JavaScript Tutorial - Strings
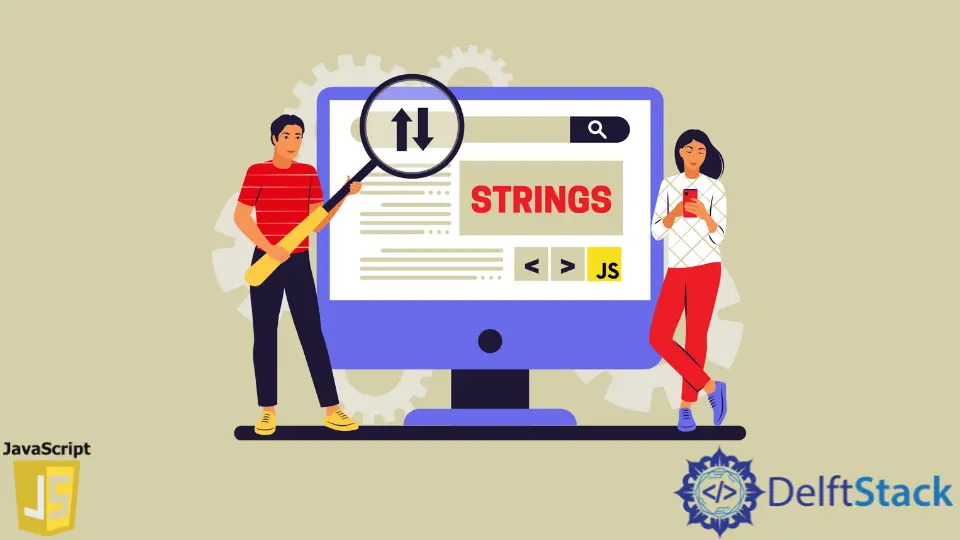
A string is a collection of text and we have to surround our string in quotation marks.
JavaScript String Concatenation
We could join two strings with the operator +
.
var exampleString = 'Hello ' +
'World'; // "Hello World"
String concatenation could be done also between string and numbers.
var x = 'My age is ' + 27; // "My age is 27".
String Method
Strings are objects like any other object in JavaScript. They have methods and properties.
JavaScript String Length Property
The length of the string is the number of characters in that string.
var stringExample = 'String Example';
console.log(stringExample.length)
// It will return 14
string.length
property returns the length of the string.
JavaScript String UpperCase/LowerCase Method
string.toUpperCase()
and string.toLowerCase()
will convert every character in the string to upper case or lower case.
> var stringExample = 'String Example';
> console.log(stringExample.toUpperCase())
STRING EXAMPLE > console.log(stringExample.toLowerCase())
string example
JavaScript indexOf
Method
indexOf
method will find the index of any particular letter or phrase within this string.
> var stringExample = 'String Example Index';
> console.log(stringExample.lastIndexOf('Example'))
7 > console.log(stringExample.lastIndexOf('example')) - 1
indexOf
method returns the first index at which it finds the given sub string in the string.
If it can’t find the phrase or the letter within the string, it will return -1
meaning that the substring doesn’t exist in this string.
JavaScript String Comparison Method
> var stringExample1 = 'ABC';
> var stringExample2 = 'abc';
> console.log(stringExample1 == stringExample2)
false > var stringExample3 = 'ABC';
> console.log(stringExample1 == stringExample3)
true
==
operator compares whether two strings are equal in the case-sensitive manner.
<
operator compares whether the first character in the first string is before the first character in the second string in the alphabet.
> var stringExample1 = 'CDE';
> var stringExample2 = 'dcd';
> console.log(stringExample1 < stringExample2)
true > var stringExample2 = 'Dcd';
> console.log(stringExample1 < stringExample2)
true > var stringExample2 = 'BCD';
> console.log(stringExample1 < stringExample2)
false
<
operator is not case sensitive, therefore, "CDE" < "DEF"
and also "CDE" < "def"
.
In contrast to <
, >
operator checks whether the first character in the first string is after the first character in the second string in the alphabet.
> var stringExample1 = 'CDE';
> var stringExample2 = 'BCD';
> console.log(stringExample1 > stringExample2)
true > var stringExample2 = 'bcd';
> console.log(stringExample1 > stringExample2)
true > var stringExample2 = 'DEF';
> console.log(stringExample1 > stringExample2)
false
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook