Python チュートリアル - 例外処理
胡金庫
2023年1月30日
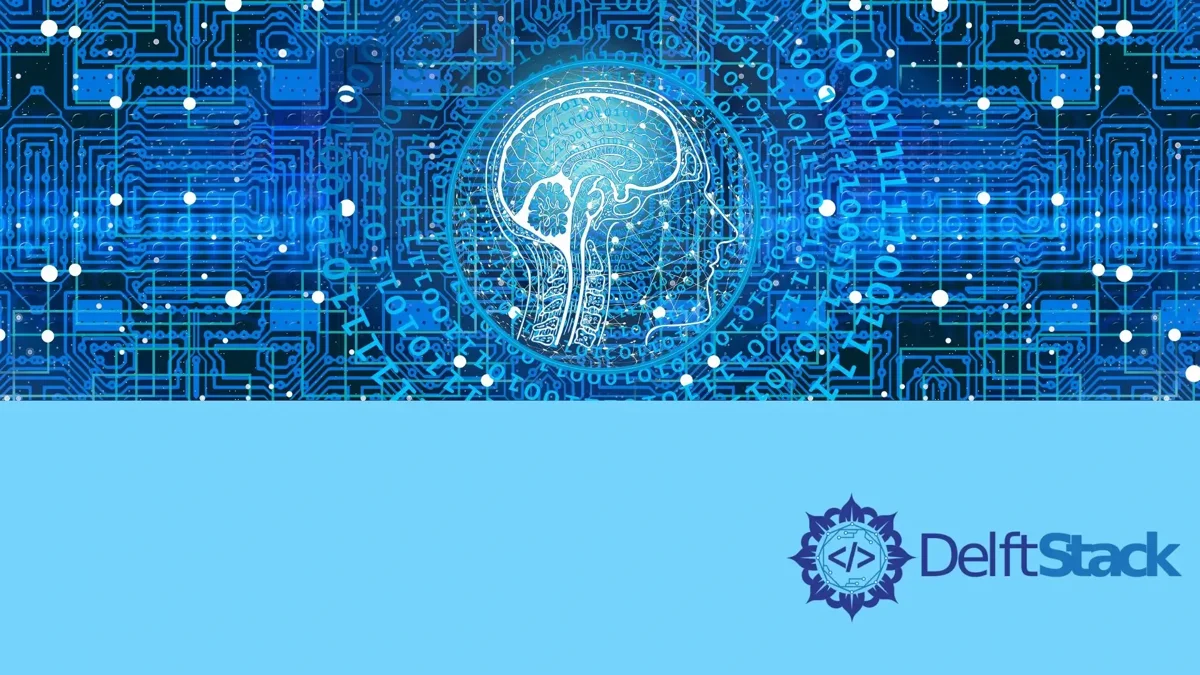
このセクションでは、Python 例外を処理する方法を学習します。さらに、例外を発生させてキャッチする方法を学習します。
プログラムで例外が発生すると、プログラムの実行は終了します。しかし、ほとんどの場合、プログラムの異常終了を好まないため、Python 例外処理を使用してプログラムのクラッシュを回避できます。
…を除く
次の例では、ユーザーは 10 個の整数を入力するよう求められます。ユーザーが整数ではなく他のタイプを入力すると、例外がスローされ、except
ブロックのステートメントが実行されます:
>>> n = 10
>>> i = 1
>>> sum = 0
>>> while i <= n:
try:
num = int(input('Enter a number: '))
sum = sum + num
i = i+1
except:
print('Please enter an integer!!')
break
Enter a number: 23
Enter a number: 12
Enter a number: Asd
Please enter an integer!!
>>> print('Sum of numbers =', sum)
Sum of numbers = 35
指定した例外をキャッチすることもできます。たとえば、ゼロによる除算が発生した場合、対応する例外を次のようにキャッチできます。
try:
# statements
except ZeroDivisionError:
# exception handling
except:
# all other exceptions are handled here
raise
例外
raise
キーワードを使用して、特定の例外を強制的に発生させることができます。
>>> raise ZeroDivisionError
Traceback (most recent call last):
File "<pyshell#17>", line 1, in <module>
raise ZeroDivisionError
ZeroDivisionError
>>> raise ValueError
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise ValueError
ValueError
カスタマイズされた情報を自己発生した例外に追加する必要がある場合は、例外エラータイプの後にかっこで追加できます。
>>> raise NameError("This is a customized exception")
Traceback (most recent call last):
File "<pyshell#18>", line 1, in <module>
raise NameError("This is a customized exception")
NameError: This is a customized exception
try...finally
try
ブロックでは、finally
ブロックはオプションです。最終
ブロックがある場合は、どのような場合でも実行されます。
これを以下の例で示します。
>>> try:
raise ValueError
finally:
print('You are in finally block')
You are in finally block
Traceback (most recent call last):
File "<pyshell#23>", line 2, in <module>
raise ValueError
ValueError
try ... finally
ブロックは、ファイルを操作するときなどのクリーンアップアクションに使用されます。ファイルを閉じることをお勧めします。そのため、ファイルは finally
ブロックで閉じられます。
Python 組み込み例外
このセクションでは、Python プログラミングの標準的な例外について学習します。
例外は、解釈中に発生するランタイムエラーです。たとえば、任意の数をゼロで除算すると、ZeroDivisionError
というランタイムエラーが発生します。
Python には、以下で説明する組み込みの例外がいくつかあります。
例外 | いつ育てられるの? |
---|---|
AssertionError |
assert ステートメントが失敗したとき |
AttributeError |
属性 参照が失敗したとき |
EOFError |
input() 関数によってデータが読み込まれず、ファイルの終わりに達したとき。 |
FloatingPointError |
浮動小数点計算が失敗したとき |
GeneratorExit |
コルーチンまたはジェネレーターが閉じられると、GeneratorExit 例外が発生します |
ImportError |
import がモジュールを見つけられないとき |
ModuleNotFoundError |
モジュールが import で見つからない場合。この例外は、ImportError のサブクラスです。 |
IndexError |
シーケンスのインデックスが範囲内にない場合。 |
KeyError |
辞書キーが辞書に見つからない場合 |
KeyboardInterrupt |
割り込みキーが押されたとき。 |
MemoryError |
指定された操作のメモリ量が少ない場合 |
NameError |
変数が見つからないとき |
NotImplementedError |
抽象メソッドの実装が必要であるが提供されていない場合。 |
OSError |
システムエラーが発生したとき |
OverFlowError |
値が大きすぎて表現できない場合。 |
RecursionError |
再帰制限が最大数を超えたとき |
IndentationError |
関数、クラスなどの定義にインデントが適切に指定されている場合 |
SystemError |
システム関連のエラーが見つかったとき |
SystemExit |
sys.exit() がインタープリターを終了するために使用される場合。 |
TypeError |
操作が指定されたデータ型に対して無効な場合 |
ValueError |
組み込み関数に有効な引数があるが、指定された値が無効な場合。 |
RunTimeError |
生成されたエラーが他のカテゴリに該当しない場合 |
IOError |
I/O 操作が失敗したとき。 |
UnBoundLocalError |
ローカル変数にアクセスしたが値がない場合 |
SyntaxError |
構文エラーがあるとき |
TabError |
不要なタブのインデントがある場合 |
UnicodeError |
Unicode 関連のエラーが発生したとき |
UnicodeEncodeError |
エンコードが原因で Unicode 関連のエラーが発生した場合 |
UnicodeDecodeError |
デコードのために Unicode 関連のエラーが発生した場合 |
UnicodeTranslateError |
翻訳のために Unicode 関連のエラーが発生した場合 |
ZeroDivisionError |
数値がゼロで除算されるとき |