How to Get Permutations of a String in Python
-
Use the
itertools.permutations()
Function to Return All the Permutations of a String in Python - Create a User-Defined Function to Return All the Permutations for a String in Python
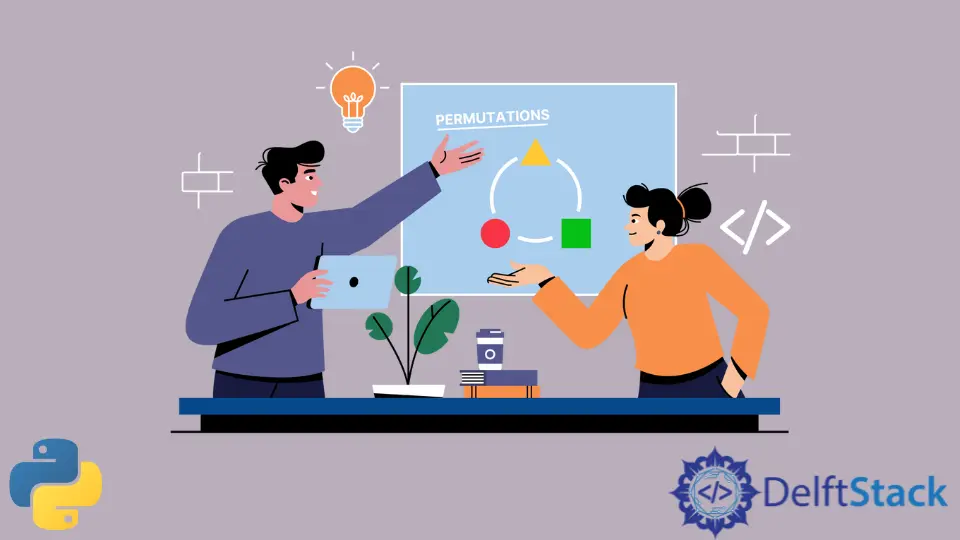
By permutation, we mean the total number of rearrangements possible for a given number of elements in a unique way without considering the rearrangement order.
A string, as we know, can be thought of as a collection of individual characters.
In this article, we will try to find all the possible permutations for a given string.
Use the itertools.permutations()
Function to Return All the Permutations of a String in Python
The itertools
module is used to create and work with different iterable objects. The permutations()
function from this module can return all the possible arrangements for a given set of values.
It returns an itertools
type object which contains a tuple containing the possible arrangement of the elements. We can use a list to view the elements of this object. We can use this function with a string also.
For example,
from itertools import permutations
lst = list(permutations("day"))
print(lst)
Output:
[('d', 'a', 'y'), ('d', 'y', 'a'), ('a', 'd', 'y'), ('a', 'y', 'd'), ('y', 'd', 'a'), ('y', 'a', 'd')]
Notice the tuples created in the output containing the arrangement of characters. We can change this to a list of strings using the join() function and list comprehension method.
See the following code.
from itertools import permutations
lst = ["".join(p) for p in permutations("day")]
print(lst)
Output:
['day', 'dya', 'ady', 'ayd', 'yda', 'yad']
We combine the tuple elements using the join()
function and use this for each tuple by iterating through the list.
Create a User-Defined Function to Return All the Permutations for a String in Python
We can create a straightforward function to find all the permutations of a string. We will create a recursive function. In this method, we will be just swapping the string elements once and calling the function again with the new arrangement. We display the final arrangements.
We implement the above logic in the following code.
def string_permutations(s, i, n):
if i == n:
print("".join(s))
else:
for j in range(i, n):
s[i], s[j] = s[j], s[i]
string_permutations(s, i + 1, n)
s[i], s[j] = s[j], s[i]
a = "day"
x = len(a)
s = list(a)
print(permute(s, 0, x))
Output:
day
dya
ady
ayd
yad
yda
None
As you can see, the start and end positions are specified where we wish to get the rearrangements done. The string is also passed as a list of characters. To find all possible permutations, we set the start to 0 and the end as the length of the string.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn