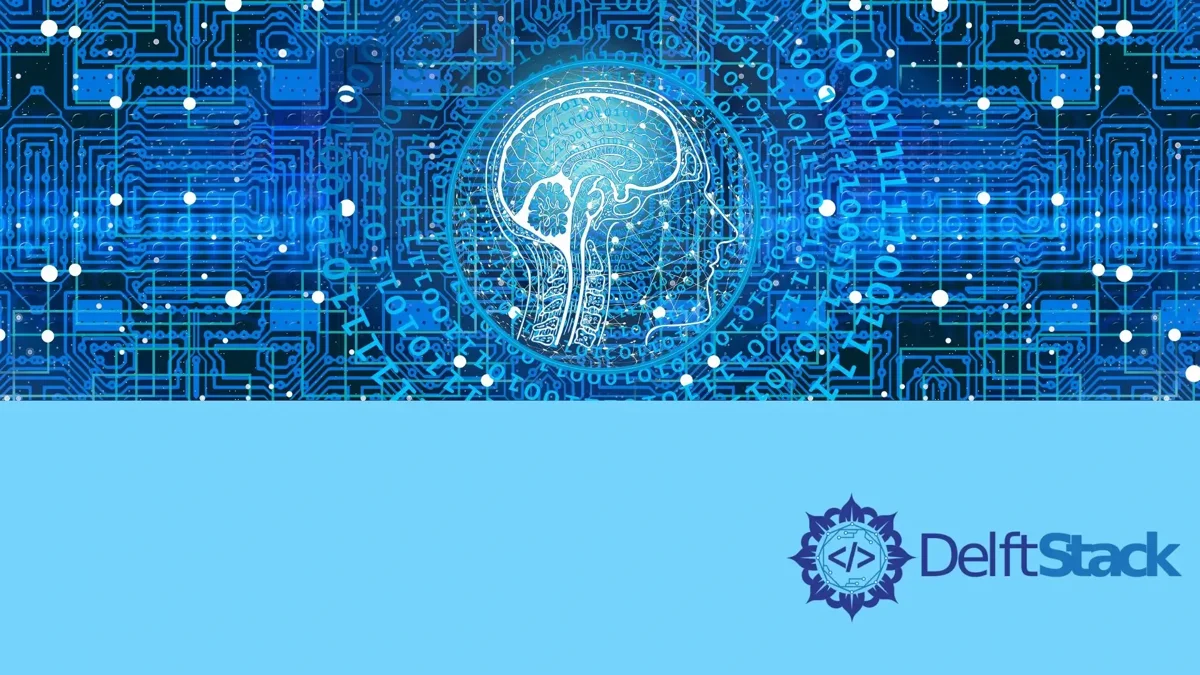
You will learn how to handle Python exceptions in this section. Further, you will learn to raise and catch exceptions.
When an exception occurs in a program, the program execution is terminated. But most of the time, you don’t prefer the abnormal termination of the program, therefore you could use Python exceptions handling to avoid the crash of your program.
try...except
In the following example, the user is asked to enter 10 integers. If the user enters another type rather than integers, an exception will be thrown and statements in except
block is executed:
>>> n = 10
>>> i = 1
>>> sum = 0
>>> while i <= n:
try:
num = int(input('Enter a number: '))
sum = sum + num
i = i+1
except:
print('Please enter an integer!!')
break
Enter a number: 23
Enter a number: 12
Enter a number: Asd
Please enter an integer!!
>>> print('Sum of numbers =', sum)
Sum of numbers = 35
You can also catch specified exceptions, for example, if division by zero happens, you can catch the corresponding exception as:
try:
# statements
except ZeroDivisionError:
# exception handling
except:
# all other exceptions are handled here
raise
Exception
You can force a specific exception to occur by using raise
keyword:
>>> raise ZeroDivisionError
Traceback (most recent call last):
File "<pyshell#17>", line 1, in <module>
raise ZeroDivisionError
ZeroDivisionError
>>> raise ValueError
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise ValueError
ValueError
If you need to add some customized information to the self-raised exception, you could add it in the parenthesis after exception error type.
>>> raise NameError("This is a customized exception")
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise NameError("This is a customized exception")
NameError: This is a customized exception
try...finally
The finally
block is optional in the try
block. If there is a finally
block, it will be executed in any case.
This is demonstrated in the example below:
>>> try:
raise ValueError
finally:
print('You are in finally block')
You are in finally block
Traceback (most recent call last):
File "<pyshell#23>", line 2, in <module>
raise ValueError
ValueError
The try...finally
block is used for the clean-up actions such as when working with a file, it is a good practice to close the file. So the file will be closed in the finally
block.
Python Built-In Exceptions
In this section, you will learn about the standard exceptions in Python programming.
Exceptions are the runtime errors that are raised during interpretation. For example, the division of any number by zero will create a runtime error called ZeroDivisionError
.
In Python, there are some built-in exceptions that are described below:
Exception | When it is raised? |
---|---|
AssertionError |
when the assert statement is failed |
AttributeError |
when the attribute reference is failed |
EOFError |
when no data is read by the input() functions and the end of file is reached. |
FloatingPointError |
when the floating point calculation is failed |
GeneratorExit |
When a coroutine or a generator is closed, GeneratorExit exception occurs |
ImportError |
when import cannot find the module |
ModuleNotFoundError |
when the module is not found by import . This exception is a subclass of ImportError . |
IndexError |
when the index of a sequence is not in range. |
KeyError |
when a dictionary key is not found in the dictionary |
KeyboardInterrupt |
when an interrupt key is pressed. |
MemoryError |
when there is less amount of memory for a specified operation |
NameError |
when a variable is not found |
NotImplementedError |
when the implementation of the abstract method is needed but not provided. |
OSError |
when a system error is encountered |
OverFlowError |
when a value is too large to be represented. |
RecursionError |
when the recursion limit exceeds the maximum number |
IndentationError |
when indentation is properly specified for the definition of any function, class, etc. |
SystemError |
when a system related error is found |
SystemExit |
when sys.exit() is used to exit interpreter. |
TypeError |
when an operation is invalid for the specified data type |
ValueError |
when a built-in function has valid arguments but the values provided are not valid. |
RunTimeError |
when the generated error falls in no other category |
IOError |
when an I/O operation is failed. |
UnBoundLocalError |
when the local variable is accessed but has no value |
SyntaxError |
when there is a syntax error |
TabError |
when there is unnecessary tab indentation |
UnicodeError |
when a Unicode related error is encountered |
UnicodeEncodeError |
when a Unicode related error is encountered because of encoding |
UnicodeDecodeError |
when a Unicode related error is encountered because of decoding |
UnicodeTranslateError |
when a Unicode related error is encountered because of translating |
ZeroDivisionError |
when a number is divided by zero |
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook