Python Tutorial - Numbers and Conversion
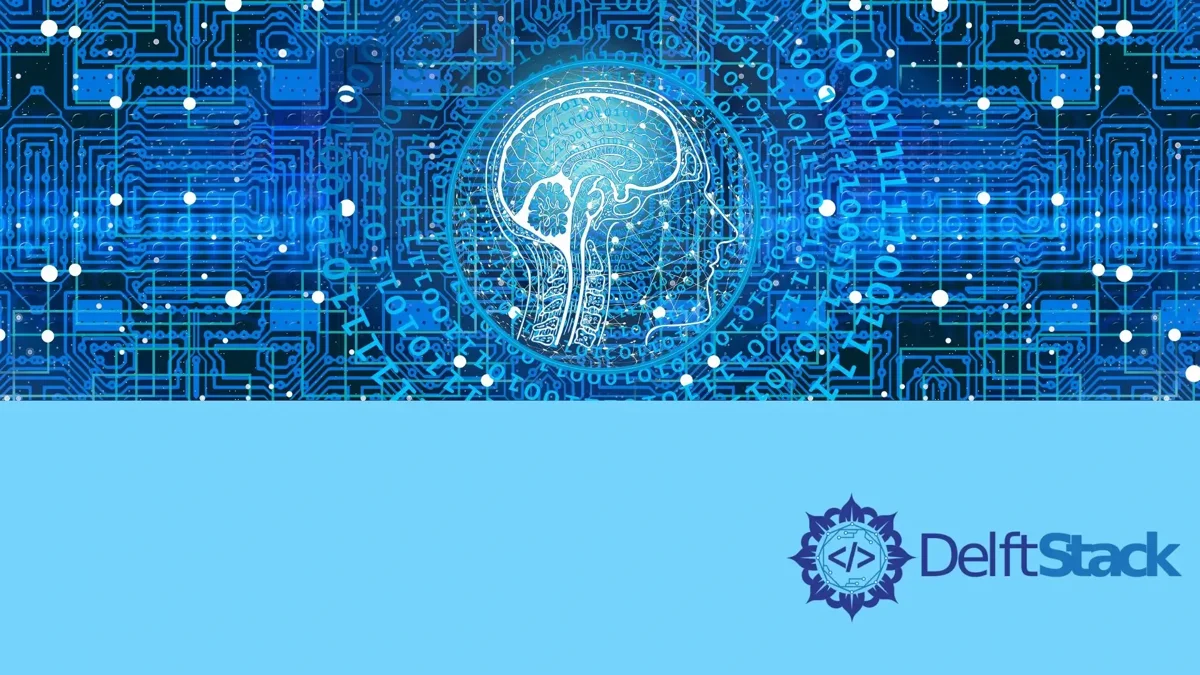
In this section, you will learn number data type in Python programming and the mathematical operations that you can perform on these numbers. Further, you will learn how to convert from one data type to another.
Python Number Data Type
The number data type in Python include:
- Integers
- Floating point numbers
- Complex numbers
Integer numbers do not have any fractional/decimal part.
Floating point numbers contain a decimal part.
Complex numbers have a real part and an imaginary part. Complex numbers are in the form x + yj
, where x
is the real part and yj
is the imaginary part.
The function type()
is used to get the data type of a variable or more generally, an object. The function isinstance()
is used to check if the variable belongs to a particular class or not.
x = 3
print("type of", x, "is", type(x))
x = 3.23
print("type of", x, "is", type(x))
x = 3 + 3j
print("is", x, "a complex number:", isinstance(x, complex))
Result:
type of 3 is <class 'int'>
type of 3.23 is <class 'float'>
is (3+3j) a complex number: True
Integer numbers can be of any length but the floating point numbers should only be up to 15 decimal places.
The integers could also be represented binary (base 2), hexadecimal (base 16) and octal (base 8) format. This can be done by using prefixes. Check the table below:
Number System | Prefixes |
---|---|
Binary | 0b or 0B |
Octal | 0o or 0O |
Hexadecimal | 0x or 0X |
Example:
>>> print(0b110)
6
>>> print(0xFA)
250
>>> print(0o12)
10
Python Number Type Conversion
Implicit Type Conversion
If you add one number of float
type and one another number of int
type, the result’s type will be float
. Here int
is converted to float
implicitly.
>>> 2 + 3.0
5.0
Here, 2 is an integer which is converted to float implicitly and you have 2.0.
This implicit type conversion is also called coercion.
Explicit Type Conversion
You could also do explicit number data type conversion using functions like int()
, float()
, str()
, etc.
>>> x = 8
>>> print("Value of x = ", int(x))
Value of x = 8
>>> print("Converted Value of x = ", float(x))
Converted Value of x = 8.0
If you want to convert float
value to int
value then the decimal part will be truncated.
Python Fractional Numbers
A module named fractions
is used to perform operations which involves fractional number. fractions
module could represent a fractional number as numerator/denominator
.
import fractions
print(fractions.Fraction(0.5))
1 / 2
The Fraction
function of the fractions
module will convert the decimal into fractions and then you can perform mathematical operations on these fractions:
print(Fraction(0.5) + Fraction(1.5))
print(Fraction(0.5) * Fraction(1.5))
print(Fraction(0.5) / Fraction(1.5))
Result:
2
3 / 4
1 / 3
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook