How to Initialize a Dictionary in Python
- Use the Literal Syntax to Initialize a Dictionary in Python
-
Use the
dict()
Constructor to Initialize a Dictionary in Python -
Use the
fromkeys()
Method to Initialize a Dictionary in Python - Use a List of Tuples to Initialize a Dictionary in Python
- Use Two Lists to Initialize a Dictionary in Python
- Use Dictionary Comprehension to Initialize a Dictionary in Python
-
Use the
setdefault()
Method to Initialize a Dictionary in Python -
Use the
__setitem__()
Method to Initialize a Dictionary in Python - Conclusion
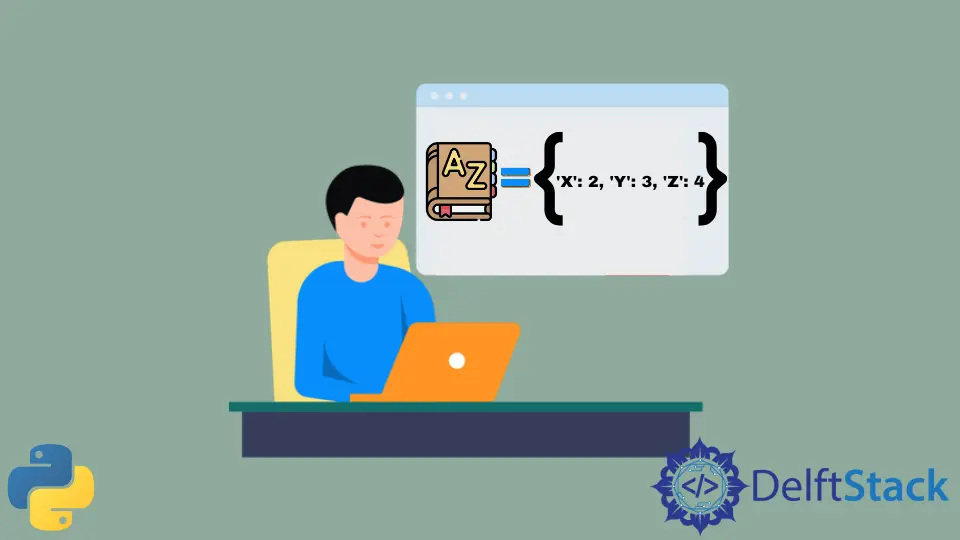
In Python, dictionaries are powerful data structures that allow us to store and retrieve data using key-value pairs. They play a crucial role in organizing and manipulating data efficiently.
Unlike lists or tuples, which use numerical indices, dictionaries use keys as unique identifiers. This key-value relationship enables us to rapidly retrieve and modify data, making dictionaries invaluable for various programming tasks.
Imagine we are building an address book application to store contact information for our friends. Each contact can have a unique name associated with a phone number.
By using a dictionary, we can initialize an address book with keys representing names and values representing corresponding phone numbers. This allows for efficient retrieval of phone numbers by simply providing the name as a key.
Understanding how to initialize a dictionary is fundamental for any Python programmer. In Python, dictionaries are ordered and changeable. Dictionaries do not allow the storage of duplicate keys.
In Python versions 3.6 and below, dictionaries used to be unordered. After the introduction of Python 3.7+, dictionaries are ordered.
This tutorial will discuss different methods to initialize a dictionary in Python.
Use the Literal Syntax to Initialize a Dictionary in Python
When initializing a dictionary in Python, we have several methods. One straightforward approach uses the literal syntax, which specifies the key-value pairs within curly braces {}
.
This method allows us to define the dictionary’s content explicitly, concisely, and readably. By leveraging the literal syntax, we can quickly create dictionaries containing any combination of data types as keys or values.
The following code uses the literal syntax to initialize a dictionary in Python.
dict1 = {"X": 2, "Y": 3, "Z": 4}
print(dict1)
Output:
The above code snippet initializes a dictionary named dict1
with three key-value pairs. The keys are strings X
, Y
, and Z
, and the corresponding values are integers 2
, 3
, and 4
.
Each key-value pair is separated by a colon and enclosed within curly braces {}
.
Use the dict()
Constructor to Initialize a Dictionary in Python
Another method to initialize a dictionary in Python is by using the dict()
constructor. The dict()
constructor allows us to create dictionaries by passing key-value pairs as arguments or providing an iterable object containing key-value pairs.
This method offers flexibility when initializing dictionaries, allowing us to dynamically generate the dictionary’s content based on varying conditions or data sources. Compared to the previously discussed literal syntax method, the dict()
constructor provides a more programmatic approach to dictionary initialization.
It enables us to construct dictionaries dynamically at runtime, making it suitable for scenarios where the dictionary’s content may change or need to be derived from other data structures or computations.
The following code uses the dict()
constructor to initialize a dictionary in Python.
dict1 = dict(X=1, Y=2, Z=3)
print(dict1)
Output:
The above code snippet initializes a dictionary named dict1
using the dict()
constructor. The constructor takes keyword arguments in the form of key-value pairs.
In this case, the keys are X
, Y
, and Z
, and the corresponding values are integers 1
, 2
, and 3
, respectively.
Use the fromkeys()
Method to Initialize a Dictionary in Python
The fromkeys()
method in Python allows us to initialize a dictionary by providing a sequence of keys and an optional default value. It creates a new dictionary where each key from the sequence is mapped to the same default value.
Syntax:
dict.fromkeys(keys, value)
Parameters:
keys
: A sequence or iterable containing the keys to be used in the dictionary.value
(optional): The value to be assigned to each key in the dictionary. If not provided, the default value isNone
.
This method is particularly useful when we want to set default values for multiple keys in a dictionary. Compared to the previously discussed methods, the fromkeys()
method provides a convenient way to initialize dictionaries with default values without explicitly specifying each key-value pair.
It offers a compact and efficient approach, especially when dealing with large dictionaries or when all keys require the same initial value. The following code uses the fromkeys()
method to initialize a dictionary in Python.
dict1 = dict.fromkeys(["X", "Y", "Z"], 0)
print(dict1)
Output:
The above code snippet initializes a dictionary named dict1
using the fromkeys()
method. The fromkeys()
method takes two arguments: the first is a sequence or iterable containing the keys to be used in the dictionary, and the second is the default value assigned to each key.
In this example, ['X', 'Y', 'Z']
is the sequence of keys, and 0
is the default value. The fromkeys()
method creates a new dictionary with keys X
, Y
, and Z
and assigns the default value of 0
to each key.
Use a List of Tuples to Initialize a Dictionary in Python
Another approach to initialize a dictionary in Python is using a list of tuples. This method allows us to provide a collection of key-value pairs as input to create the dictionary. Each tuple within the list represents a key-value pair.
Syntax:
dict1 = dict([(key1, value1), (key2, value2), ...])
Parameters:
key1
,key2
, …: Keys to be used in the dictionary.value1
,value2
, …: Corresponding values to be assigned to the respective keys.
A tuple is an ordered and immutable collection of objects. It can be utilized to stock multiple items in a single variable.
Lists are similar to tuples; the only difference is that lists can be changed, and tuples do not allow that.
Compared to the previously discussed methods, using a list of tuples provides a more explicit way of defining key-value pairs. It allows us to initialize dictionaries using existing data structures or computations, offering greater flexibility in populating the dictionary.
The following code uses a list of tuples to initialize a dictionary in Python.
LOT = [("X", 5), ("Y", 6), ("Z", 8)]
dict1 = dict(LOT)
print(dict1)
Output:
The above code snippet initializes a list of tuples named LOT
. Each tuple within the list represents a key-value pair, where the first element is the key and the second element is the corresponding value.
The line dict1 = dict(LOT)
creates a dictionary named dict1
by passing the LOT
list of tuples as an argument to the dict()
constructor. This allows us to convert the list of tuples into a dictionary where each key-value pair is preserved.
Use Two Lists to Initialize a Dictionary in Python
Another method to initialize a dictionary in Python is using two lists, one for keys and another for values. This approach allows us to create a dictionary by pairing corresponding elements from both lists.
To implement the above statements, we can use the zip()
function, which iterates over both the given lists in parallel. The zip()
function will create a parallel key-value pair for all the entries and successfully create a zipped object, which can then be passed to the dict()
constructor to create a dictionary.
Syntax:
keys = ["key1", "key2", ...]
values = ["value1", "value2", ...]
dict1 = dict(zip(keys, values))
Parameters:
keys
: A list containing the keys used in the dictionary.values
: A list containing the corresponding values to be assigned to the respective keys.
Compared to the previously discussed methods, using two lists provides a straightforward way to create a dictionary by explicitly mapping keys to their corresponding values. It allows for flexibility in constructing dictionaries from pre-existing lists, enabling efficient data organization and retrieval.
The following code uses two lists to initialize a dictionary in Python.
L1 = ["X", "Y", "Z"]
L2 = [5, 6, 8]
dict1 = dict(zip(L1, L2))
print(dict1)
Output:
The above code snippet L1 = ["X", "Y", "Z"]
initializes a list L1
containing the keys for the dictionary, while L2 = [5, 6, 8]
initializes a list L2
containing the corresponding values. The line dict1 = dict(zip(L1, L2))
creates a dictionary named dict1
by using the zip()
function to pair the elements of L1
and L2
together.
The zip()
function combines each key from L1
with its corresponding value from L2
, resulting in a sequence of tuples. The dict()
constructor converts this sequence of tuples into a dictionary.
Use Dictionary Comprehension to Initialize a Dictionary in Python
Python provides a concise and powerful method to initialize a dictionary called dictionary comprehension. Dictionary comprehension allows us to create dictionaries dynamically by iterating over iterable objects and defining key-value pairs in a single line of code.
Syntax:
dict1 = {key_expression: value_expression for item in iterable}
Parameters:
key_expression
: The expression that defines the key for each key-value pair.value_expression
: The expression defining each key-value pair’s value.item
: The variable representing each item in the iterable object.
The following code uses dictionary comprehension to initialize a dictionary in Python.
keys = ["X", "Y", "Z"]
dict1 = {x: 0 for x in keys}
print(dict1)
Output:
The above code snippet initializes a list, keys
, containing the keys for the dictionary. The line dict1 = {x: 0 for x in keys}
uses dictionary comprehension to create a dictionary named dict1
.
It iterates over each element x
in the keys
list and assigns a value of 0
to each key.
Use the setdefault()
Method to Initialize a Dictionary in Python
The setdefault()
method in Python provides a way to initialize a dictionary while also checking and setting a default value for a given key. It allows us to specify a default value for a key if it doesn’t already exist in the dictionary.
Syntax:
dict1.setdefault(key, default_value)
Parameters:
key
: The key to be checked and set a default value.default_value
: The value to be assigned to the key if it doesn’t exist in the dictionary.
Compared to the previously discussed methods, setdefault()
provides a way to initialize a dictionary while simultaneously handling potential key existence scenarios. It allows us to set default values for keys not present in the dictionary. It makes it useful for scenarios where we need to initialize values for new keys or update existing ones.
The following code uses the setdefault()
method to initialize a dictionary in Python.
dict1 = {}
dict1.setdefault("X", 0)
dict1.setdefault("Y", 1)
dict1.setdefault("Z", 2)
print(dict1)
Output:
An empty dictionary dict1
is created in the above code snippet. The setdefault()
method is then used to initialize keys 'X'
, 'Y'
, and 'Z'
with default values of 0
, 1
, and 2
, respectively.
If a key already exists in the dictionary, setdefault()
doesn’t modify its value.
Use the __setitem__()
Method to Initialize a Dictionary in Python
In Python, the __setitem__()
method provides another way to initialize a dictionary by directly setting key-value pairs. It is an internal method that is automatically called when assigning a value to a key in a dictionary.
Syntax:
dict1 = {}
dict1.__setitem__(key, value)
Parameters:
key
: The key to be set in the dictionary.value
: The value to be assigned to the key.
Compared to the previously discussed methods, the __setitem__()
method provides a low-level way to initialize dictionaries by directly modifying individual keys and their corresponding values. It offers fine-grained control over the initialization process and is useful in specific scenarios where custom logic is required to set key values.
The following code uses the __setitem__()
method to initialize a dictionary in Python.
dict1 = {}
dict1.__setitem__("X", 0)
dict1.__setitem__("Y", 1)
dict1.__setitem__("Z", 2)
print(dict1)
Output:
The lines dict1.__setitem__('X', 0)
, dict1.__setitem__('Y', 1)
, and dict1.__setitem__('Z', 2)
use the __setitem__()
method to set key-value pairs in the dict1
dictionary. The method explicitly assigns the values 0
, 1
, and 2
to the keys X
, Y
, and Z
.
Conclusion
This tutorial explored several methods to initialize dictionaries in Python, each offering advantages and use cases.
When choosing the appropriate method, we should consider the specific requirements of our program. The literal syntax and dict()
constructor are suitable for straightforward initialization, while the list of tuples, setdefault()
, and __setitem__()
allow for more customization.
By mastering these techniques, we gain the knowledge and tools to efficiently initialize dictionaries in Python, allowing us to organize and manipulate data in our programs effectively.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn