How to Count the Occurrences of an Item in a One-Dimensional Array in Python
-
Use
collections
to Find the Number of Occurrence in an Array in Python - Use NumPy Library to Find the Number of Occurrence in an Array in Python
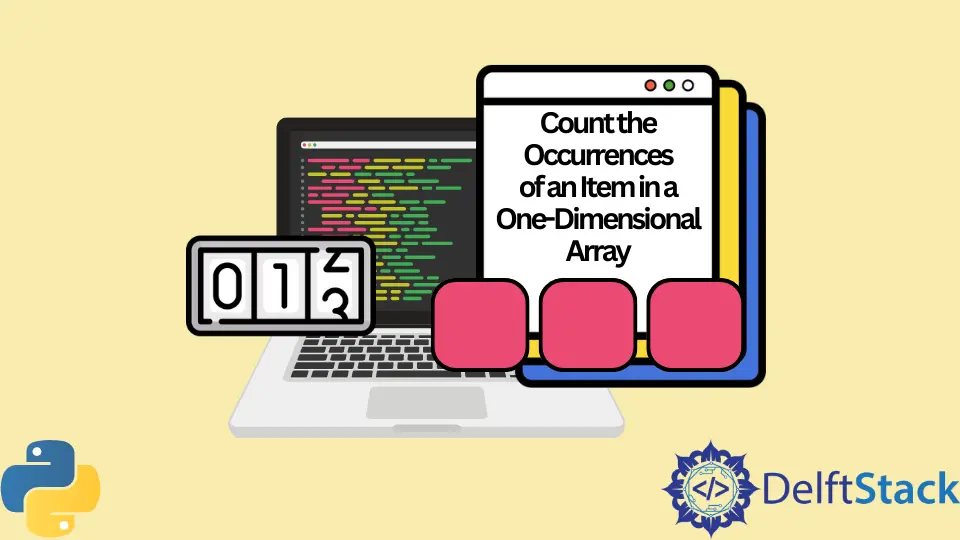
While working with an array one of the major issues a developer can face, is counting the number of occurrences of an item. Imagine if you have an array of the number of items sold in an eCommerce site over 10 days, you would like to know the number of days more than 100 items are sold.
sales = [0, 100, 100, 80, 70, 80, 20, 10, 100, 100, 80, 70, 10, 30, 40]
The easiest way to solve is to get a count of the number of times 100 occurs in the array.
Use collections
to Find the Number of Occurrence in an Array in Python
collections
act like containers to store collections of data. We can easily import the collections
module and use the Counter
function.
Check the code below:
import collections
sales = [0, 100, 100, 80, 70, 80, 20, 10, 100, 100, 80, 70, 10, 30, 40]
print(collections.Counter(sales))
Output:
Counter({100: 4, 80: 3, 70: 2, 10: 2, 0: 1, 20: 1, 30: 1, 40: 1})
The resultant output is a Python dictionary. It lists how many times each item in the array has occurred.
However, if we want to print the number of times 100 occurs in the sales
array, we can fetch it from the dictionary.
>>>print(collections.Counter(sales)[100])
4
The collections
module also works with decimal numbers and strings.
floatarr = [
0.7,
10.0,
10.1,
0.8,
0.7,
0.8,
0.2,
0.1,
10.0,
10.0,
0.8,
0.8,
0.7,
0.7,
0.8,
]
print(collections.Counter(floatarr))
stringarr = ["george", "mark", "george", "steve", "george"]
print(collections.Counter(stringarr))
Output:
Counter({0.8: 5, 0.7: 4, 10.0: 3, 10.1: 1, 0.2: 1, 0.1: 1})
Counter({'george': 3, 'mark': 1, 'steve': 1})
Use NumPy Library to Find the Number of Occurrence in an Array in Python
However, we can also use NumPy, which is a library defined in Python to handle large arrays and also contains a large number of mathematical functions.
There are several ways you can use the functions defined in NumPy to return the item counts in an array.
Use unique
Function in NumPy
The unique
function along with Count, returns a dictionary of the count of each item. It also works with decimal numbers and strings.
import collections
import numpy
aUnique = numpy.array([0, 100, 100, 80, 70, 80, 20, 10, 100, 100, 80, 70, 10, 30, 40])
unique, counts = numpy.unique(aUnique, return_counts=True)
print(dict(zip(unique, counts)))
Output:
{0: 1, 10: 2, 20: 1, 30: 1, 40: 1, 70: 2, 80: 3, 100: 4}
Use count_nonzero
Function in NumPy
Using the count_nonzero
returns the count of the item we are searching for. It provides an easy to read interface and fewer lines of code.
>>>aCountZero = numpy.array([0, 100.1, 100.1, 80, 70, 80, 20, 10,
100, 100, 80, 70, 10, 30, 40,"abc"])
>>>print(numpy.count_nonzero(aCountZero == "abc"))
1
count_nonzero
also works with decimal numbers and strings.
>>>aCountZero = numpy.array([0, 100.1, 100.1, 80, 70, 80, 20, 10,
100, 100, 80, 70, 10, 30, 40])
>>>print(numpy.count_nonzero(aCountZero == 100.1))
1
Use bincount
Function in NumPy - Only for Array With Integers
However, if you have an array that has only integers, you can use bincount
function of NumPy. The best part is, it returns the results as an array.
>>>abit = numpy.array([0, 6, 0, 10, 0, 1, 1, 0, 10, 9, 0, 1])
>>>print(numpy.bincount(abit))
[5 3 0 0 0 0 1 0 0 1 2]
For the numbers in the array, the result displays the count of items in ascending order. For example, 0 in array abit
occurs 5 times and 10 occurs 2 times as denoted by the first and last item of the array.