How to Split String in Half in Python
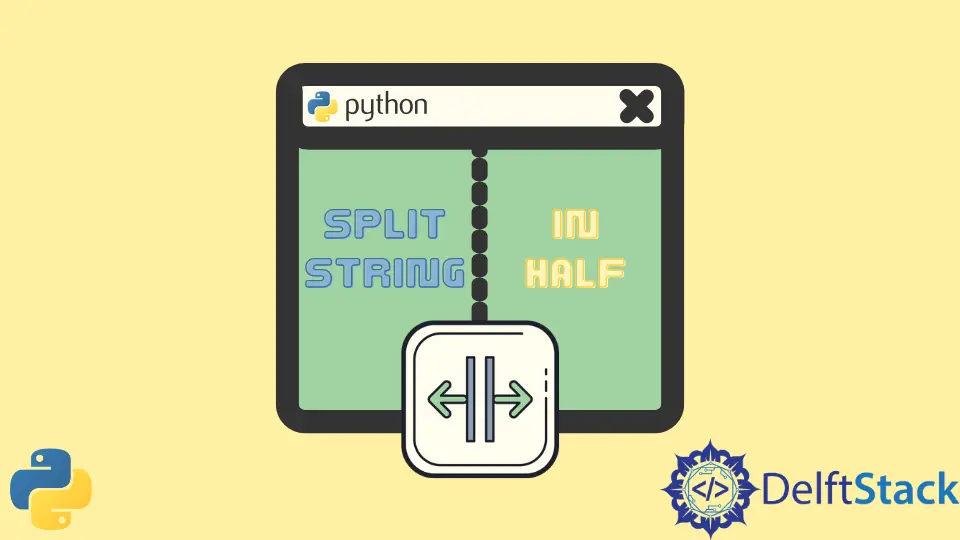
Strings can be considered as a sequence of characters. In Python, such objects are immutable and can be operated on using different functions.
In this tutorial, we will discuss how to split a string into two halves in Python.
To achieve this, we will use the string slicing method.
In strings, every character is stored at a particular position. We can use these indexes to access characters. String slicing is a method of dividing a string into substrings using the indexing method. We can use this method to split a string in half.
See the code below.
s = "string"
s1 = s[: len(s) // 2]
s2 = s[len(s) // 2 :]
print(s1, s2)
Output:
str ing
In the above code, we were dealing with a string containing an even number of characters. The len()
function here is used to return the length of the string. We split the string into one half containing the first half of the characters and the second substring containing the other half.
We use the //
operator to divide the length of the string because it performs floor division, and an integer is returned. When we work with a string containing an odd number of characters, we have to deal with the extra character resulting in two substrings of unequal length.
For example,
s = "example"
s1 = s[: len(s) // 2]
s2 = s[len(s) // 2 :]
print(s1, s2)
Output:
exa mple
We can also remove this extra character if we want. To achieve that, we just need to make some slight changes to the above code. We will use the if()
statement to check if the length of the string is even or not. If it is even, then we divide it with two and split the string; otherwise, we will increment its half with one and skip the extra character in the middle.
For example,
s = "example"
s1 = s[: len(s) // 2]
s2 = s[len(s) // 2 if len(s) % 2 == 0 else (((len(s) // 2)) + 1) :]
print(s1, s2)
Output:
exa ple
We can also use the slice()
constructor to perform string slicing. To use this method, we need to use this function and initiate an object. We use this object to perform the slicing and divide the string into two halves.
For example,
s = "string"
a = len(s)
s1 = slice(0, len(s) // 2)
s2 = slice(len(s) // 2, len(s))
print(s[s1], s[s2])
Output:
str ing
Note that this article discussed how to split a string into two equal parts. We can very easily use the above methods to split a string based on some index also.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn