How to Use Pi in Python
- Using the math Module to Access Pi
- Using NumPy for Advanced Mathematical Operations
- Defining Your Own Pi Constant
- Using SymPy for Symbolic Mathematics
- Conclusion
- FAQ
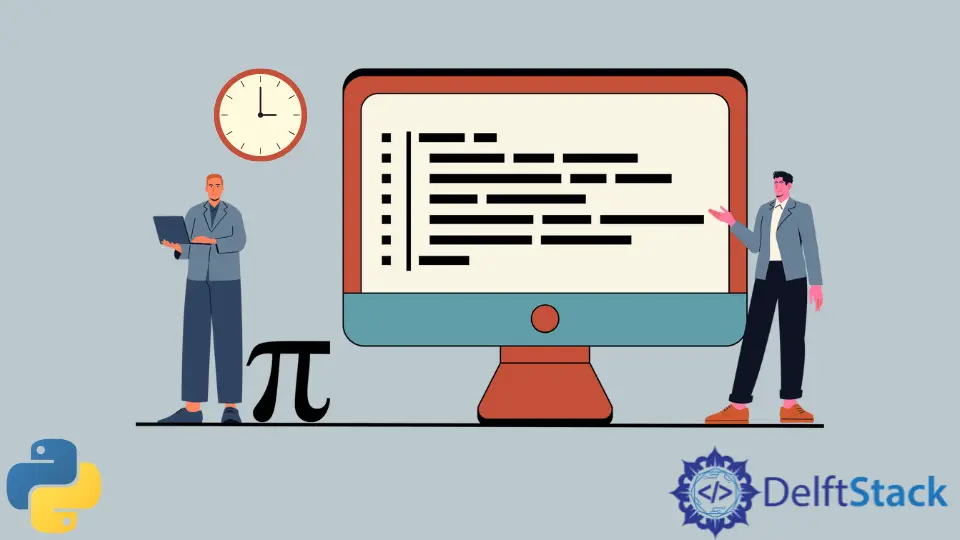
In the world of programming, mathematical constants like pi play a vital role in various applications, from scientific calculations to graphical representations. Python, known for its simplicity and versatility, provides several ways to use the value of pi in your projects. Whether you’re a beginner looking to understand the basics or an experienced developer needing a refresher, this tutorial will guide you through using pi effectively in Python. We’ll explore the different methods available, including importing from libraries and using built-in functions. By the end of this article, you’ll have a solid understanding of how to implement pi in your Python code seamlessly.
Using the math Module to Access Pi
One of the most straightforward ways to access the value of pi in Python is by using the built-in math
module. This module includes a constant math.pi
, which provides the value of pi to a high degree of accuracy. To use this method, you first need to import the math
module. Here’s how you can do it.
import math
radius = 5
area = math.pi * (radius ** 2)
print(f"The area of a circle with radius {radius} is {area}.")
Output:
The area of a circle with radius 5 is 78.53981633974483.
In this example, we first import the math
module, which gives us access to the constant math.pi
. We then define a radius for our circle and calculate the area using the formula A = πr². The result is printed out, showing the area of the circle. This method is highly efficient and widely used in various mathematical computations, making it a go-to option for many Python developers.
Using NumPy for Advanced Mathematical Operations
If you’re working on more complex mathematical tasks, the NumPy library is another excellent choice. This library not only provides the value of pi but also offers a wide range of mathematical functions for array and matrix operations. To use pi from NumPy, you need to install the library if you haven’t already. Here’s how you can utilize pi with NumPy.
import numpy as np
angle_degrees = 90
angle_radians = np.deg2rad(angle_degrees)
sine_value = np.sin(angle_radians)
print(f"The sine of {angle_degrees} degrees is {sine_value}.")
Output:
The sine of 90 degrees is 1.0.
In this example, we import the NumPy library and convert an angle from degrees to radians using np.deg2rad()
. We then calculate the sine of that angle with np.sin()
. The result indicates that the sine of 90 degrees is 1.0, which aligns with trigonometric principles. Using NumPy is particularly beneficial when dealing with arrays or performing more advanced mathematical operations, making it a powerful tool in your Python arsenal.
Defining Your Own Pi Constant
For those who prefer a more hands-on approach, you can also define your own constant for pi. While this is not necessary for most applications, it can be useful for educational purposes or when you want to avoid importing external libraries. Here’s how you can define and use your own pi constant.
PI = 3.141592653589793
diameter = 10
circumference = PI * diameter
print(f"The circumference of a circle with diameter {diameter} is {circumference}.")
Output:
The circumference of a circle with diameter 10 is 31.41592653589793.
In this code snippet, we define our own constant PI
with a value of 3.141592653589793. We then calculate the circumference of a circle using the formula C = πd, where d is the diameter. The output shows the circumference of the circle based on our defined constant. While this method works well, it’s important to note that using the math
module or NumPy is generally more reliable for precision in mathematical calculations.
Using SymPy for Symbolic Mathematics
If you’re delving into symbolic mathematics, the SymPy library is an excellent option. It allows for symbolic computation, which means you can manipulate mathematical expressions symbolically rather than numerically. Here’s how to use pi with SymPy.
from sympy import pi, symbols
x = symbols('x')
expression = pi * x**2
print(f"The symbolic expression for the area of a circle is: {expression}.")
Output:
The symbolic expression for the area of a circle is: pi*x**2.
In this example, we import pi
from SymPy and create a symbolic variable x
. We then define an expression for the area of a circle symbolically as πx². The output displays the symbolic expression rather than a numerical value. This method is particularly useful in fields such as algebra and calculus, where you may need to manipulate formulas or solve equations symbolically.
Conclusion
Using pi in Python is straightforward and versatile, thanks to the various libraries and methods available. Whether you choose to use the built-in math
module, leverage the power of NumPy, define your own constant, or explore symbolic mathematics with SymPy, understanding how to work with pi can enhance your programming skills and mathematical computations. By incorporating these methods into your projects, you can ensure accurate and efficient calculations, making your Python applications more robust and effective.
FAQ
-
What is the value of pi in Python?
The value of pi in Python can be accessed using themath
module asmath.pi
, which is approximately 3.14159. -
Can I use pi in NumPy?
Yes, you can use pi in NumPy by accessing it vianp.pi
, which provides the same value as themath
module. -
Is it necessary to import a library to use pi in Python?
While it is common to import libraries likemath
orNumPy
to access pi, you can also define your own constant if needed. -
What is the difference between
math.pi
andnumpy.pi
?
Both provide the same value of pi, butnumpy.pi
is often used in the context of array operations and mathematical functions provided by NumPy. -
Can I perform symbolic mathematics with pi in Python?
Yes, you can use the SymPy library to perform symbolic mathematics with pi, allowing for manipulation of mathematical expressions.