How to Initiate 2-D Array in Python
- List Comprehension Method to Initiate a 2D Array
-
Nested
range
Method to Initiate a 2D Array -
NumPy
Method to Initiate a 2D Array
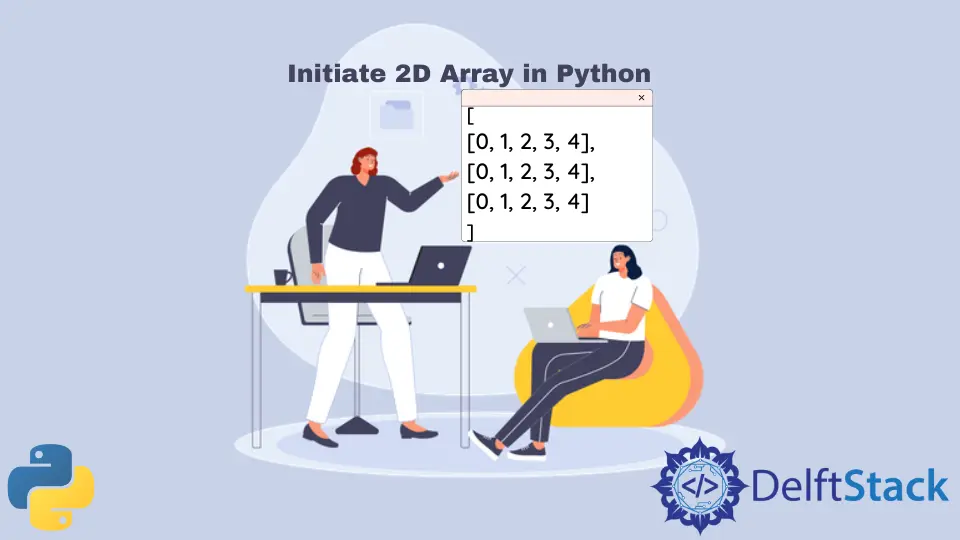
This tutorial guide will introduce different methods to initiate a 2-D array in Python. We will make a 3x5
2-D array in the following examples.
List Comprehension Method to Initiate a 2D Array
>>> column, row = 3, 5
>>> array2D = [[0 for _ in range(row)] for _ in range(column)]
>>> array2D
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]
This nested list comprehension method creates 2-D array with the initial value as 0
. Of course, you could change the initial value to any value you need to assign in your application.
Nested range
Method to Initiate a 2D Array
If you don’t care about the initial value in the 2-D array, the value 0
could be even eliminated.
In Python 2.x
>>> column, row = 3, 5
>>> A = [range(row) for _ in range(column)]
>>> A
[[0, 1, 2, 3, 4], [0, 1, 2, 3, 4], [0, 1, 2, 3, 4]]
In Python 3.x
>>> column, row = 3, 5
>>> A = [range(row) for _ in range(column)]
>>> A
[range(0, 5), range(0, 5), range(0, 5)]
We couldn’t simply use range(x)
to initiate 2-D array in Python 3.x because range
returns an object containing a sequence of integers in Python 3.x, but not a list of integers as in Python 2.x.
range
in Python 3.x is more similar to xrange
in Python 2.x. range
object in Python 3.x is immutable, therefore, you don’t assign items to its elements.
If you need item assignment, you need to convert the range
to list
object.
>>> A = [list(range(row)) for _ in range(column)]
>>> A
[[0, 1, 2, 3, 4], [0, 1, 2, 3, 4], [0, 1, 2, 3, 4]]
[0] * N
Method to Initiate a 2D Array
One Pythonic way to initiate a 2-D array could be
>>> column, row = 3, 5
>>> A = [[0]*row for _ in range(column)]
>>> A
[[0, 0, 0, 0, 0], [0, 0, 0, 0, 0], [0, 0, 0, 0, 0]]
Although we should be cautious when we use list multiplication because it simply creates a sequence with multiple times referred to one same object, we are relieved to use [0]*n
here because data object 0
is immutable so that we will never encounter problems even with references to the same immutable object.
NumPy
Method to Initiate a 2D Array
Besides the native Python array, NumPy
should be the best option to create a 2-D array, or to be more precise, a matrix.
You could create a matrix filled with zeros with numpy.zeros
.
>>> import numpy as np
>>> column, row = 3, 5
>>> np.zeros(column, row)
array([[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.],
[0., 0., 0., 0., 0.]])
Or initiate a matrix filled with ones
with numpy.ones
>>> import numpy as np
>>> column, row = 3, 5
>>> np.ones((column, row))
array([[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.],
[1., 1., 1., 1., 1.]])
You could even create a new array without initializing entries with numpy.empty
>>> import numpy as np
>>> column, row = 3, 5
>>> np.empty((5,5))
array([[6.23042070e-307, 4.67296746e-307, 1.69121096e-306,
1.33511562e-306, 1.89146896e-307],
[7.56571288e-307, 3.11525958e-307, 1.24610723e-306,
1.37962320e-306, 1.29060871e-306],
[2.22518251e-306, 1.33511969e-306, 1.78022342e-306,
1.05700345e-307, 1.11261027e-306],
[1.11261502e-306, 1.42410839e-306, 7.56597770e-307,
6.23059726e-307, 1.42419530e-306],
[7.56599128e-307, 1.78022206e-306, 8.34451503e-308,
2.22507386e-306, 7.20705877e+159]])
Initialize 2D Array in Python Using the numpy.full()
Method
This method will also initialize the list elements, but it is slower than the list Comprehension method.
The complete example code is as follows:
import numpy
dim_columns = 2
dim_rows = 2
output = numpy.full((dim_columns, dim_rows), 0).tolist()
print(output)
The numpy.full()
function of the NumPy will create an array and the tolist()
function of NumPy will convert that array to a Python List.
Output:
[[0, 0], [0, 0]]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook